java安卓ble蓝牙连接订阅功能的实现以及使用的完整代码
时间: 2024-02-17 22:01:34 浏览: 23
好的,这是一个基于Java的Android BLE蓝牙连接订阅功能的示例代码:
```
public class MainActivity extends AppCompatActivity {
private BluetoothManager bluetoothManager;
private BluetoothAdapter bluetoothAdapter;
private BluetoothGatt bluetoothGatt;
private BluetoothGattCallback bluetoothGattCallback = new BluetoothGattCallback() {
@Override
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) {
super.onConnectionStateChange(gatt, status, newState);
if(newState == BluetoothProfile.STATE_CONNECTED) {
Log.d("BLE", "Connected to GATT server.");
gatt.discoverServices();
}
else if(newState == BluetoothProfile.STATE_DISCONNECTED) {
Log.d("BLE", "Disconnected from GATT server.");
}
}
@Override
public void onServicesDiscovered(BluetoothGatt gatt, int status) {
super.onServicesDiscovered(gatt, status);
if(status == BluetoothGatt.GATT_SUCCESS) {
BluetoothGattService service = gatt.getService(UUID.fromString("service_uuid"));
BluetoothGattCharacteristic characteristic = service.getCharacteristic(UUID.fromString("characteristic_uuid"));
boolean result = gatt.setCharacteristicNotification(characteristic, true);
if(result) {
Log.d("BLE", "Characteristic notification set successfully.");
}
else {
Log.d("BLE", "Characteristic notification set failed.");
}
BluetoothGattDescriptor descriptor = characteristic.getDescriptor(UUID.fromString("descriptor_uuid"));
descriptor.setValue(BluetoothGattDescriptor.ENABLE_NOTIFICATION_VALUE);
gatt.writeDescriptor(descriptor);
}
}
@Override
public void onCharacteristicChanged(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic) {
super.onCharacteristicChanged(gatt, characteristic);
byte[] data = characteristic.getValue();
String message = new String(data);
Log.d("BLE", "Received message: " + message);
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
bluetoothManager = (BluetoothManager) getSystemService(Context.BLUETOOTH_SERVICE);
bluetoothAdapter = bluetoothManager.getAdapter();
BluetoothDevice device = bluetoothAdapter.getRemoteDevice("device_address");
bluetoothGatt = device.connectGatt(this, false, bluetoothGattCallback);
}
@Override
protected void onDestroy() {
super.onDestroy();
if(bluetoothGatt != null) {
bluetoothGatt.disconnect();
bluetoothGatt.close();
}
}
}
```
在这个示例代码中,我们首先获取了系统的BluetoothManager和BluetoothAdapter对象,然后通过BluetoothAdapter获取了要连接的远程设备的BluetoothDevice对象。接下来,我们通过BluetoothDevice对象调用connectGatt()方法建立与设备的BLE连接,并传入一个BluetoothGattCallback对象作为回调函数。
在BluetoothGattCallback中,我们实现了三个回调函数:onConnectionStateChange()、onServicesDiscovered()和onCharacteristicChanged()。其中,onConnectionStateChange()用于处理BLE连接状态的变化,当连接建立成功时,我们调用discoverServices()方法发现设备的服务和特征。在onServicesDiscovered()回调中,我们首先获取指定UUID的服务和特征,然后设置特征的通知使得设备能够向我们发送数据。最后,我们将特征的描述符设置为ENABLE_NOTIFICATION_VALUE,并发送给设备。当设备有数据发送过来时,onCharacteristicChanged()回调函数将被调用,我们可以在其中获取到设备发送的数据并进行处理。
需要注意的是,这个示例代码中的UUID、设备地址等信息需要根据实际情况进行修改。另外,由于BLE的连接和数据传输是异步进行的,因此需要注意回调函数的执行顺序和时机,以保证代码的正确性。
希望这个示例代码能够对你有所帮助!
相关推荐
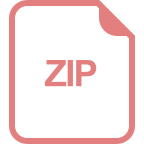
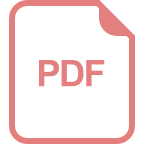
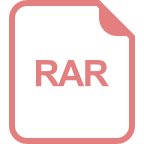














