请基于python编写一段程序,该程序可以识别照片并指出照片中的内容是什么
时间: 2024-10-16 08:06:37 浏览: 21
在Python中,我们可以使用开源的计算机视觉库如OpenCV和深度学习框架如TensorFlow或PyTorch来识别图像中的物体。这里我们将展示一个基础的例子,使用预训练的模型`tf.keras.applications.imagenet_utils.decode_predictions()`,这个模型是为ImageNet数据集设计的,但可能不适用于所有的场景。
首先,确保已经安装了所需的库:
```bash
pip install opencv-python
pip install tensorflow
```
然后,使用以下代码作为起点:
```python
import cv2
import numpy as np
from tensorflow.keras.applications.resnet50 import ResNet50, decode_predictions
# 加载预训练的ResNet50模型(没有包括权重)
model = ResNet50(weights='imagenet', include_top=True)
def predict_image(image_path):
# 读取图片
img = cv2.imread(image_path)
if img is None:
raise ValueError("Failed to load image")
# 预处理图片(归一化、转为RGB格式等)
img = cv2.resize(img, (224, 224))
img = img.astype(np.float32) / 255.0
img = np.expand_dims(img, axis=0)
# 进行预测
predictions = model.predict(img)
# 解码并获取概率最高的类别
decoded_preds = decode_predictions(predictions, top=1)
class_name = decoded_preds # 获取类别名称
confidence = decoded_preds * 100 # 获取置信度百分比
return class_name, confidence
# 示例用法
image_path = 'path_to_your_image.jpg'
class_name, confidence = predict_image(image_path)
print(f"图片中的主要物体是:{class_name},置信度为:{confidence}%")
```
注意这只是一个基本示例,实际应用中可能需要更复杂的图像预处理步骤(如颜色空间转换、目标检测等),以及更精确的模型,如YOLOv3或Faster R-CNN。
阅读全文
相关推荐
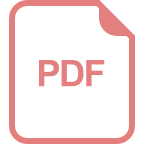
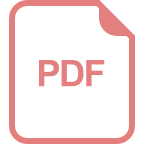
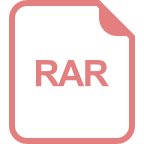
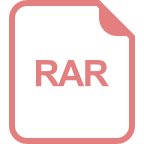
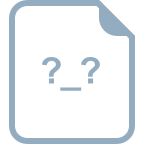
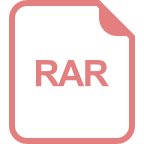
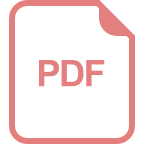
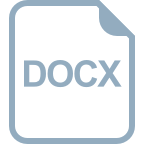
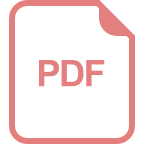
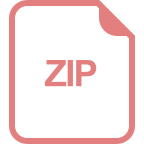
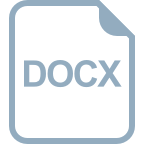
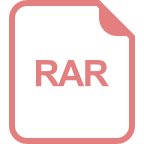
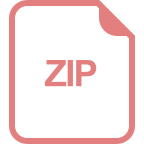
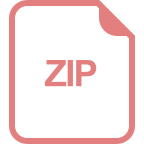
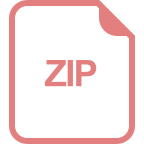
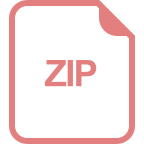
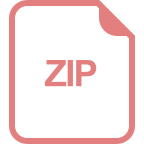
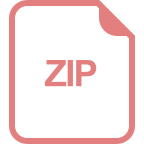
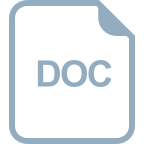
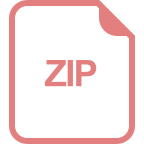