unity 在字符串中提取数字
时间: 2024-10-15 10:12:33 浏览: 38
Unity引擎本身并不提供直接的函数用于在字符串中提取数字,但它支持字符串操作,你可以通过编写脚本来实现这个功能。常见的做法是使用正则表达式(RegEx)来匹配和提取数字。例如,可以使用`Regex.Match`和`Regex.Replace`方法:
```csharp
using System;
using System.Text.RegularExpressions;
public string ExtractNumbers(string input) {
string pattern = @"\d+"; // 匹配一串数字
Regex regex = new Regex(pattern);
MatchCollection matches = regex.Matches(input);
StringBuilder result = new StringBuilder();
foreach (Match match in matches) {
result.Append(match.Value); // 将找到的数字添加到结果字符串
}
return result.ToString(); // 返回包含所有数字的新字符串
}
// 使用示例
string textWithNumbers = "Hello123World456";
string numbersOnly = ExtractNumbers(textWithNumbers);
```
相关问题
unity提取字符串中的数字
在Unity中,如果你想要从字符串中提取数字,可以使用正则表达式(RegEx)或者内置的一些文本处理函数。这里是一个简单的示例,我们将使用C#的`Regex`类:
```csharp
using System;
using System.Text.RegularExpressions;
public class ExtractNumbersExample {
public static void Main() {
string inputString = "包含数字如123、456和789的字符串";
string regexPattern = @"\d+"; // 匹配一串连续的数字
// 使用正则表达式查找所有匹配的数字
MatchCollection matches = Regex.Matches(inputString, regexPattern);
foreach (Match match in matches) {
int number = Int32.Parse(match.Value); // 将匹配到的字符串转换为整数
Console.WriteLine("找到的数字: " + number);
}
}
}
```
在这个例子中,`Regex.Matches()`会返回所有匹配到数字的所有组。然后你可以遍历这些匹配项并使用`Int32.Parse()`将它们转换为整数。
unity如何提取text文本里的数字
在Unity中,如果你需要从Text组件内的文本中提取数字,可以按照以下步骤操作:
1. 获取Text组件:首先,你需要获取包含数字的Text组件。你可以通过`GameObject.Find()`、` GetComponent<Text>()` 或者存储的游戏对象引用直接访问它。
```csharp
Text textComponent = GetComponent<Text>();
```
2. 提取文本字符串:使用`textComponent.text`属性获取当前显示的文字内容。
```csharp
string rawText = textComponent.text;
```
3. 使用正则表达式:由于文本可能包含非数字字符,可以使用`Regex`类和正则表达式模式来匹配和提取数字。例如,`@"\d+"`可以匹配一串连续的数字。
```csharp
using System.Text.RegularExpressions;
Regex regex = new Regex(@"\d+");
MatchCollection matches = regex.Matches(rawText);
```
4. 遍历并获取数字:`matches`现在是一个`MatchCollection`,你可以遍历这个集合,提取每个匹配到的数字。
```csharp
foreach (Match match in matches)
{
string digit = match.Value; // 现在digit变量就包含了匹配到的一个数字
}
```
阅读全文
相关推荐
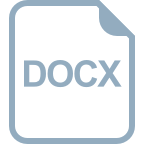
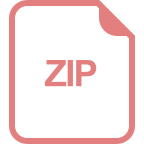
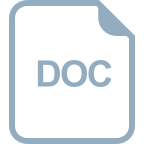
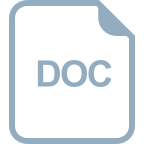
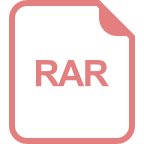
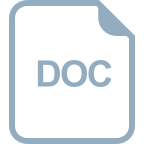
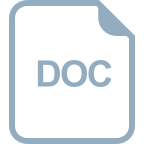
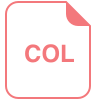




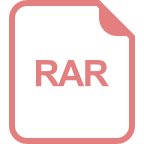
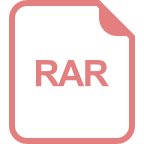
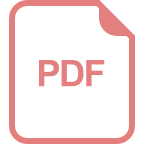