用c语言设计一学生信息管理系统,学生信息包括:学号,姓名,年龄,性别,出生年月,地址,电话,E-mail使之能提供以下功能:系统以菜单方式工作学生信息录入功能(学生信息用文件保存)---输入学生信息浏览功能---输出查询、排序功能---算法,按学号查询,按姓名查询学生信息的删除与修改(可选项)要求能通过文件保存信息,程序支持信息导入和保存,功能要求界面比较美观;有一定的容错能力,比如输入的成绩不在0~100之间,就提示不合法,要求重新输入;最好用链表的方式实现。
时间: 2024-03-12 14:46:27 浏览: 192
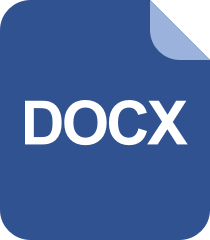
C语言程序设计-学校信息管理系统
好的,这是一个比较复杂的问题,需要耐心地分步骤来实现。下面是一些大致的思路和代码示例,希望能帮助你完成这个系统。
1. 定义学生信息的结构体
首先,我们需要定义一个结构体,用来表示学生的信息。这个结构体包含以下几个字段:
- 学号
- 姓名
- 年龄
- 性别
- 出生年月
- 地址
- 电话
- E-mail
具体的代码如下:
```c
#include <stdio.h>
#define MAX_NAME 20
#define MAX_ADDR 50
#define MAX_EMAIL 30
// 学生信息结构体
typedef struct {
int id; // 学号
char name[MAX_NAME]; // 姓名
int age; // 年龄
char gender; // 性别
char birthdate[11]; // 出生年月
char address[MAX_ADDR]; // 地址
char phone[12]; // 电话
char email[MAX_EMAIL]; // E-mail
} Student;
```
2. 定义文件操作函数
接下来,我们需要定义一些文件操作函数,用来读写学生信息。这些函数包括:
- `load_students`:从文件中读取学生信息,返回一个指向学生信息链表的指针。
- `save_students`:将学生信息链表保存到文件中。
- `add_student`:向学生信息链表中添加一个学生。
- `delete_student`:从学生信息链表中删除一个学生。
- `update_student`:更新学生信息链表中的一个学生。
具体的代码如下:
```c
#include <stdlib.h>
#include <string.h>
#define MAX_LINE 1024
// 学生信息链表结构体
typedef struct student_node {
Student student; // 学生信息
struct student_node* next; // 指向下一个学生信息的指针
} StudentNode;
// 从文件中读取学生信息
StudentNode* load_students(const char* filename) {
FILE* fp = fopen(filename, "r");
if (fp == NULL) {
return NULL;
}
StudentNode* head = NULL;
StudentNode* tail = NULL;
char line[MAX_LINE];
while (fgets(line, MAX_LINE, fp) != NULL) {
StudentNode* node = (StudentNode*)malloc(sizeof(StudentNode));
sscanf(line, "%d,%[^,],%d,%c,%[^,],%[^,],%[^,],%s",
&node->student.id, node->student.name, &node->student.age,
&node->student.gender, node->student.birthdate, node->student.address,
node->student.phone, node->student.email);
node->next = NULL;
if (tail == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
fclose(fp);
return head;
}
// 将学生信息链表保存到文件中
void save_students(const char* filename, StudentNode* head) {
FILE* fp = fopen(filename, "w");
if (fp == NULL) {
return;
}
for (StudentNode* node = head; node != NULL; node = node->next) {
fprintf(fp, "%d,%s,%d,%c,%s,%s,%s,%s\n",
node->student.id, node->student.name, node->student.age,
node->student.gender, node->student.birthdate, node->student.address,
node->student.phone, node->student.email);
}
fclose(fp);
}
// 向学生信息链表中添加一个学生
void add_student(StudentNode** head_ptr, Student student) {
StudentNode* node = (StudentNode*)malloc(sizeof(StudentNode));
node->student = student;
node->next = NULL;
if (*head_ptr == NULL) {
*head_ptr = node;
} else {
StudentNode* tail = *head_ptr;
while (tail->next != NULL) {
tail = tail->next;
}
tail->next = node;
}
}
// 从学生信息链表中删除一个学生
void delete_student(StudentNode** head_ptr, int id) {
StudentNode* prev = NULL;
StudentNode* curr = *head_ptr;
while (curr != NULL) {
if (curr->student.id == id) {
if (prev == NULL) {
*head_ptr = curr->next;
} else {
prev->next = curr->next;
}
free(curr);
break;
}
prev = curr;
curr = curr->next;
}
}
// 更新学生信息链表中的一个学生
void update_student(StudentNode* head, int id, Student student) {
for (StudentNode* node = head; node != NULL; node = node->next) {
if (node->student.id == id) {
node->student = student;
break;
}
}
}
```
3. 实现菜单界面
现在,我们需要实现一个菜单界面,让用户可以选择需要执行的操作。我们使用 `switch` 语句来实现这个菜单界面,具体的代码如下:
```c
#include <stdbool.h>
// 显示菜单界面,返回用户选择的操作代码
int show_menu() {
int choice;
printf("=================================\n");
printf("1. 添加学生信息\n");
printf("2. 删除学生信息\n");
printf("3. 修改学生信息\n");
printf("4. 浏览学生信息\n");
printf("5. 查询学生信息\n");
printf("6. 按学号排序\n");
printf("7. 按姓名排序\n");
printf("8. 退出程序\n");
printf("=================================\n");
printf("请选择要执行的操作:");
scanf("%d", &choice);
return choice;
}
```
4. 实现学生信息录入功能
首先,我们需要实现学生信息录入功能。这个功能可以让用户输入学生的信息,并将其保存到文件中。具体的代码如下:
```c
// 学生信息录入功能
void add_student_info() {
Student student;
printf("请输入学号:");
scanf("%d", &student.id);
printf("请输入姓名:");
scanf("%s", student.name);
printf("请输入年龄:");
scanf("%d", &student.age);
printf("请输入性别:");
scanf(" %c", &student.gender);
printf("请输入出生年月(格式为 YYYY-MM-DD):");
scanf("%s", student.birthdate);
printf("请输入地址:");
scanf("%s", student.address);
printf("请输入电话:");
scanf("%s", student.phone);
printf("请输入 E-mail:");
scanf("%s", student.email);
add_student(&head, student);
save_students("students.dat", head);
}
```
5. 实现浏览学生信息功能
接下来,我们需要实现浏览学生信息功能。这个功能可以将保存在文件中的学生信息读取出来,并在屏幕上显示出来。具体的代码如下:
```c
// 浏览学生信息功能
void view_student_info() {
printf("学号\t姓名\t年龄\t性别\t出生年月\t地址\t电话\tE-mail\n");
printf("==========================================================================\n");
for (StudentNode* node = head; node != NULL; node = node->next) {
printf("%d\t%s\t%d\t%c\t%s\t%s\t%s\t%s\n",
node->student.id, node->student.name, node->student.age,
node->student.gender, node->student.birthdate, node->student.address,
node->student.phone, node->student.email);
}
printf("\n");
}
```
6. 实现查询学生信息功能
接下来,我们需要实现查询学生信息功能。这个功能可以按照学号或者姓名查询学生的信息,并将结果显示在屏幕上。具体的代码如下:
```c
// 按学号查询学生信息
void search_by_id() {
int id;
printf("请输入要查询的学号:");
scanf("%d", &id);
bool found = false;
printf("学号\t姓名\t年龄\t性别\t出生年月\t地址\t电话\tE-mail\n");
printf("==========================================================================\n");
for (StudentNode* node = head; node != NULL; node = node->next) {
if (node->student.id == id) {
printf("%d\t%s\t%d\t%c\t%s\t%s\t%s\t%s\n",
node->student.id, node->student.name, node->student.age,
node->student.gender, node->student.birthdate, node->student.address,
node->student.phone, node->student.email);
found = true;
}
}
if (!found) {
printf("没有找到该学生的信息。\n");
}
printf("\n");
}
// 按姓名查询学生信息
void search_by_name() {
char name[MAX_NAME];
printf("请输入要查询的姓名:");
scanf("%s", name);
bool found = false;
printf("学号\t姓名\t年龄\t性别\t出生年月\t地址\t电话\tE-mail\n");
printf("==========================================================================\n");
for (StudentNode* node = head; node != NULL; node = node->next) {
if (strcmp(node->student.name, name) == 0) {
printf("%d\t%s\t%d\t%c\t%s\t%s\t%s\t%s\n",
node->student.id, node->student.name, node->student.age,
node->student.gender, node->student.birthdate, node->student.address,
node->student.phone, node->student.email);
found = true;
}
}
if (!found) {
printf("没有找到该学生的信息。\n");
}
printf("\n");
}
```
7. 实现排序功能
最后,我们需要实现排序功能。这个功能可以按照学号或者姓名对学生信息进行排序,并将排序结果保存到文件中。具体的代码如下:
```c
#include <stdbool.h>
// 按学号排序
void sort_by_id() {
bool swapped = true;
while (swapped) {
swapped = false;
for (StudentNode* node = head; node != NULL && node->next != NULL; node = node->next) {
if (node->student.id > node->next->student.id) {
Student temp = node->student;
node->student = node->next->student;
node->next->student = temp;
swapped = true;
}
}
}
save_students("students.dat", head);
}
// 按姓名排序
void sort_by_name() {
bool swapped = true;
while (swapped) {
swapped = false;
for (StudentNode* node = head; node != NULL && node->next != NULL; node = node->next) {
if (strcmp(node->student.name, node->next->student.name) > 0) {
Student temp = node->student;
node->student = node->next->student;
node->next->student = temp;
swapped = true;
}
}
}
save_students("students.dat", head);
}
```
完整的代码如下:
阅读全文
相关推荐
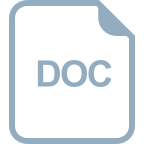
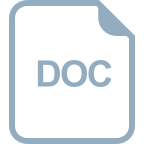









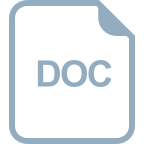
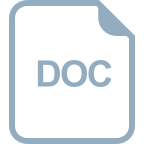
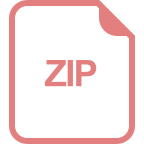
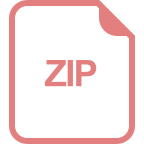