请用.Net Core帮我写一段快排代码,并帮我讲解下实现逻辑
时间: 2024-11-13 10:22:18 浏览: 16
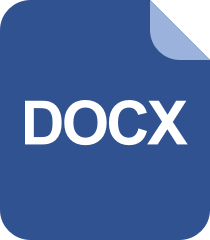
【ASP.NET编程知识】.NET Core实现分表分库、读写分离的通用 Repository功能.docx
在.NET Core中,快速排序(QuickSort)是一种常用的高效排序算法。以下是一个简单的快速排序实现:
```csharp
using System;
public class QuickSort
{
public static void QuickSort(int[] arr, int left = 0, int right = int.MaxValue)
{
if (left < right)
{
int pivotIndex = Partition(arr, left, right);
QuickSort(arr, left, pivotIndex - 1); // 对左半部分递归排序
QuickSort(arr, pivotIndex + 1, right); // 对右半部分递归排序
}
}
private static int Partition(int[] arr, int left, int right)
{
int pivotValue = arr[right]; // 选择最右边的元素作为基准
int i = left - 1; // 指针,初始位置在数组左侧
for (int j = left; j < right; j++)
{
if (arr[j] <= pivotValue) // 如果当前元素小于等于基准
{
i++; // 移动指针到右侧
Swap(ref arr[i], ref arr[j]); // 交换两个元素
}
}
Swap(ref arr[i + 1], ref arr[right]); // 将基准放在正确的位置上
return i + 1;
}
private static void Swap(ref int a, ref int b)
{
int temp = a;
a = b;
b = temp;
}
}
// 使用示例
public static void Main()
{
int[] numbers = { 9, 7, 5, 11, 12, 2, 14, 3, 10 };
QuickSort(numbers, 0, numbers.Length - 1);
Console.WriteLine("Sorted array:");
foreach (var num in numbers)
{
Console.Write(num + " ");
}
}
```
实现逻辑简述:
1. `QuickSort`方法接收一个整数数组、起始索引(left)和结束索引(right)。如果left小于right,说明还有待排序的部分。
2. `Partition`函数是关键步骤,它将数组分为两部分:左边的元素都小于等于基准值(pivot),右边的元素都大于基准。它通过一个指针i从左向右遍历,遇到小于等于pivot的元素就交换其与指针位置的元素,然后移动指针i。
3. 最后,当遍历完数组后,基准值位于正确的位置,这时基准右边的所有元素都是比它大的,左边则是比它小的或相等的。然后对左右两部分递归地进行快速排序。
阅读全文
相关推荐
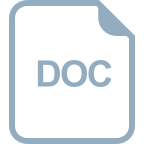
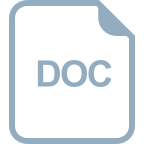
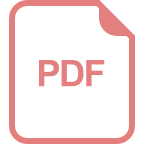
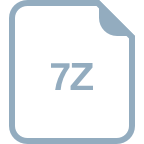
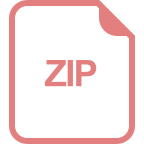
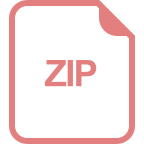
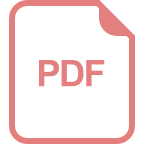
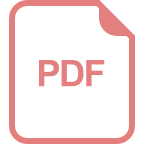
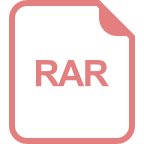
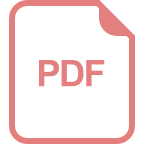
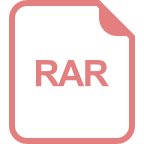
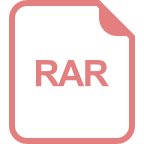
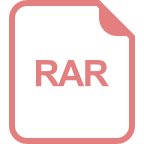
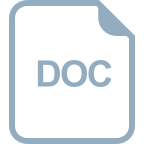
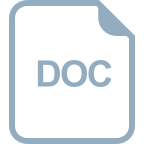
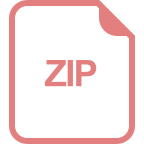