微信小程序画布 canvas 内容拖拽
时间: 2023-05-13 10:00:59 浏览: 531
微信小程序提供了canvas组件来绘制图形和动画。在canvas组件中,我们可以实现内容拖拽的效果。
实现内容拖拽需要用到canvas的两个API:touchstart 和 touchmove。当用户手指按下屏幕时,我们可以使用touchstart事件来记录手指的初始坐标,作为拖拽的起始点。然后在touchmove事件中,通过计算手指在屏幕上移动的距离,可以实现内容的拖拽。将计算出的距离加上内容的初始坐标,就可以获得内容在拖拽后的新坐标。
需要注意的是,canvas中的内容不能直接被点击或拖拽。我们需要自己定义一个支持拖拽的区域,如一个矩形,然后在拖拽时判断手指是否在这个区域内。如果在区域内,则执行拖拽效果,否则忽略。
除了实现拖拽效果,我们还需要注意canvas中的性能优化。因为canvas可能会绘制复杂的图形和动画,我们需要避免频繁调用canvas相关的API,以减少开销。同时,需要对canvas内容的大小进行限制,以避免资源的浪费和卡顿的情况发生。
总之,在微信小程序中实现内容拖拽,需要使用canvas的touchstart和touchmove事件,并注意canvas的性能优化。通过这些步骤,我们可以实现一个交互友好的小程序画布。
相关问题
微信小程序拖动按帧裁剪视频
微信小程序拖动按帧裁剪视频的具体实现方法如下:
1. 在 wxml 文件中添加 video 组件、两个按钮和一个 canvas 组件,用于渲染拖动进度条。
```
<video id="myVideo" src="{{src}}" controls></video>
<button type="primary" bindtap="startCutting">开始裁剪</button>
<button type="primary" bindtap="endCutting">结束裁剪</button>
<canvas id="progressCanvas"></canvas>
```
2. 在 js 文件中监听视频播放事件和按钮的点击事件,并在开始裁剪时记录当前时间,结束裁剪时计算裁剪后的时长。
```
data: {
src: '', // 视频地址
ctx: null, // video 组件的上下文
duration: 0, // 视频总时长
startTime: 0, // 裁剪开始时间
endTime: 0, // 裁剪结束时间
progressWidth: 0, // 进度条宽度
progressHeight: 0, // 进度条高度
progressLeft: 0 // 进度条左边距
},
onLoad() {
this.ctx = wx.createVideoContext('myVideo', this);
},
onReady() {
this.ctx.pause(); // 加载后暂停播放
this.ctx.duration((res) => {
this.duration = res.duration;
});
},
onPlay() {
this.drawProgress(); // 每次播放时绘制进度条
},
startCutting() {
this.ctx.play(); // 开始播放
this.startTime = this.ctx.currentTime; // 记录开始时间
},
endCutting() {
this.ctx.pause(); // 暂停播放
this.endTime = this.ctx.currentTime; // 记录结束时间
const cutDuration = this.endTime - this.startTime; // 计算裁剪后的时长
console.log(`裁剪后的时长为 ${cutDuration}`);
},
drawProgress() {
const query = wx.createSelectorQuery();
query.select('#progressCanvas').boundingClientRect((rect) => {
const canvasWidth = rect.width;
const canvasHeight = rect.height;
const progressWidth = canvasWidth * this.ctx.currentTime / this.duration; // 计算进度条宽度
const progressHeight = canvasHeight;
const progressLeft = 0;
const ctx = wx.createCanvasContext('progressCanvas', this);
ctx.clearRect(0, 0, canvasWidth, canvasHeight); // 清空画布
ctx.setFillStyle('#f00');
ctx.fillRect(progressLeft, 0, progressWidth, progressHeight);
ctx.draw();
this.progressWidth = progressWidth;
this.progressHeight = progressHeight;
this.progressLeft = progressLeft;
}).exec();
}
```
3. 在 wxss 文件中设置 canvas 的样式。
```
canvas {
width: 100%;
height: 20rpx;
background-color: #eee;
}
```
4. 在 canvas 组件上绑定 touchstart、touchmove 和 touchend 事件,实现拖动进度条的功能。
```
onProgressTouchStart(e) {
const touch = e.touches[0];
this.progressTouchStartX = touch.clientX;
this.progressTouchStartLeft = this.progressLeft;
},
onProgressTouchMove(e) {
const touch = e.touches[0];
const distanceX = touch.clientX - this.progressTouchStartX;
const progressLeft = this.progressTouchStartLeft + distanceX;
if (progressLeft < 0) {
this.progressLeft = 0;
} else if (progressLeft > this.progressWidth) {
this.progressLeft = this.progressWidth;
} else {
this.progressLeft = progressLeft;
}
this.updateProgress();
},
onProgressTouchEnd(e) {
this.ctx.seek(this.duration * this.progressLeft / this.progressWidth); // 根据进度条位置跳转视频播放进度
},
updateProgress() {
const ctx = wx.createCanvasContext('progressCanvas', this);
ctx.clearRect(0, 0, this.progressWidth, this.progressHeight); // 清空画布
ctx.setFillStyle('#f00');
ctx.fillRect(this.progressLeft, 0, this.progressWidth - this.progressLeft, this.progressHeight);
ctx.draw(true);
}
```
注意:拖动进度条时需要记录开始触摸时的位置和进度条的左边距,然后根据移动距离计算进度条的位置,最后根据进度条位置跳转视频播放进度。由于视频播放的精度可能不够,裁剪时可能会有一些误差。如果需要更精确的裁剪,可以使用一些第三方的视频处理库。
阅读全文
相关推荐
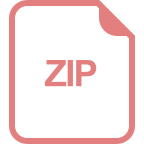
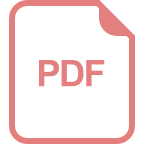
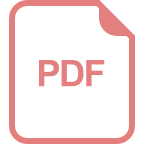
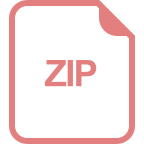
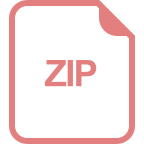
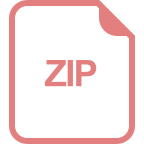
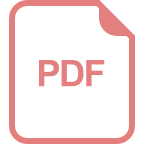
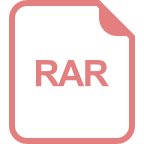
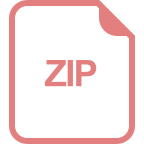
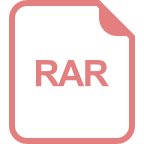
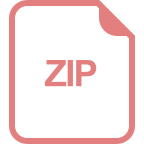
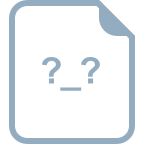
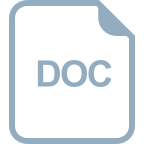
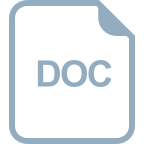
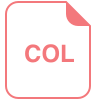
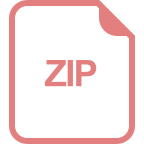
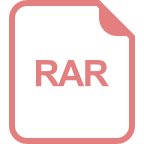