uniapp 小程序蓝牙控制车位锁代码
时间: 2023-08-07 07:02:51 浏览: 54
以下是一个基于uniapp框架的小程序蓝牙控制车位锁的代码示例:
1. 在uniapp项目中创建一个新的页面,用于蓝牙控制
2. 在该页面中引入uni的蓝牙插件uni-ble,并初始化蓝牙设备
```javascript
import ble from '@/uni_modules/uni-ble/js_sdk/uni-ble.js'
export default {
data() {
return {
deviceId: '', // 蓝牙设备id
serviceId: '', // 蓝牙服务id
writeId: '', // 写入特征值id
readId: '', // 读取特征值id
notifyId: '', // 通知特征值id
connected: false // 是否连接成功
}
},
onLoad() {
this.initBluetooth()
},
methods: {
initBluetooth() {
// 初始化蓝牙
ble.openAdapter()
// 监听蓝牙状态变化
ble.onBluetoothAdapterStateChange({
success: res => {
if (res.available) {
console.log('蓝牙可用')
} else {
console.log('蓝牙不可用')
}
}
})
}
}
}
```
3. 扫描蓝牙设备,连接车位锁
```javascript
methods: {
// 开始扫描蓝牙设备
startScan() {
ble.startBluetoothDevicesDiscovery({
success: res => {
console.log('扫描蓝牙成功')
this.devices = res.devices
}
})
},
// 停止扫描蓝牙设备
stopScan() {
ble.stopBluetoothDevicesDiscovery()
},
// 连接蓝牙设备
connectDevice(deviceId) {
ble.createBLEConnection({
deviceId: deviceId,
success: res => {
console.log('连接蓝牙成功')
this.deviceId = deviceId
this.getServices()
}
})
},
// 获取蓝牙服务
getServices() {
ble.getBLEDeviceServices({
deviceId: this.deviceId,
success: res => {
console.log('获取蓝牙服务成功')
for (let i = 0; i < res.services.length; i++) {
if (res.services[i].isPrimary) {
this.serviceId = res.services[i].uuid
this.getCharacteristics()
break
}
}
}
})
},
// 获取特征值
getCharacteristics() {
ble.getBLEDeviceCharacteristics({
deviceId: this.deviceId,
serviceId: this.serviceId,
success: res => {
console.log('获取特征值成功')
for (let i = 0; i < res.characteristics.length; i++) {
let item = res.characteristics[i]
if (item.properties.write) {
this.writeId = item.uuid
} else if (item.properties.read) {
this.readId = item.uuid
ble.readBLECharacteristicValue({
deviceId: this.deviceId,
serviceId: this.serviceId,
characteristicId: this.readId,
success: res => {
console.log('读取特征值成功')
}
})
} else if (item.properties.notify) {
this.notifyId = item.uuid
ble.notifyBLECharacteristicValueChange({
deviceId: this.deviceId,
serviceId: this.serviceId,
characteristicId: this.notifyId,
state: true,
success: res => {
console.log('监听特征值变化成功')
// 监听特征值变化
ble.onBLECharacteristicValueChange({
characteristicId: this.notifyId,
success: res => {
console.log('特征值变化:', res)
// 根据特征值的变化进行车位锁的控制
}
})
}
})
}
}
this.connected = true
}
})
}
}
```
4. 根据特征值变化进行车位锁的控制
```javascript
methods: {
// ...
// 监听特征值变化
ble.notifyBLECharacteristicValueChange({
deviceId: this.deviceId,
serviceId: this.serviceId,
characteristicId: this.notifyId,
state: true,
success: res => {
console.log('监听特征值变化成功')
// 监听特征值变化
ble.onBLECharacteristicValueChange({
characteristicId: this.notifyId,
success: res => {
console.log('特征值变化:', res)
// 根据特征值的变化进行车位锁的控制
let value = new Uint8Array(res.value)
if (value[0] === 1) {
console.log('车位锁已经打开')
} else if (value[0] === 0) {
console.log('车位锁已经关闭')
}
}
})
}
})
}
```
以上是一个简单的小程序蓝牙控制车位锁的代码示例,你可以根据自己的需求进行修改和完善。
相关推荐
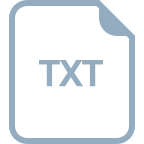














