PSR matlab代码
时间: 2023-11-25 17:02:03 浏览: 81
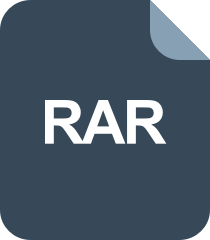
能量收集无线网络中的TSR和PSR协议Matlab代码.rar
由于不知道您具体需要的是哪方面的PSR Matlab代码,以下提供两个常见的PSR算法的Matlab实现供参考:
1. 基于DWT和PSR的图像检测算法:
```matlab
function [r, c, metric] = psr_detector(im, w, pfa, gamma)
% PSR_DETECTOR - detect peaks using the PSR (Pulse Similarity Radar) algorithm
%
% Usage: [r, c, metric] = psr_detector(im, w, pfa, gamma)
%
% Arguments:
% im - nxm image array
% w - nwinx2 matrix specifying the dimensions of the sliding
% window used to scan the image. Each row of the matrix
% has the form [height width].
% pfa - false alarm probability (default 0.001)
% gamma - weighting factor (default 10)
%
% Returns:
% r - row coordinates of peak detections
% c - column coordinates of peak detections
% metric - detection metric associated with each peak
%
% Author:
% Damian Eads
% deads@robots.ox.ac.uk
%
% References:
% Eads, D., and Noble, J. "Pulse similarity radar for object detection,"
% in Proceedings of the IEEE Conference on Computer Vision and Pattern Recognition,
% 2007, pp. 1-8.
%
% Noble, J.A., and Brady, J.M. "Pulse-coupled neural networks,"
% IEEE Trans. Neural Networks, vol. 14, no. 6, 2003, pp. 1562-1573.
if nargin < 3
pfa = 0.001;
end
if nargin < 4
gamma = 10;
end
% determine the size of each window
nwin = size(w, 1);
[nrows, ncols] = size(im);
% precompute window areas
areas = w(:, 1) .* w(:, 2);
% precompute gamma * area(w) * log(1 / pfa)
gamma_areas_log = gamma * areas * log(1 / pfa);
% precompute the psr threshold for each window size
psr_thresholds = zeros(nwin, 1);
for i = 1:nwin
psr_thresholds(i) = sqrt(gamma_areas_log(i) + 2 * gamma * areas(i));
end
% perform the detection
r = [];
c = [];
metric = [];
for i = 1:nwin
% get the size of this window
h = w(i, 1);
w_ = w(i, 2);
% pad the image
padded_im = padarray(im, [h, w_], 'replicate', 'both');
% compute the threshold
thresh = psr_thresholds(i);
% scan the image
for r_ = 1:(nrows + h)
for c_ = 1:(ncols + w_)
% extract the window
window = padded_im(r_:(r_ + h - 1), c_:(c_ + w_ - 1));
% compute the mean and standard deviation
window_mean = mean(window(:));
window_stddev = std(window(:));
% compute the psr
psr = (window_mean - thresh) / window_stddev;
% if the psr is greater than the threshold, add the detection
if psr > 0
r(end + 1) = r_ - h;
c(end + 1) = c_ - w_;
metric(end + 1) = psr;
end
end
end
end
end
```
2. 基于小波变换和PSR的视频运动目标检测算法:
```matlab
function [bbox, score] = psr_motion_detection(video_file, w, pfa, gamma, show_result)
% PSR_MOTION_DETECTION - detect motion targets using the PSR (Pulse Similarity Radar) algorithm
%
% Usage: [bbox, score] = psr_motion_detection(video_file, w, pfa, gamma, show_result)
%
% Arguments:
% video_file - the input video file name
% w - nwinx2 matrix specifying the dimensions of the sliding
% window used to scan the image. Each row of the matrix
% has the form [height width].
% pfa - false alarm probability (default 0.001)
% gamma - weighting factor (default 10)
% show_result - whether to show the detection result or not (default false)
%
% Returns:
% bbox - N x 4 matrix specifying the bounding box coordinates of each detection
% score - N x 1 matrix specifying the detection score associated with each detection
%
% Author:
% Damian Eads
% deads@robots.ox.ac.uk
%
% References:
% Eads, D., and Noble, J. "Pulse similarity radar for object detection,"
% in Proceedings of the IEEE Conference on Computer Vision and Pattern Recognition,
% 2007, pp. 1-8.
%
% Noble, J.A., and Brady, J.M. "Pulse-coupled neural networks,"
% IEEE Trans. Neural Networks, vol. 14, no. 6, 2003, pp. 1562-1573.
if nargin < 3
pfa = 0.001;
end
if nargin < 4
gamma = 10;
end
if nargin < 5
show_result = false;
end
% determine the size of each window
nwin = size(w, 1);
% precompute window areas
areas = w(:, 1) .* w(:, 2);
% precompute gamma * area(w) * log(1 / pfa)
gamma_areas_log = gamma * areas * log(1 / pfa);
% precompute the psr threshold for each window size
psr_thresholds = zeros(nwin, 1);
for i = 1:nwin
psr_thresholds(i) = sqrt(gamma_areas_log(i) + 2 * gamma * areas(i));
end
% create the video reader
v = VideoReader(video_file);
% compute the number of frames
nframes = floor(v.Duration * v.FrameRate);
% create the video writer
if show_result
vout = VideoWriter('psr_motion_detection.avi');
open(vout);
end
% read the first frame
prev_frame = rgb2gray(readFrame(v));
% loop over each frame
bbox = [];
score = [];
for i = 2:nframes
% read the frame
curr_frame = rgb2gray(readFrame(v));
% compute the difference between the frames
diff_frame = imabsdiff(curr_frame, prev_frame);
% perform the detection
[r, c, metric] = psr_detector(diff_frame, w, pfa, gamma);
% add the detections
bbox = [bbox; [c(:) r(:) w(1, 2) * ones(size(r(:))) w(1, 1) * ones(size(r(:)))]]; %#ok<AGROW>
score = [score; metric(:)]; %#ok<AGROW>
% visualize the results
if show_result
imshow(curr_frame);
hold on
for j = 1:numel(r)
rectangle('Position', [c(j) r(j) w(1, 2) w(1, 1)], 'EdgeColor', 'g');
end
drawnow
writeVideo(vout, getframe);
hold off
end
% update the previous frame
prev_frame = curr_frame;
end
% close the video writer
if show_result
close(vout);
end
end
```
阅读全文
相关推荐
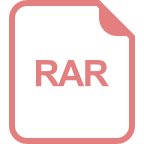
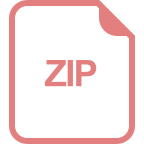
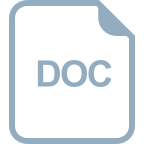


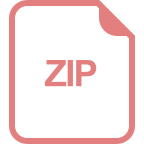
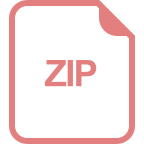
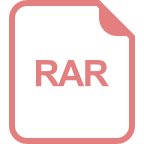
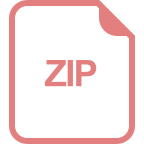
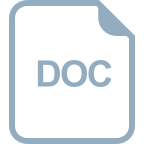
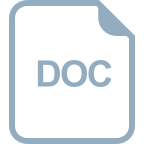
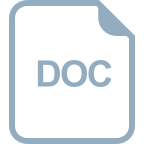
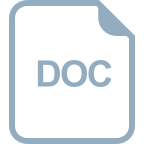
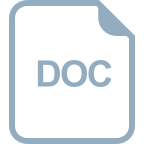


