C#使用FTP上传图片
时间: 2024-12-10 20:17:49 浏览: 24
在C#中,使用FTP(File Transfer Protocol)上传图片通常涉及到两个步骤:首先创建一个FTP连接,然后使用FTP命令将图片文件发送到服务器上。以下是一个简单的示例:
```csharp
using System;
using System.IO;
using System.Net.FtpClient;
class Program
{
static void Main(string[] args)
{
string ftpServer = "ftp.example.com";
int ftpPort = 21; // FTP默认端口
string username = "your_username";
string password = "your_password";
string localFilePath = @"C:\path\to\image.jpg"; // 图片本地路径
string remoteDirectory = "/path/to/remote/directory"; // 服务器目录
FtpWebRequest request = (FtpWebRequest)WebRequest.Create($"ftp://{ftpServer}:{ftpPort}/{remoteDirectory}");
request.Method = WebRequestMethods.Ftp.UploadFile;
request.Credentials = new NetworkCredential(username, password);
request.UseBinary = true;
using (Stream requestStream = request.GetRequestStream())
{
File.Copy(localFilePath, requestStream);
}
try
{
FtpWebResponse response = (FtpWebResponse)request.GetResponse();
Console.WriteLine("Upload successful, server response: " + response.StatusDescription);
response.Close();
}
catch (Exception ex)
{
Console.WriteLine($"Error uploading file: {ex.Message}");
}
}
}
```
在这个例子中,你需要替换`ftpServer`, `username`, `password`, `localFilePath`以及`remoteDirectory`为你实际的FTP服务器信息和文件路径。程序会建立FTP连接,打开请求流,读取本地图片并将其写入到远程服务器指定的目录。
阅读全文
相关推荐










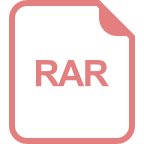




