pytorch 3D prewitt
时间: 2023-06-21 18:10:28 浏览: 98
PyTorch does not have a built-in implementation of 3D Prewitt filter. However, you can implement it using PyTorch's built-in 3D convolution operation (`torch.nn.Conv3d`) and manually defining the Prewitt kernel. Here's an example code snippet:
```
import torch
import torch.nn as nn
# Define the 3D Prewitt kernel
prewitt_kernel_x = torch.Tensor([[[[-1, 0, 1], [-1, 0, 1], [-1, 0, 1]],
[[-1, 0, 1], [-1, 0, 1], [-1, 0, 1]],
[[-1, 0, 1], [-1, 0, 1], [-1, 0, 1]]]])
prewitt_kernel_y = torch.Tensor([[[[-1, -1, -1], [0, 0, 0], [1, 1, 1]],
[[-1, -1, -1], [0, 0, 0], [1, 1, 1]],
[[-1, -1, -1], [0, 0, 0], [1, 1, 1]]]])
prewitt_kernel_z = torch.Tensor([[[[-1, -1, -1], [-1, -1, -1], [-1, -1, -1]],
[[0, 0, 0], [0, 0, 0], [0, 0, 0]],
[[1, 1, 1], [1, 1, 1], [1, 1, 1]]]])
# Define the 3D Prewitt convolution layer
prewitt_conv_x = nn.Conv3d(1, 1, kernel_size=3, stride=1, padding=1, bias=False)
prewitt_conv_y = nn.Conv3d(1, 1, kernel_size=3, stride=1, padding=1, bias=False)
prewitt_conv_z = nn.Conv3d(1, 1, kernel_size=3, stride=1, padding=1, bias=False)
# Set the prewitt kernel to the convolution layer
prewitt_conv_x.weight = nn.Parameter(prewitt_kernel_x)
prewitt_conv_y.weight = nn.Parameter(prewitt_kernel_y)
prewitt_conv_z.weight = nn.Parameter(prewitt_kernel_z)
# Apply the 3D Prewitt filter to a 3D tensor
input_tensor = torch.randn(1, 1, 10, 10, 10) # 1 channel, 10x10x10 volume
output_tensor_x = prewitt_conv_x(input_tensor)
output_tensor_y = prewitt_conv_y(input_tensor)
output_tensor_z = prewitt_conv_z(input_tensor)
```
In the code above, we manually define the 3D Prewitt kernel for the x, y and z directions. We then define a 3D convolution layer for each direction using PyTorch's `nn.Conv3d` module. We set the prewitt kernel to the convolution layer and apply the 3D Prewitt filter to a 3D tensor.
阅读全文
相关推荐
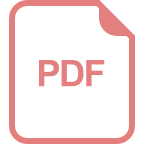
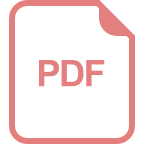
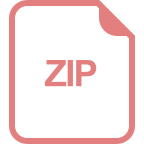
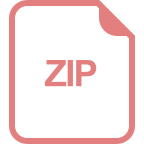
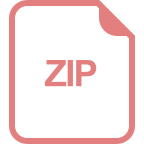
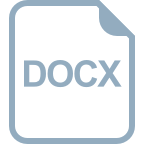
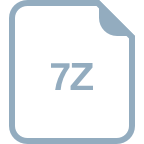
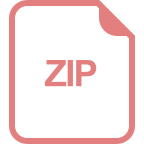
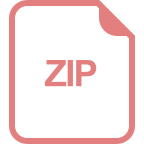
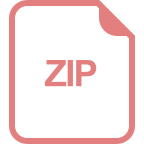
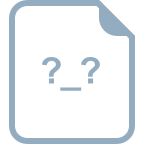
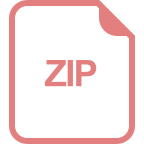
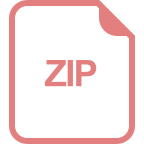
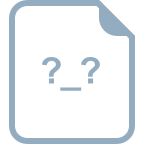
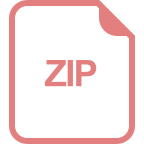
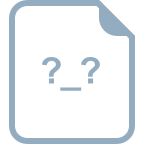
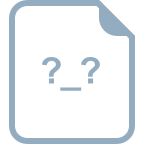