Tcl读取文件和向文件中写入数据的相关命令和讲解以及代码举例说明
时间: 2024-05-04 20:20:45 浏览: 18
Tcl 读取文件和向文件中写入数据的相关命令包括:
1. 读取文件的命令:
- `open` 打开文件
- `read` 读取文件内容
- `gets` 逐行读取文件内容
- `close` 关闭文件
2. 向文件中写入数据的命令:
- `open` 打开文件
- `puts` 向文件中写入数据
- `close` 关闭文件
下面我们分别对这些命令进行讲解,并且给出相应的代码示例:
读取文件的命令:
1. `open` 打开文件:
使用 `open` 命令可以打开一个文件。语法如下:
```tcl
set fileHandler [open fileName mode]
```
其中,`fileName` 是文件名,`mode` 是文件打开模式。文件打开模式包括:
- `r`:只读模式
- `w`:只写模式
- `a`:追加模式
示例代码:
```tcl
set fileHandler [open "test.txt" r]
```
2. `read` 读取文件内容:
使用 `read` 命令可以读取文件的全部内容。语法如下:
```tcl
set fileContent [read fileHandler]
```
其中,`fileHandler` 是文件句柄,`fileContent` 是文件内容。
示例代码:
```tcl
set fileHandler [open "test.txt" r]
set fileContent [read $fileHandler]
```
3. `gets` 逐行读取文件内容:
使用 `gets` 命令可以逐行读取文件内容。语法如下:
```tcl
while {[gets fileHandler line] != -1} {
# 处理每一行的数据
}
```
其中,`fileHandler` 是文件句柄,`line` 是每一行的内容。
示例代码:
```tcl
set fileHandler [open "test.txt" r]
while {[gets $fileHandler line] != -1} {
puts $line
}
```
4. `close` 关闭文件:
使用 `close` 命令可以关闭文件。语法如下:
```tcl
close fileHandler
```
其中,`fileHandler` 是文件句柄。
示例代码:
```tcl
set fileHandler [open "test.txt" r]
set fileContent [read $fileHandler]
close $fileHandler
```
向文件中写入数据的命令:
1. `open` 打开文件:
同样使用 `open` 命令可以打开一个文件。语法如下:
```tcl
set fileHandler [open fileName mode]
```
其中,`fileName` 是文件名,`mode` 是文件打开模式。文件打开模式包括:
- `r`:只读模式
- `w`:只写模式
- `a`:追加模式
示例代码:
```tcl
set fileHandler [open "test.txt" w]
```
2. `puts` 向文件中写入数据:
使用 `puts` 命令可以向文件中写入数据。语法如下:
```tcl
puts fileHandler data
```
其中,`fileHandler` 是文件句柄,`data` 是要写入的数据。
示例代码:
```tcl
set fileHandler [open "test.txt" w]
puts $fileHandler "Hello, World!"
```
3. `close` 关闭文件:
同样使用 `close` 命令可以关闭文件。语法如下:
```tcl
close fileHandler
```
其中,`fileHandler` 是文件句柄。
示例代码:
```tcl
set fileHandler [open "test.txt" w]
puts $fileHandler "Hello, World!"
close $fileHandler
```
相关推荐
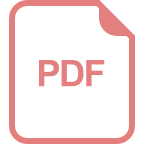
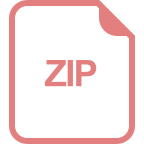














