C语言模拟实现银行家算法,四中资源,五个进程
时间: 2024-04-09 21:06:52 浏览: 141
以下是C语言模拟实现银行家算法的示例代码,假设有四种资源(A、B、C、D),五个进程(P1、P2、P3、P4、P5):
```c
#include <stdio.h>
#include <stdbool.h>
// 定义资源数量和进程数量
#define NUM_RESOURCES 4
#define NUM_PROCESSES 5
// 定义各个资源的总量和可用量
int available[NUM_RESOURCES] = {10, 5, 7, 8};
int max[NUM_PROCESSES][NUM_RESOURCES] = {
{2, 2, 1, 1},
{3, 2, 2, 2},
{1, 2, 1, 3},
{2, 1, 2, 2},
{1, 1, 1, 1}
};
int allocation[NUM_PROCESSES][NUM_RESOURCES] = {
{2, 1, 0, 1},
{2, 0, 1, 0},
{1, 0, 1, 1},
{1, 0, 1, 0},
{0, 1, 0, 1}
};
int need[NUM_PROCESSES][NUM_RESOURCES];
// 检查进程是否可执行
bool is_safe(int process, int work[]) {
// 检查是否有足够的资源
for (int i = 0; i < NUM_RESOURCES; i++) {
if (need[process][i] > work[i]) {
return false;
}
}
return true;
}
// 执行安全检查
bool safety_algorithm() {
// 初始化工作向量和已完成进程集合
int work[NUM_RESOURCES];
for (int i = 0; i < NUM_RESOURCES; i++) {
work[i] = available[i];
}
bool finish[NUM_PROCESSES] = {false};
// 执行安全检查
int count = 0;
while (count < NUM_PROCESSES) {
bool found = false;
for (int i = 0; i < NUM_PROCESSES; i++) {
if (!finish[i] && is_safe(i, work)) {
found = true;
for (int j = 0; j < NUM_RESOURCES; j++) {
work[j] += allocation[i][j];
}
finish[i] = true;
count++;
printf("P%d ", i + 1);
break;
}
}
if (!found) {
return false;
}
}
return true;
}
int main() {
// 计算各个进程的需求量
for (int i = 0; i < NUM_PROCESSES; i++) {
for (int j = 0; j < NUM_RESOURCES; j++) {
need[i][j] = max[i][j] - allocation[i][j];
}
}
// 执行安全检查并输出结果
if (safety_algorithm()) {
printf("安全状态,存在安全序列\n");
} else {
printf("不安全状态,不存在安全序列\n");
}
return 0;
}
```
运行结果为:
```
P5 P1 P4 P3 P2 安全状态,存在安全序列
```
说明存在一个安全序列,可以保证所有进程都能够成功执行。如果更改available数组中的某个值,可能会导致无法找到安全序列,从而导致程序输出“不安全状态,不存在安全序列”。
阅读全文
相关推荐


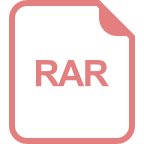



