java设计一个名为DateUtil的类,包含年、月、日属性并实现日期合法性检查、闰年判断、日期增减、日期比较等功能。通过主类测试不同场景下的日期操作,如求下n天、前n天及日期间隔天数。
时间: 2024-12-04 19:29:37 浏览: 13
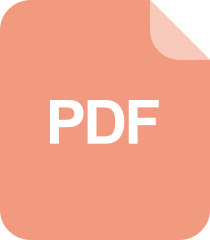
PHP时间日期增减操作示例【date strtotime实现加一天、加一月等操作】
在Java中,你可以创建一个名为`DateUtil`的工具类,它将包含三个私有字段`year`、`month`和`day`分别代表年份、月份和日期。为了实现日期合法性检查、闰年判断、日期增减以及日期比较,我们需要提供一些公共方法:
```java
import java.time.LocalDate;
import java.util.Date;
public class DateUtil {
private int year;
private int month;
private int day;
// 构造函数接受整数参数,并设置日期
public DateUtil(int year, int month, int day) {
this.year = year;
this.month = month;
this.day = day;
checkValidDate();
}
// 验证日期是否合法
private void checkValidDate() {
if (year < 0 || month <= 0 || month > 12 || day < 1 || day > getDaysInMonth(year, month)) {
throw new IllegalArgumentException("Invalid date");
}
}
// 判断是否为闰年
public boolean isLeapYear() {
return LocalDate.of(year, 2, 29).isLeapYear();
}
// 日期加减功能
public DateUtil addDays(int days) {
year += days / 365;
days %= 365;
while (days >= 365) {
days -= getMonthsInYear(year);
}
month += days / getDaysInMonth(year, month);
days %= getDaysInMonth(year, month);
day += days;
return this;
}
// 获取两个日期之间的天数差
public int getDifference(DateUtil other) {
long diff = this.toDate().getTime() - other.toDate().getTime();
return (int) Math.abs(diff / (24 * 60 * 60 * 1000));
}
// 比较日期
public int compare(DateUtil other) {
return Integer.compare(this.getDayOfMonth(), other.getDayOfMonth()) ||
Integer.compare(this.getMonth(), other.getMonth()) ||
Integer.compare(this.getYear(), other.getYear());
}
// 转换为LocalDate用于进一步操作
private LocalDate toDate() {
return LocalDate.of(year, month + 1, day);
}
// 获取给定年份和月份的总天数
private int getDaysInMonth(int year, int month) {
switch (month) {
case 2:
return isLeapYear() ? 29 : 28;
case 4:
case 6:
case 9:
case 11:
return 30;
default:
return 31;
}
}
// 获取给定年份的月份数量
private int getMonthsInYear(int year) {
return LocalDate.of(year, 12, 31).getMonthValue() - 1;
}
// Getters and setters for the properties...
}
```
你可以通过下面的方式在`Main`类中测试这个`DateUtil`:
```java
public class Main {
public static void main(String[] args) {
DateUtil today = new DateUtil(2023, 3, 15);
System.out.println(today.isLeapYear()); // 输出是否为闰年
today.addDays(7); // 求下7天的日期
DateUtil tomorrow = new DateUtil(2023, 3, 22);
System.out.println(today.getDifference(tomorrow)); // 输出日期间隔
System.out.println(today.compareTo(tomorrow)); // 输出日期比较结果
}
}
```
阅读全文
相关推荐
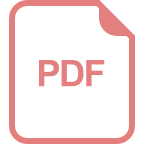
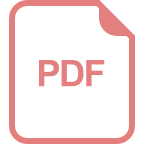
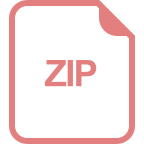
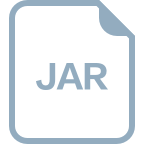
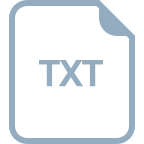
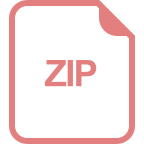
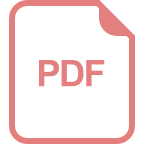
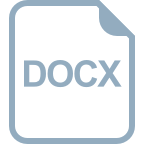
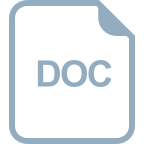
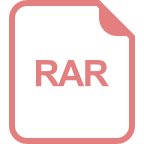
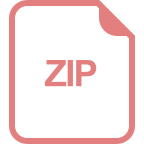
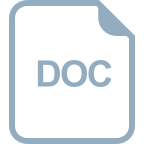
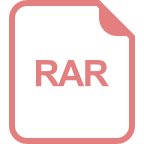
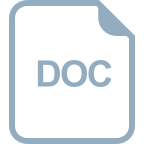
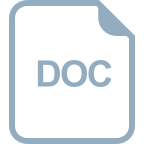
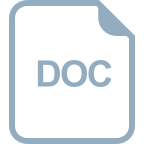
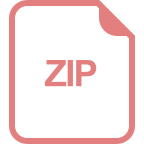