matlab ICP
时间: 2023-10-24 19:34:02 浏览: 50
ICP(Iterative Closest Point)是一种常用于点云配准的算法,用于将两个或多个点云之间进行对齐和匹配。在Matlab中,有多种方式可以实现ICP算法。
一种常见的实现方式是使用点云处理工具箱(PointCloud Processing Toolbox),该工具箱提供了许多用于点云处理和配准的函数。其中包括`pcfit`函数,可以用于点云的配准和拟合。
另一种方式是自己编写ICP算法的代码。ICP算法的基本思想是迭代地寻找最佳的变换矩阵,将源点云与目标点云进行对齐。这个过程包括了计算最近邻点对、计算变换矩阵、应用变换等步骤。
在Matlab中,可以使用函数`pcregistericp`来实现ICP算法。该函数可以根据需要选择不同的参数,如初始变换矩阵、最大迭代次数、收敛阈值等。
这只是ICP算法在Matlab中的一些简单介绍,如果你有更具体的问题或需求,可以提供更多细节,我会尽力回答。
相关问题
matlab icp
ICP (Iterative Closest Point) 是一种在点云配准中常用的算法,用于寻找两个或多个点云之间的最佳变换以使它们尽可能地对齐。在 MATLAB 中,您可以使用 `pcfitransform` 函数来执行 ICP。
以下是一个简单的示例,展示了如何使用 MATLAB 中的 ICP 算法来配准两个点云:
```matlab
% 创建两个示例点云
ptCloud1 = pointCloud(pointCloudData1);
ptCloud2 = pointCloud(pointCloudData2);
% 创建 ICP 对象
icp = pcregistericp;
% 设置 ICP 相关参数
icp.MaximumIterations = 50;
icp.TransformationEstimate = 'rigid';
% 执行 ICP 配准
[tform, ~] = icp.register(ptCloud1, ptCloud2);
% 应用变换到第二个点云
ptCloudAligned = pctransform(ptCloud2, tform);
% 可视化结果
figure
pcshow(ptCloud1, 'r');
hold on
pcshow(ptCloudAligned, 'b');
hold off
```
在这个示例中,`ptCloud1` 和 `ptCloud2` 分别代表两个输入的点云数据。然后创建了一个 ICP 对象 `icp`,并设置了一些参数,比如最大迭代次数和变换估计类型。接下来,使用 `register` 函数执行 ICP 配准,并得到变换矩阵 `tform`。最后,使用 `pctransform` 函数将第二个点云应用变换,并可视化配准结果。
请注意,这只是 ICP 算法的一个简单示例,您可能需要根据自己的具体需求进行更多的参数调整和优化。
MATLAB ICP 代码
ICP(Iterative Closest Point)算法是一种常用的点云配准算法,MATLAB提供了ICP算法的实现,可以通过点云数据进行三维重建、三维建模等应用。MATLAB ICP算法的实现主要包括以下步骤:
1. 加载点云数据并初始化初始变换矩阵;
2. 选择匹配方法(点到点或点到平面)并设置匹配参数;
3. 迭代计算变换矩阵,直到收敛;
4. 输出最终变换矩阵,并将原始点云进行变换得到配准后的点云。
在MATLAB中,可以通过PointCloudRegistration类来实现ICP算法。具体用法可以参考以下示例代码:
```
% 加载点云数据
ptCloud1 = pcread('ptCloud1.ply');
ptCloud2 = pcread('ptCloud2.ply');
% 初始化初始变换矩阵
tform = affine3d(eye(4));
% 选择匹配方法并设置匹配参数
matchMethod = 'pointToPoint';
matchParam.MaxIterations = 100;
matchParam.Tolerance = [0.001 0.001 0.001];
matchParam.DistanceThreshold = 0.02;
% 迭代计算变换矩阵
[tform, ptCloud2Registered] = pcregistericp(ptCloud2, ptCloud1, matchMethod, ...
'InitialTransform', tform, 'Metric', 'pointToPoint', 'MatchTolerance', matchParam);
% 输出最终变换矩阵
disp(tform.T);
% 可视化配准后的点云
figure;
pcshowpair(ptCloud1, ptCloud2Registered, 'VerticalAxis', 'Y', 'VerticalAxisDir', 'down');
xlabel('X'); ylabel('Y'); zlabel('Z');
title('ICP配准结果');
```
相关推荐
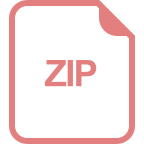
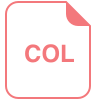











