vue 鼠标在父元素内移动,子元素跟随鼠标移动,且子元素不能超出父元素边框
时间: 2023-11-30 18:02:18 浏览: 119
你可以使用 `@mousemove` 监听鼠标移动事件,然后通过计算鼠标位置和父元素位置的差值,来设置子元素的位置。
具体实现步骤如下:
1. 在父元素上添加 `@mousemove` 监听事件,获取鼠标位置 `event.pageX` 和 `event.pageY` 以及父元素位置 `parentNode.offsetLeft` 和 `parentNode.offsetTop`。
2. 计算鼠标位置和父元素位置的差值 `deltaX` 和 `deltaY`。
3. 判断子元素的位置是否超出父元素的边界,如果超出则设置子元素的位置为父元素的边界位置。
4. 否则,设置子元素的位置为鼠标位置和父元素位置的差值。
代码示例:
```
<template>
<div class="parent" @mousemove="handleMouseMove">
<div class="child" :style="{left: childLeft + 'px', top: childTop + 'px'}"></div>
</div>
</template>
<script>
export default {
data() {
return {
childLeft: 0,
childTop: 0
}
},
methods: {
handleMouseMove(event) {
const parentNode = event.currentTarget
const mouseX = event.pageX
const mouseY = event.pageY
const parentX = parentNode.offsetLeft
const parentY = parentNode.offsetTop
const deltaX = mouseX - parentX
const deltaY = mouseY - parentY
const childWidth = 100 // 子元素宽度
const childHeight = 100 // 子元素高度
const maxX = parentNode.clientWidth - childWidth // 子元素最大可移动的横向距离
const maxY = parentNode.clientHeight - childHeight // 子元素最大可移动的纵向距离
let childLeft = deltaX
let childTop = deltaY
if (childLeft < 0) {
childLeft = 0
} else if (childLeft > maxX) {
childLeft = maxX
}
if (childTop < 0) {
childTop = 0
} else if (childTop > maxY) {
childTop = maxY
}
this.childLeft = childLeft
this.childTop = childTop
}
}
}
</script>
<style>
.parent {
position: relative;
width: 300px;
height: 300px;
border: 1px solid #ccc;
}
.child {
position: absolute;
width: 100px;
height: 100px;
background-color: red;
}
</style>
```
阅读全文
相关推荐
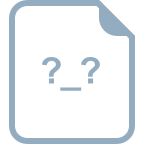
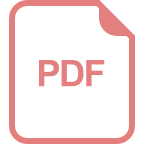
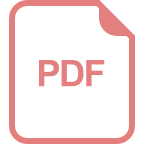
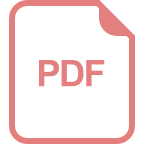
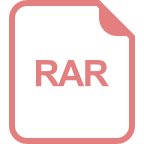
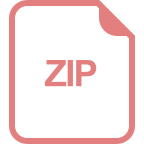
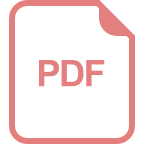
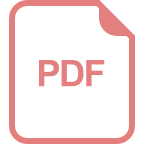
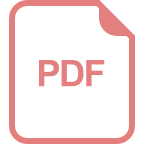
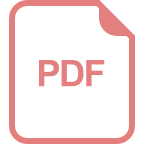
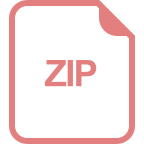
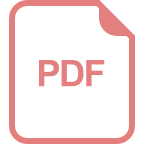
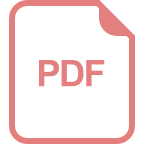
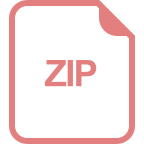
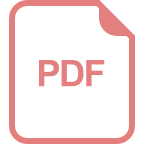
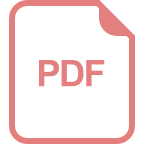