Java EE高级编程,创建职工表和部门信息表(字段自定义,数据自定义)。
时间: 2024-02-24 22:55:34 浏览: 68
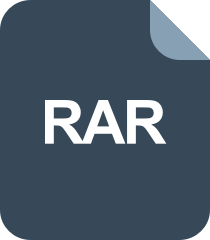
自定义报表开发,自定义报表工具

以下是一个简单的Java EE高级编程示例,使用MySQL数据库,创建职工表和部门信息表,同时添加数据:
首先,我们需要创建一个 `Department` 类和一个 `Employee` 类,分别用于表示部门和职工信息:
```java
// Department.java
public class Department {
private int id;
private String name;
public Department() {}
public Department(int id, String name) {
this.id = id;
this.name = name;
}
// getters and setters
// ...
}
// Employee.java
public class Employee {
private int id;
private String name;
private double salary;
private int departmentId;
public Employee() {}
public Employee(int id, String name, double salary, int departmentId) {
this.id = id;
this.name = name;
this.salary = salary;
this.departmentId = departmentId;
}
// getters and setters
// ...
}
```
接下来,我们需要编写一个数据库访问类 `DBUtil`,用于连接数据库并执行 SQL 语句:
```java
// DBUtil.java
public class DBUtil {
private static final String URL = "jdbc:mysql://localhost:3306/test?useSSL=false&allowPublicKeyRetrieval=true&serverTimezone=UTC";
private static final String USERNAME = "root";
private static final String PASSWORD = "password";
public static Connection getConnection() throws SQLException {
return DriverManager.getConnection(URL, USERNAME, PASSWORD);
}
public static void close(Connection conn, Statement stmt, ResultSet rs) {
try {
if (rs != null) rs.close();
if (stmt != null) stmt.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
// 执行 SQL 语句
public static void execute(String sql) throws SQLException {
Connection conn = null;
Statement stmt = null;
try {
conn = getConnection();
stmt = conn.createStatement();
stmt.execute(sql);
} finally {
close(conn, stmt, null);
}
}
}
```
然后,我们可以在 `main` 方法中调用 `DBUtil.execute` 方法,创建表并插入数据:
```java
public static void main(String[] args) {
try {
// 创建部门信息表
String createDeptTableSql = "CREATE TABLE department ("
+ "id INT PRIMARY KEY,"
+ "name VARCHAR(50) NOT NULL"
+ ")";
DBUtil.execute(createDeptTableSql);
// 插入部门信息
Department[] departments = {
new Department(1, "人事部"),
new Department(2, "财务部"),
new Department(3, "市场部")
};
for (Department dept : departments) {
String insertDeptSql = "INSERT INTO department (id, name) VALUES ("
+ dept.getId() + ", '"
+ dept.getName() + "')";
DBUtil.execute(insertDeptSql);
}
// 创建职工表
String createEmpTableSql = "CREATE TABLE employee ("
+ "id INT PRIMARY KEY,"
+ "name VARCHAR(50) NOT NULL,"
+ "salary DOUBLE NOT NULL,"
+ "department_id INT NOT NULL,"
+ "FOREIGN KEY (department_id) REFERENCES department (id)"
+ ")";
DBUtil.execute(createEmpTableSql);
// 插入职工信息
Employee[] employees = {
new Employee(1, "张三", 5000, 1),
new Employee(2, "李四", 6000, 1),
new Employee(3, "王五", 7000, 2),
new Employee(4, "赵六", 8000, 3)
};
for (Employee emp : employees) {
String insertEmpSql = "INSERT INTO employee (id, name, salary, department_id) VALUES ("
+ emp.getId() + ", '"
+ emp.getName() + "', "
+ emp.getSalary() + ", "
+ emp.getDepartmentId() + ")";
DBUtil.execute(insertEmpSql);
}
} catch (SQLException e) {
e.printStackTrace();
}
}
```
注意,在实际项目中,我们应该将数据库访问和业务逻辑分离,使用 DAO 层或者 ORM 框架来管理数据库访问。
阅读全文
相关推荐
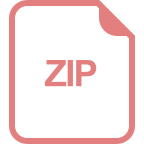
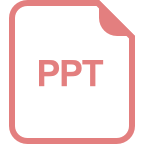
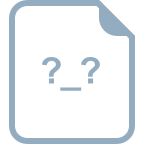
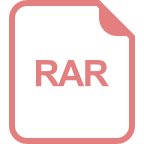
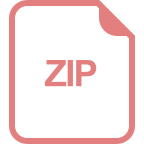
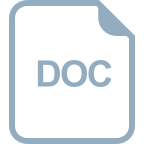
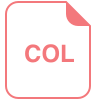
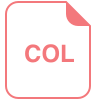
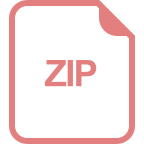
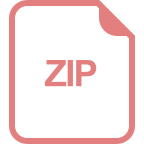
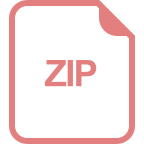
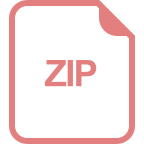
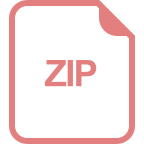