Linux C代码实现进程是否存在
时间: 2024-08-10 10:01:24 浏览: 39
Linux下的C语言确实可以用于创建、管理以及控制进程。Linux操作系统提供了一系列的系统调用(System Call),通过这些系统调用,程序可以在用户空间和内核空间之间进行交互,进而实现对进程的操作。
以下是几个常用的C语言实现进程操作的例子:
### 1. 创建新进程
在Linux中,可以通过`fork()`函数创建一个新的进程。这个函数会生成当前进程的一个副本,新的进程被称为当前进程的子进程。`fork()`函数调用成功返回0给子进程,返回其子进程的PID给父进程。
```c
#include <unistd.h>
#include <stdio.h>
int main() {
pid_t child_pid = fork();
if (child_pid == -1) { // Error in creating the child process.
perror("Fork error");
return 1;
}
else if (child_pid > 0) { // Parent process
printf("Parent: Process ID of child is %d\n", child_pid);
}
else { // Child process
printf("Child: I am a new process with PID %d!\n", getpid());
}
return 0;
}
```
### 2. 进程等待结束
当一个父进程想要等待子进程完成并退出后再继续运行时,可以使用`wait()`函数或者`waitpid()`函数。
```c
#include <unistd.h>
#include <stdio.h>
int main() {
pid_t child_pid = fork();
if (child_pid == -1) {
perror("Fork error");
return 1;
} else if (child_pid > 0) {
// 父进程等待子进程完成
int status;
wait(&status);
printf("Parent: Child process has finished, exit status is %d.\n", WEXITSTATUS(status));
} else {
// 子进程
printf("Child process exiting...\n");
}
return 0;
}
```
### 3. 终止进程
有时我们需要强制终止一个正在运行的进程。这可以通过向进程发送特定信号来实现,例如SIGTERM或SIGKILL。
```c
#include <signal.h>
#include <stdio.h>
void handler(int sig) {
switch (sig) {
case SIGTERM:
printf("Received SIGTERM signal. Exiting...\n");
break;
default:
printf("Received an unknown signal: %d\n", sig);
break;
}
}
int main() {
signal(SIGTERM, handler);
while (true); // Keep running to receive signals.
return 0;
}
```
以上就是使用C语言在Linux下实现基本进程操作的一些例子。Linux的系统调用提供了强大的功能来管理和控制进程,使得开发者能够高效地进行并发处理和其他高级操作。
阅读全文
相关推荐
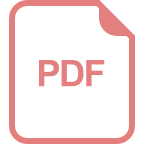
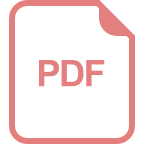



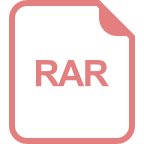
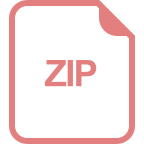
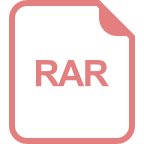
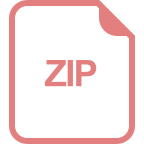
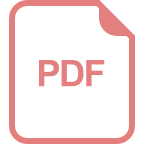
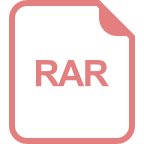
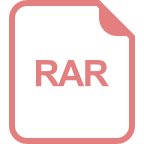
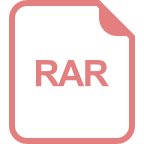
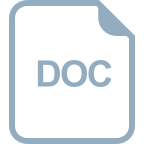
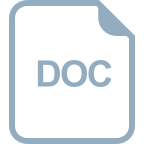
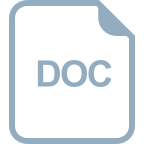
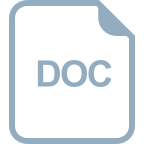