时钟程序的基础上实现有秒计时的数字万年历时钟。 必选内容:年、月、日、小时、分钟、秒的模拟; 可选内容:星期、农历的模拟。 2.提示: 给时钟程序加上一个表示秒的Display; 给时钟程序加上一个表示日期的Display(注意每月天数28、29、30、31); 给时钟程序加上一个表示月份的Display; 给时钟程序加上一个表示年份的Display; 为Clock增加以下public的成员函数: public Clock(int hour, int minute, int second, int year, int month, int day); 3.输出: 输出String的值,以“yyyy:mm:dd:hh:mm:ss“的形式表示当前时间。这里除了年份占四位外,每个数值都占据两位,不足两位时补0。如“00:01:22"。注意其中的冒号是西文的,不是中文的。(String.format()可以用和printf一样的方式来格式化一个字符串。)
时间: 2024-04-13 10:30:11 浏览: 183
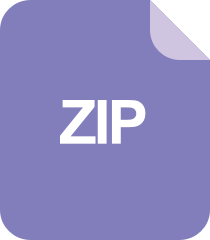
数字钟设计,包括万年历,闹钟,倒计时,秒表,进制转换

根据您的要求,以下是一个基于时钟程序的数字万年历时钟的Java代码:
```java
import java.util.Calendar;
public class Clock {
private int hour;
private int minute;
private int second;
private int year;
private int month;
private int day;
public Clock(int hour, int minute, int second, int year, int month, int day) {
this.hour = hour;
this.minute = minute;
this.second = second;
this.year = year;
this.month = month;
this.day = day;
}
public void tick() {
second++;
if (second >= 60) {
second = 0;
minute++;
if (minute >= 60) {
minute = 0;
hour++;
if (hour >= 24) {
hour = 0;
nextDay();
}
}
}
}
private void nextDay() {
day++;
if (day > getDaysInMonth(year, month)) {
day = 1;
month++;
if (month > 12) {
month = 1;
year++;
}
}
}
private int getDaysInMonth(int year, int month) {
int[] daysInMonth = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
if (month == 2 && isLeapYear(year)) {
return 29;
}
return daysInMonth[month - 1];
}
private boolean isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
public String toString() {
return String.format("%04d:%02d:%02d:%02d:%02d:%02d", year, month, day, hour, minute, second);
}
public static void main(String[] args) throws InterruptedException {
// 获取当前时间
Calendar now = Calendar.getInstance();
// 创建时钟对象
Clock clock = new Clock(now.get(Calendar.HOUR_OF_DAY), now.get(Calendar.MINUTE),
now.get(Calendar.SECOND), now.get(Calendar.YEAR), now.get(Calendar.MONTH) + 1,
now.get(Calendar.DAY_OF_MONTH));
// 输出当前时间
System.out.println(clock);
// 模拟时钟运行
while (true) {
Thread.sleep(1000); // 休眠1秒
clock.tick();
System.out.println(clock);
}
}
}
```
这个代码创建了一个名为`Clock`的类,通过构造函数传入初始的小时、分钟、秒、年、月、日。`tick()`方法用于模拟时钟的运行,每次调用该方法时,秒数加1,如果秒数超过60,则进位到分钟,并依次类推。`nextDay()`方法用于模拟日期的变化,当天数超过当前月份的天数时,进位到下一个月。`toString()`方法用于将时间格式化为字符串。
在`main()`方法中,首先获取当前时间,并使用当前时间创建一个`Clock`对象。然后,通过循环不断模拟时钟的运行,每秒钟调用`tick()`方法,并输出当前时间。程序会一直运行下去,直到手动停止。
注意:由于这是一个无限循环的程序,请谨慎运行。如果您想停止程序,请使用合适的方式终止程序的运行。
阅读全文
相关推荐
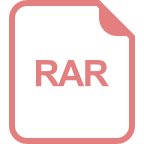
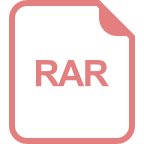
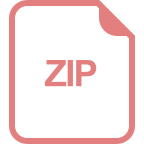
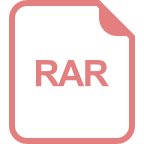
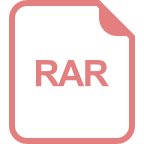
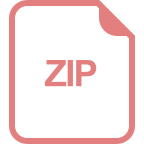
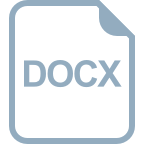
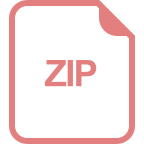
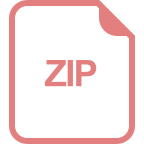
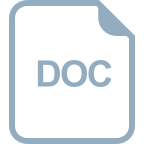
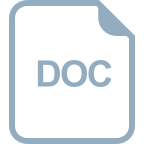
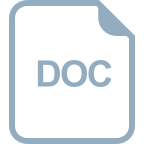
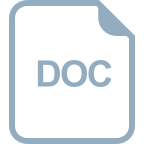
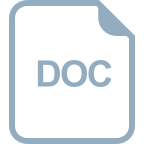
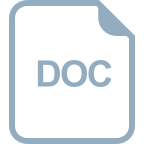
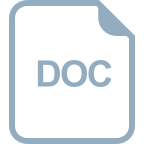
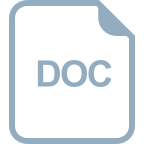