1.ES6定义一个类(函数内部定义属性,,原型定义方法 ), 实现继承?
时间: 2024-05-01 08:17:06 浏览: 9
可以使用ES6的class语法来定义一个类,并使用extends关键字实现继承。在类内部使用constructor方法定义属性,使用prototype定义方法。
例如,定义一个Animal类,包含name和age属性,和eat方法:
```
class Animal {
constructor(name, age) {
this.name = name;
this.age = age;
}
eat(food) {
console.log(`${this.name} is eating ${food}.`);
}
}
```
然后定义一个继承自Animal的Cat类,包含color属性,和meow方法:
```
class Cat extends Animal {
constructor(name, age, color) {
super(name, age);
this.color = color;
}
meow() {
console.log(`${this.name} is meowing.`);
}
}
```
在Cat类中使用super关键字调用父类的constructor方法,继承父类的属性和方法。然后定义自己的属性和方法。
这样,就可以创建一个Cat对象,并调用其继承自Animal的属性和方法:
```
let myCat = new Cat("Kitty", 2, "black");
myCat.eat("fish"); // output: Kitty is eating fish.
myCat.meow(); // output: Kitty is meowing.
```
相关推荐
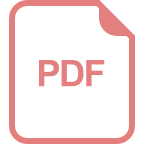














