JAVA现在在D盘中放有一个名为MultiFile的文件夹,请编写程序将此文件夹下的所有文件夹和文件复制到E盘的MultiFileCopy文件夹下。 注意:要求MultiFile文件夹下至少含有三层以上的文件夹,每层文件夹中都有文件。
时间: 2023-07-29 08:05:40 浏览: 115
以下是Java代码实现:
```java
import java.io.*;
public class FileCopy {
public static void main(String[] args) throws IOException {
String srcPath = "D:\\MultiFile";
String destPath = "E:\\MultiFileCopy";
File srcDir = new File(srcPath);
File destDir = new File(destPath);
if (!destDir.exists()) {
destDir.mkdirs();
}
copyDir(srcDir, destDir);
}
private static void copyDir(File srcDir, File destDir) throws IOException {
File[] files = srcDir.listFiles();
if (files != null) {
for (File file : files) {
if (file.isDirectory()) {
File newDestDir = new File(destDir, file.getName());
newDestDir.mkdirs();
copyDir(file, newDestDir);
} else {
File newDestFile = new File(destDir, file.getName());
copyFile(file, newDestFile);
}
}
}
}
private static void copyFile(File srcFile, File destFile) throws IOException {
try (InputStream in = new FileInputStream(srcFile);
OutputStream out = new FileOutputStream(destFile)) {
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
}
}
}
```
该程序使用了递归的方式,先判断源目录是否为文件夹,是则创建目标文件夹,然后遍历源目录中的所有文件和文件夹,如果是文件夹则递归调用自己,如果是文件则进行复制。复制过程中使用了字节流的方式,每次读取1024个字节进行复制。最终实现了将多层文件夹下的所有文件和文件夹复制到指定目录下的功能。
阅读全文
相关推荐
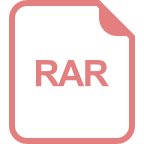













