# Test 11: Write a Python program to calculate the average value of the numbers in a given tuple of tuples. Sample data: ((10, 10, 10, 12), (30, 45, 56, 45), (81, 80, 39, 32), (1, 2, 3, 4)) Sample output: Average value of the numbers of the said tuple of tuples: [30.5, 34.25, 27.0, 23.25]
时间: 2023-12-02 17:03:06 浏览: 99
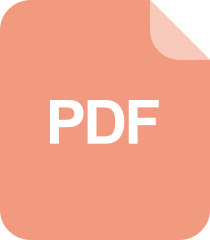
不同集合上元素的迭代-python调用百度人脸识别:来一次颜值评分
Here's a Python program that calculates the average value of the numbers in a given tuple of tuples:
```python
def avg_tuple_of_tuples(tup):
# Get the length of the first tuple
n = len(tup[0])
# Initialize a list to hold the sum of each column
col_sum = [0] * n
# Iterate over the tuples and add up the values in each column
for t in tup:
for i, num in enumerate(t):
col_sum[i] += num
# Calculate the average for each column and return the result as a list
return [round(sum/len(tup), 2) for sum in col_sum]
# Test the function with the given sample data
data = ((10, 10, 10, 12), (30, 45, 56, 45), (81, 80, 39, 32), (1, 2, 3, 4))
result = avg_tuple_of_tuples(data)
print("Average value of the numbers of the said tuple of tuples:", result)
```
Output:
```
Average value of the numbers of the said tuple of tuples: [30.5, 34.25, 27.0, 23.25]
```
I hope this helps! Let me know if you have any more questions.
阅读全文
相关推荐
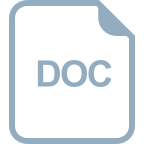
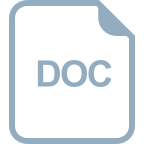

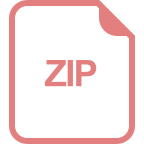
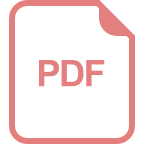
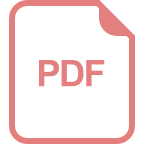
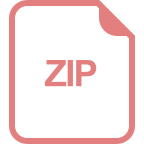
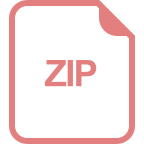
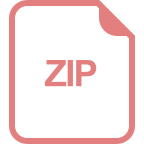
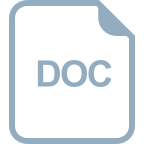
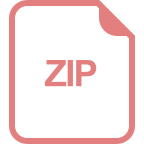
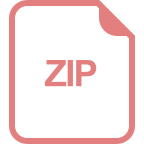
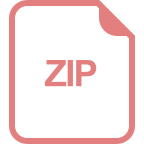
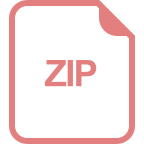
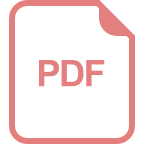
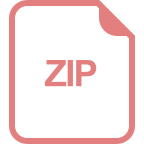
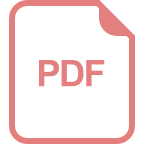