linux c++ 压缩文件夹
时间: 2023-11-10 15:51:13 浏览: 283
您可以使用`tar`命令来压缩文件夹。下面是一个示例命令:
```
tar -czvf compressed.tar.gz folder_to_compress
```
其中,`-c`表示创建压缩文件,`-z`表示使用gzip压缩算法,`-v`表示显示压缩过程的详细信息,`-f`表示指定要创建的压缩文件。您需要将`folder_to_compress`替换为要压缩的文件夹的路径。
此命令将在当前目录下创建一个名为`compressed.tar.gz`的压缩文件,并将指定的文件夹压缩到其中。
您还可以使用其他压缩算法,如bzip2(使用`-j`参数)或xz(使用`-J`参数),具体取决于您的需求。
相关问题
linux c++ 编程压缩文件夹为tar.gz
在Linux下,可以使用tar命令来压缩文件夹为tar.gz格式。以下是C++代码示例:
```c++
#include <iostream>
#include <cstdlib>
#include <cstdio>
using namespace std;
int main() {
string folderName = "/path/to/folder"; // 要压缩的文件夹路径
string tarName = "compressed.tar.gz"; // 压缩后的文件名
string cmd = "tar -zcvf " + tarName + " " + folderName; // 组装命令
int ret = system(cmd.c_str()); // 执行命令
if (ret == -1) {
cout << "Failed to compress folder!" << endl;
return -1;
}
cout << "Folder compressed successfully!" << endl;
return 0;
}
```
该代码首先指定要压缩的文件夹路径和压缩后的文件名,然后使用system函数执行tar命令。如果执行成功,返回值为0,否则返回-1。
需要注意的是,如果要压缩的文件夹中包含大量文件或文件夹,可能会花费一定时间来完成压缩。
linux c+压缩解压文件夹
要在 Linux C++ 中进行文件夹的压缩和解压缩,可以使用 libarchive 库。该库提供了一组函数,可以在 C++ 中使用。
以下是一个简单的示例代码,展示了如何使用 libarchive 库进行文件夹的压缩和解压缩:
```c++
#include <iostream>
#include <archive.h>
#include <archive_entry.h>
// 压缩文件夹
void compressFolder(const std::string& srcFolder, const std::string& dstFile)
{
struct archive* a = archive_write_new();
archive_write_add_filter_gzip(a);
archive_write_set_format_pax_restricted(a);
archive_write_open_filename(a, dstFile.c_str());
struct archive* b = archive_read_new();
archive_read_support_format_tar(b);
archive_read_support_filter_gzip(b);
archive_read_open_filename(b, srcFolder.c_str(), 10240);
struct archive_entry* entry;
while (archive_read_next_header(b, &entry) == ARCHIVE_OK) {
archive_write_header(a, entry);
char buff[1024];
size_t len;
while ((len = archive_read_data(b, buff, sizeof(buff))) > 0) {
archive_write_data(a, buff, len);
}
}
archive_read_close(b);
archive_read_free(b);
archive_write_close(a);
archive_write_free(a);
}
// 解压文件夹
void uncompressFolder(const std::string& srcFile, const std::string& dstFolder)
{
struct archive* a = archive_read_new();
archive_read_support_format_all(a);
archive_read_support_filter_all(a);
struct archive_entry* entry;
int r = archive_read_open_filename(a, srcFile.c_str(), 10240);
if (r != ARCHIVE_OK) {
std::cerr << "archive_read_open_filename failed" << std::endl;
return;
}
while (archive_read_next_header(a, &entry) == ARCHIVE_OK) {
std::string path = dstFolder + "/" + archive_entry_pathname(entry);
if (archive_entry_filetype(entry) == AE_IFDIR) {
mkdir(path.c_str(), 0755);
} else {
std::ofstream ofs(path, std::ios::binary);
if (!ofs) {
std::cerr << "open file failed: " << path << std::endl;
return;
}
char buff[1024];
size_t len;
while ((len = archive_read_data(a, buff, sizeof(buff))) > 0) {
ofs.write(buff, len);
}
}
}
archive_read_close(a);
archive_read_free(a);
}
int main()
{
const std::string srcFolder = "/path/to/folder";
const std::string dstFile = "test.tar.gz";
// 压缩文件夹
compressFolder(srcFolder, dstFile);
// 解压文件夹
uncompressFolder(dstFile, "/path/to/output/folder");
return 0;
}
```
在上面的代码中,compressFolder 函数用于压缩文件夹,uncompressFolder 函数用于解压文件夹。这两个函数都包含了对 libarchive 库的初始化、数据处理和结束操作。请注意,这里使用的是 tar 和 gzip 格式,因此压缩文件的扩展名为 .tar.gz。
阅读全文
相关推荐
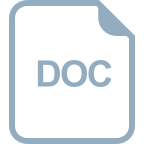
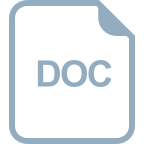
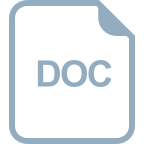
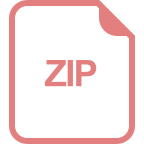
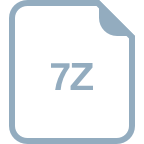
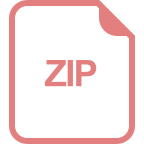
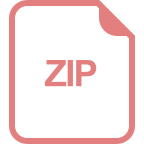
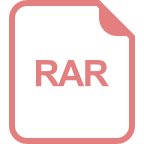
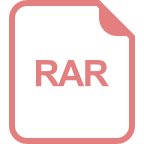
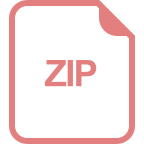
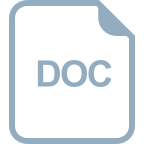
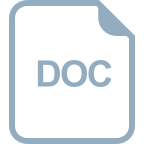
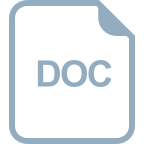
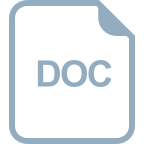
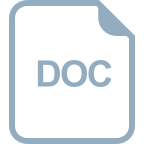
