帮我写一个STM32F407 TFT LCD显示在指定圆形区域填充颜色的代码
时间: 2023-08-28 21:45:33 浏览: 239
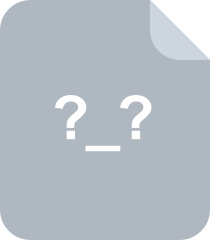
stm32f407lcd代码
好的,以下是一个基本的示例代码:
```c
#include "stm32f4xx.h"
#include "stm32f4xx_gpio.h"
#include "stm32f4xx_rcc.h"
#include "stm32f4xx_spi.h"
#define LCD_CS_HIGH() GPIO_SetBits(GPIOB, GPIO_Pin_12)
#define LCD_CS_LOW() GPIO_ResetBits(GPIOB, GPIO_Pin_12)
#define LCD_DC_HIGH() GPIO_SetBits(GPIOB, GPIO_Pin_13)
#define LCD_DC_LOW() GPIO_ResetBits(GPIOB, GPIO_Pin_13)
#define SPI_TIMEOUT 1000
void LCD_WriteCommand(uint8_t cmd);
void LCD_WriteData(uint8_t data);
void LCD_SetAddrWindow(uint16_t x0, uint16_t y0, uint16_t x1, uint16_t y1);
void LCD_FillCircle(uint16_t x0, uint16_t y0, uint16_t r, uint16_t color);
int main(void)
{
/* 初始化 GPIO 和 SPI */
GPIO_InitTypeDef GPIO_InitStruct;
SPI_InitTypeDef SPI_InitStruct;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOB, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_SPI1, ENABLE);
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_3 | GPIO_Pin_5 | GPIO_Pin_7;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOB, &GPIO_InitStruct);
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_12 | GPIO_Pin_13;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOB, &GPIO_InitStruct);
GPIO_PinAFConfig(GPIOB, GPIO_PinSource3, GPIO_AF_SPI1);
GPIO_PinAFConfig(GPIOB, GPIO_PinSource5, GPIO_AF_SPI1);
GPIO_PinAFConfig(GPIOB, GPIO_PinSource7, GPIO_AF_SPI1);
SPI_InitStruct.SPI_Direction = SPI_Direction_2Lines_FullDuplex;
SPI_InitStruct.SPI_Mode = SPI_Mode_Master;
SPI_InitStruct.SPI_DataSize = SPI_DataSize_8b;
SPI_InitStruct.SPI_CPOL = SPI_CPOL_Low;
SPI_InitStruct.SPI_CPHA = SPI_CPHA_1Edge;
SPI_InitStruct.SPI_NSS = SPI_NSS_Soft;
SPI_InitStruct.SPI_BaudRatePrescaler = SPI_BaudRatePrescaler_16;
SPI_InitStruct.SPI_FirstBit = SPI_FirstBit_MSB;
SPI_InitStruct.SPI_CRCPolynomial = 7;
SPI_Init(SPI1, &SPI_InitStruct);
SPI_Cmd(SPI1, ENABLE);
/* 初始化 TFT LCD */
LCD_CS_HIGH();
LCD_DC_HIGH();
LCD_WriteCommand(0x01); // 软件复位
Delay(50);
LCD_WriteCommand(0x11); // 退出睡眠模式
Delay(50);
LCD_WriteCommand(0x3A); // 设置像素数据格式
LCD_WriteData(0x05); // RGB565
LCD_WriteCommand(0xB1); // 设置 Frame Rate Control
LCD_WriteData(0x00);
LCD_WriteData(0x10);
LCD_WriteCommand(0xB4); // 设置 Display Inversion Control
LCD_WriteData(0x00);
LCD_WriteCommand(0xC0); // 设置 Power Control 1
LCD_WriteData(0x0D);
LCD_WriteData(0x0D);
LCD_WriteData(0x0D);
LCD_WriteCommand(0xC1); // 设置 Power Control 2
LCD_WriteData(0x43);
LCD_WriteData(0x00);
LCD_WriteCommand(0xC5); // 设置 VCOM Control 1
LCD_WriteData(0x00);
LCD_WriteData(0x12);
LCD_WriteData(0x80);
LCD_WriteCommand(0xC7); // 设置 VCOM Control 2
LCD_WriteData(0x1D);
LCD_WriteCommand(0x36); // 设置 Memory Access Control
LCD_WriteData(0x48); // BGR
LCD_WriteCommand(0x29); // 开启显示
/* 在指定区域填充圆形 */
LCD_FillCircle(120, 160, 50, 0xF800); // (120,160)为圆心,50为半径,0xF800为颜色
while (1)
{
}
}
void LCD_WriteCommand(uint8_t cmd)
{
LCD_CS_LOW();
LCD_DC_LOW();
SPI_I2S_SendData(SPI1, cmd);
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_BSY) != RESET);
LCD_CS_HIGH();
}
void LCD_WriteData(uint8_t data)
{
LCD_CS_LOW();
LCD_DC_HIGH();
SPI_I2S_SendData(SPI1, data);
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_BSY) != RESET);
LCD_CS_HIGH();
}
void LCD_SetAddrWindow(uint16_t x0, uint16_t y0, uint16_t x1, uint16_t y1)
{
LCD_WriteCommand(0x2A); // 设置列地址
LCD_WriteData(x0 >> 8);
LCD_WriteData(x0);
LCD_WriteData(x1 >> 8);
LCD_WriteData(x1);
LCD_WriteCommand(0x2B); // 设置行地址
LCD_WriteData(y0 >> 8);
LCD_WriteData(y0);
LCD_WriteData(y1 >> 8);
LCD_WriteData(y1);
LCD_WriteCommand(0x2C); // 写入数据
}
void LCD_FillCircle(uint16_t x0, uint16_t y0, uint16_t r, uint16_t color)
{
int x = r, y = 0;
int radiusError = 1 - x;
while (x >= y)
{
LCD_SetAddrWindow(x0 - x, y0 + y, x0 + x, y0 + y); // 上半部分
for (int i = 0; i < 2 * x + 1; i++)
{
LCD_WriteData(color >> 8);
LCD_WriteData(color);
}
LCD_SetAddrWindow(x0 - x, y0 - y, x0 + x, y0 - y); // 下半部分
for (int i = 0; i < 2 * x + 1; i++)
{
LCD_WriteData(color >> 8);
LCD_WriteData(color);
}
y++;
if (radiusError < 0)
{
radiusError += 2 * y + 1;
}
else
{
x--;
radiusError += 2 * (y - x + 1);
}
}
}
```
以上代码假定液晶屏使用 SPI 接口,且连接在 GPIOB 的 PB3、PB5、PB7 三个引脚上,CS、DC 分别连接在 GPIOB 的 PB12、PB13 引脚上。在主函数中,首先对 GPIO 和 SPI 进行初始化,然后初始化 TFT LCD,最后调用 LCD_FillCircle 函数在指定区域填充颜色。LCD_FillCircle 函数中使用中点画圆法绘制圆形,具体实现可以参考注释。
阅读全文
相关推荐
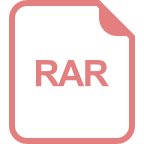
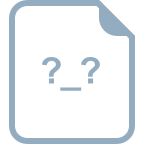
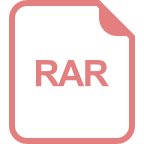
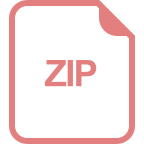
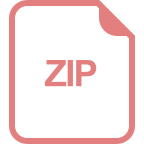
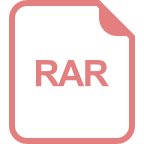
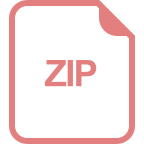
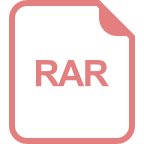
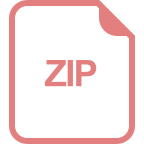
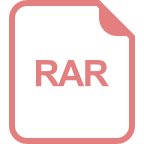
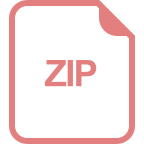
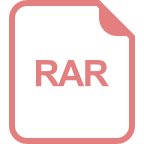
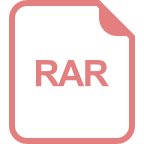
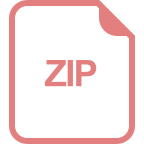
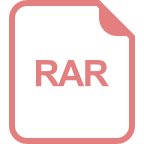
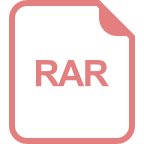