设计一个酒店房间预订的管理员界面jsp
时间: 2023-12-04 14:04:45 浏览: 99
好的,以下是我为您设计的酒店房间预订的管理员界面JSP页面:
1. 登录页面:管理员需要输入用户名和密码才能进入系统。
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>管理员登录</title>
</head>
<body>
<form action="login.jsp" method="post">
<label for="username">用户名:</label>
<input type="text" id="username" name="username"><br>
<label for="password">密码:</label>
<input type="password" id="password" name="password"><br>
<input type="submit" value="登录">
</form>
</body>
</html>
```
2. 首页:管理员登录后,会看到一个简洁的主页,上面显示了系统的一些基本信息,比如房间总数、已预订房间数、剩余房间数等等。管理员可以在这个页面上进行房间管理和预订管理。
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>管理员首页</title>
</head>
<body>
<h1>欢迎您,管理员!</h1>
<ul>
<li>房间总数:${roomCount}</li>
<li>已预订房间数:${bookedRoomCount}</li>
<li>剩余房间数:${availableRoomCount}</li>
</ul>
<ul>
<li><a href="roomList.jsp">房间管理</a></li>
<li><a href="bookingList.jsp">预订管理</a></li>
<li><a href="dataStatistics.jsp">数据统计</a></li>
<li><a href="settings.jsp">系统设置</a></li>
</ul>
</body>
</html>
```
3. 房间管理页面:管理员可以在这个页面上查看所有房间的基本信息,包括房间号、房型、价格、状态等等。管理员可以添加新房间、编辑已有房间信息、删除不需要的房间等等。
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>房间管理</title>
</head>
<body>
<h1>房间管理</h1>
<table>
<tr>
<th>房间号</th>
<th>房型</th>
<th>价格</th>
<th>状态</th>
<th>操作</th>
</tr>
<c:forEach items="${roomList}" var="room">
<tr>
<td>${room.roomNumber}</td>
<td>${room.roomType}</td>
<td>${room.price}</td>
<td>${room.status}</td>
<td>
<a href="editRoom.jsp?roomId=${room.id}">编辑</a>
<a href="deleteRoom.jsp?roomId=${room.id}">删除</a>
</td>
</tr>
</c:forEach>
</table>
<a href="addRoom.jsp">添加新房间</a>
</body>
</html>
```
4. 预订管理页面:管理员可以在这个页面上查看所有预订的房间信息,包括客人姓名、入住时间、离店时间、房间号、价格等等。管理员可以查看未确认的预订、已确认的预订、已取消的预订等等。管理员可以根据需要进行确认、取消、编辑预订信息等等。
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>预订管理</title>
</head>
<body>
<h1>预订管理</h1>
<table>
<tr>
<th>客人姓名</th>
<th>入住时间</th>
<th>离店时间</th>
<th>房间号</th>
<th>价格</th>
<th>状态</th>
<th>操作</th>
</tr>
<c:forEach items="${bookingList}" var="booking">
<tr>
<td>${booking.guestName}</td>
<td>${booking.checkInDate}</td>
<td>${booking.checkOutDate}</td>
<td>${booking.roomNumber}</td>
<td>${booking.price}</td>
<td>${booking.status}</td>
<td>
<a href="confirmBooking.jsp?bookingId=${booking.id}">确认</a>
<a href="cancelBooking.jsp?bookingId=${booking.id}">取消</a>
<a href="editBooking.jsp?bookingId=${booking.id}">编辑</a>
</td>
</tr>
</c:forEach>
</table>
</body>
</html>
```
5. 数据统计页面:管理员可以在这个页面上查看各种数据的统计信息,比如每月的房间入住率、每月的收入情况等等。管理员可以根据需要进行各种数据的筛选和分析。
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>数据统计</title>
</head>
<body>
<h1>数据统计</h1>
<form action="dataStatistics.jsp" method="get">
<label for="year">年份:</label>
<select id="year" name="year">
<c:forEach var="i" begin="2020" end="2030">
<option value="${i}" ${i eq year ? 'selected' : ''}>${i}</option>
</c:forEach>
</select>
<input type="submit" value="查询">
</form>
<p>房间入住率:${occupancyRate}%</p>
<p>当月收入:${income}元</p>
</body>
</html>
```
6. 设置页面:管理员可以在这个页面上进行系统设置,比如设置管理员账号、修改密码、设置房型和价格等等。
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>系统设置</title>
</head>
<body>
<h1>系统设置</h1>
<form action="updatePassword.jsp" method="post">
<label for="oldPassword">旧密码:</label>
<input type="password" id="oldPassword" name="oldPassword"><br>
<label for="newPassword">新密码:</label>
<input type="password" id="newPassword" name="newPassword"><br>
<label for="confirmPassword">确认密码:</label>
<input type="password" id="confirmPassword" name="confirmPassword"><br>
<input type="submit" value="修改密码">
</form>
<form action="updateRoomType.jsp" method="post">
<label for="roomType">房型:</label>
<input type="text" id="roomType" name="roomType"><br>
<label for="price">价格:</label>
<input type="text" id="price" name="price"><br>
<input type="submit" value="添加新房型">
</form>
</body>
</html>
```
希望这个设计能够满足您的需求。请注意,这只是一个简单的示例,实际的代码可能需要更加复杂和完善。
阅读全文
相关推荐
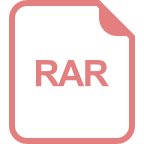
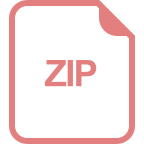
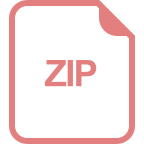
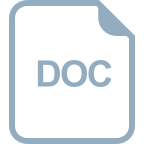
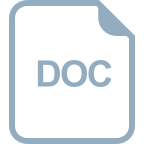
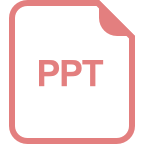
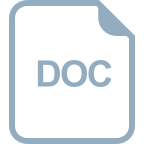
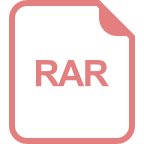
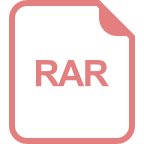
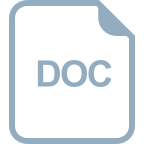
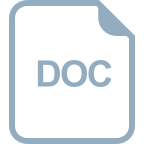
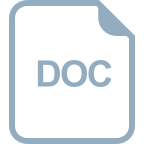
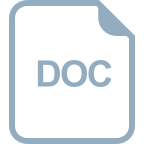
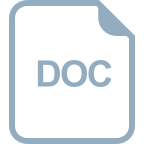
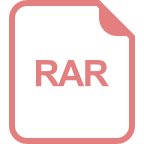
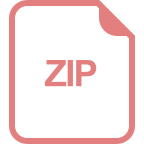