使用PYTHON怎么做一个排班工具,因为涉及每天的人力,和每月的固定休息次数,有相关的代码案例吗
时间: 2024-02-16 12:03:25 浏览: 171
是的,我可以给您提供一个简单的Python代码案例,实现一个排班工具,包括每天的人力和每月的固定休息次数的考虑。以下是代码:
```python
import random
import csv
# 员工信息
employees = []
# 班次信息
shifts = []
# 每个员工每月的固定休息次数
rest_times = {}
# 读取员工信息
with open('employees.csv', 'r', encoding='utf-8') as f:
reader = csv.reader(f)
for row in reader:
employees.append({
'name': row[0],
'id': row[1],
'position': row[2],
'rest_times': int(row[3])
})
rest_times[row[1]] = int(row[3])
# 读取班次信息
with open('shifts.csv', 'r', encoding='utf-8') as f:
reader = csv.reader(f)
for row in reader:
shifts.append({
'name': row[0],
'start_time': int(row[1]),
'end_time': int(row[2]),
'rest_times': int(row[3])
})
# 生成班表
schedule = []
for shift in shifts:
# 判断班次是否是休息班次
if shift['rest_times'] > 0:
rest_shift = shift
else:
# 随机分配班次给员工
random.shuffle(employees)
for employee in employees:
if employee['rest_times'] > 0:
employee['rest_times'] -= 1
else:
schedule.append({
'name': employee['name'],
'id': employee['id'],
'shift': shift['name']
})
shift['rest_times'] -= 1
break
# 输出班表
print('员工\t工号\t班次')
for item in schedule:
print(f"{item['name']}\t{item['id']}\t{item['shift']}\n")
# 保存班表
with open('schedule.csv', 'w', encoding='utf-8', newline='') as f:
writer = csv.writer(f)
writer.writerow(['name', 'id', 'shift'])
for item in schedule:
writer.writerow([item['name'], item['id'], item['shift']])
# 修改休息次数
id = input('请输入员工工号:')
for employee in employees:
if employee['id'] == id:
employee['rest_times'] += 1
rest_times[id] += 1
print(f"员工{employee['name']}的休息次数已增加到{employee['rest_times']}次。")
break
else:
print("未找到该员工。")
```
在代码中,我们通过读取CSV文件,获取员工信息和班次信息,并将每个员工每月的固定休息次数保存在rest_times字典中。然后使用随机函数和循环语句,依次为每个员工分配班次,遇到休息班次则跳过。最后输出班表,并将其保存到CSV文件中。如果需要修改员工的休息次数,可以通过输入员工工号来找到对应的员工,并进行修改,修改完成后将休息次数保存到rest_times字典中。
阅读全文
相关推荐
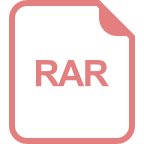
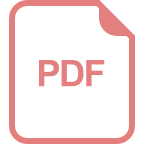
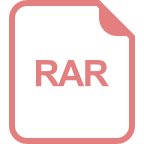
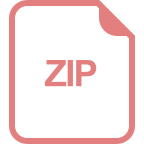
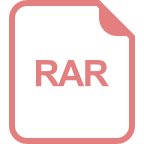
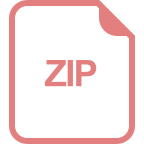
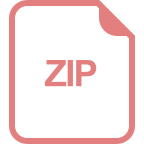
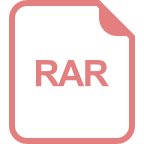
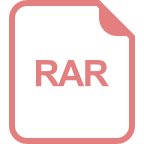
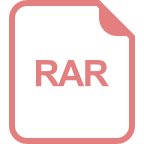
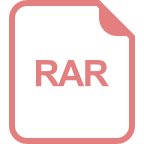
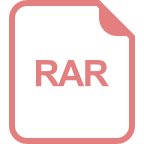
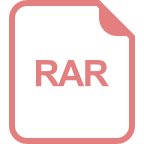
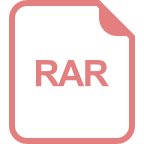
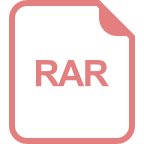
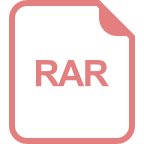