agent与app 实现websocket protobuf通信代码
时间: 2024-02-25 21:52:56 浏览: 22
以下是示例代码,演示如何在agent和app之间实现websocket protobuf通信。其中使用的是Python语言和protobuf库。
#### Agent端代码
```python
import websocket
import protobuf_message_pb2
# 定义websocket连接地址
websocket_url = "ws://localhost:8000"
# 建立websocket连接
ws = websocket.create_connection(websocket_url)
# 创建protobuf消息对象
message = protobuf_message_pb2.Message()
message.msg_type = protobuf_message_pb2.MessageType.TEXT
message.content = "Hello, App!"
# 将消息序列化为二进制数据
data = message.SerializeToString()
# 发送消息
ws.send(data)
# 接收消息
result = ws.recv()
# 将接收到的数据反序列化为protobuf消息对象
received_message = protobuf_message_pb2.Message()
received_message.ParseFromString(result)
# 处理接收到的消息
if received_message.msg_type == protobuf_message_pb2.MessageType.TEXT:
print("Received message from App:", received_message.content)
# 关闭websocket连接
ws.close()
```
#### App端代码
```python
import websocket
import protobuf_message_pb2
# 定义websocket连接地址
websocket_url = "ws://localhost:8000"
# 建立websocket连接
ws = websocket.create_connection(websocket_url)
# 接收消息
result = ws.recv()
# 将接收到的数据反序列化为protobuf消息对象
received_message = protobuf_message_pb2.Message()
received_message.ParseFromString(result)
# 处理接收到的消息
if received_message.msg_type == protobuf_message_pb2.MessageType.TEXT:
print("Received message from Agent:", received_message.content)
# 创建protobuf消息对象
message = protobuf_message_pb2.Message()
message.msg_type = protobuf_message_pb2.MessageType.TEXT
message.content = "Hello, Agent!"
# 将消息序列化为二进制数据
data = message.SerializeToString()
# 发送消息
ws.send(data)
# 关闭websocket连接
ws.close()
```
以上是示例代码,仅供参考。实际场景中需要根据具体需求进行修改和完善。
相关推荐
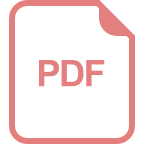
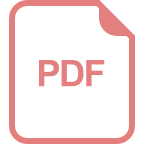














