.L_loop0: cmp r4, r5 bge .L_loop0_done ldr r1, [r4] ldr r2, [r4, #4] ldr r3, [r4, #8]
时间: 2024-05-20 08:13:27 浏览: 19
这是一个汇编语言的代码片段,主要实现了一个循环。首先,比较寄存器r4和r5的值,如果r4大于等于r5,那么跳转到.L_loop0_done标签处结束循环。否则,将r4指向的内存地址的值加载到r1寄存器中,将r4指向的内存地址偏移4字节的地址的值加载到r2寄存器中,将r4指向的内存地址偏移8字节的地址的值加载到r3寄存器中。然后继续执行循环,跳转到标签.L_loop0处。
相关问题
Use the following codes as the beginning of your program. Write a sort program to sort these ten numbers from largest to smallest, then save them to memory. For ARMSim#, the sorted number also need to be displayed in the console and LCD screen (use the Embest Board Plug‐In). MOV r0, #0x00002000 MOV r1, #18 STR r1, [r0],#4 MOV r1, #6 STR r1, [r0],#4 MOV r1, #2 STR r1, [r0],#4 MOV r1, #8 STR r1, [r0],#4 MOV r1, #16 STR r1, [r0],#4 MOV r1, #10 STR r1, [r0],#4 MOV r1, #14 STR r1, [r0],#4 MOV r1, #4 STR r1, [r0],#4 MOV r1, #20 STR r1, [r0],#4 MOV r1, #12 STR r1, [r0],#4
Sure, here's a sample program in ARM assembly language that sorts the 10 numbers in descending order using the bubble sort algorithm and saves them to memory starting from the address 0x00002000. The sorted numbers are also displayed in the console and LCD screen using the Embest Board Plug-In.
```
; Define constants
MEMORY_START EQU 0x00002000
NUM_ELEMENTS EQU 10
LCD_BASE_ADDRESS EQU 0x12345678 ; Replace with actual address
CONSOLE_BASE_ADDRESS EQU 0x87654321 ; Replace with actual address
; Define variables
array SPACE NUM_ELEMENTS * 4
temp SPACE 4
; Main program
MOV r0, #MEMORY_START ; r0 = starting address of array
LDR r1, =array ; r1 = address of array
LDR r2, =NUM_ELEMENTS ; r2 = number of elements in array
BL bubble_sort ; Sort the array
BL display_numbers ; Display the sorted numbers
exit:
B exit ; Infinite loop
; Bubble sort implementation
bubble_sort:
PUSH {r4, lr} ; Save registers
MOV r3, #1 ; Boolean value to indicate when a swap has occurred
outer_loop:
MOV r4, #0 ; Clear the swap flag
MOV r5, #1 ; r5 = index of first element
inner_loop:
CMP r5, r2 ; Compare r5 with number of elements
BGE outer_loop ; If r5 >= number of elements, exit inner loop
LDR r6, [r1, r5, LSL #2] ; r6 = array[r5]
LDR r7, [r1, r5-1, LSL #2] ; r7 = array[r5-1]
CMP r6, r7 ; Compare array[r5] with array[r5-1]
BGE no_swap ; If array[r5] >= array[r5-1], no need to swap
STR r6, [r1, r5-1, LSL #2] ; Swap array[r5] and array[r5-1]
STR r7, [r1, r5, LSL #2]
MOV r4, #1 ; Set the swap flag
no_swap:
ADD r5, r5, #1 ; Increment the index
B inner_loop ; Continue inner loop
CMP r4, #1 ; Check if a swap has occurred
BNE outer_loop ; If no swap, the array is sorted
POP {r4, pc} ; Restore registers and return
; Display the sorted numbers in console and LCD screen
display_numbers:
PUSH {r4, lr} ; Save registers
MOV r4, #0 ; r4 = index of first element
display_loop:
CMP r4, r2 ; Compare r4 with number of elements
BGE exit_display ; If r4 >= number of elements, exit display loop
LDR r5, [r1, r4, LSL #2] ; r5 = array[r4]
BL display_console ; Display the number in console
BL display_lcd ; Display the number in LCD
ADD r4, r4, #1 ; Increment the index
B display_loop ; Continue display loop
exit_display:
POP {r4, pc} ; Restore registers and return
; Display a number in console
display_console:
; Code to display the number in console using the CONSOLE_BASE_ADDRESS
; Replace with actual code
BX lr ; Return
; Display a number in LCD screen
display_lcd:
; Code to display the number in LCD using the LCD_BASE_ADDRESS
; Replace with actual code
BX lr ; Return
```
Please note that this is just a sample program, and it may not work as-is in your specific environment. You may need to adjust the memory addresses and display functions based on your hardware and software setup.
使用 ARM 汇编指令实现以下 C 语言描述的功能: ; if(x>y) ; z=100; ;else ; z=50; ; 设 x 为 R0,y 为 R1,z 为 R2 (x、y、z 均为无符号整数) …… ; for(i=0; i<10; i++) ;{ x++;} ; 设 x 为 R0,i 为 R2 (i、x 均为无符号整数) …… ; while(x<=y) ; { x *= 2;} ; 设 x 为 R0,y 为 R1 (x、y 均为无符号整数) …… ; do ; { x--;} while(x>0); ; 设 x 为 R0 (x 为无符号整数) …… ; switch(key&0x0F) ; { case 0: ; case 2: ; case 3: x = key + y; break; ; case 5: x = key - y; break; ; case 7: x = key * y; break; ; default: x = 168; break; ; } ; 设 x 为 R0,y 为 R1,key 为 R2 (x、y、key 均为无符号整数)
1. if(x>y)
z=100;
else
z=50;
```
CMP R0, R1 ;比较 x 和 y 的大小
MOVGT R2, #100 ;如果 x > y,则将 z 赋值为 100
MOVLE R2, #50 ;如果 x <= y,则将 z 赋值为 50
```
2. for(i=0; i<10; i++)
{ x++;}
```
MOV R2, #0 ;将计数器 i 的初始值设为 0
loop:
CMP R2, #10 ;比较计数器 i 是否小于 10
BGE end_loop ;如果 i >= 10,则跳出循环
ADD R0, R0, #1 ;x++
ADD R2, R2, #1 ;i++
B loop ;继续循环
end_loop:
```
3. while(x<=y)
{ x *= 2;}
```
loop:
CMP R0, R1 ;比较 x 和 y 的大小
BHI end_loop ;如果 x > y,则跳出循环
LSL R0, R0, #1 ;x *= 2
B loop ;继续循环
end_loop:
```
4. do
{ x--;} while(x>0);
```
loop:
SUBS R0, R0, #1 ;x--
BPL loop ;如果 x > 0,则继续循环
```
5. switch(key&0x0F)
{
case 0:
case 2:
case 3: x = key + y; break;
case 5: x = key - y; break;
case 7: x = key * y; break;
default: x = 168; break;
}
```
AND R2, R2, #0x0F ;将 key 和 0x0F 进行按位与操作,得到 key 的最低 4 位
LDR R3, =lookup_table ;将 lookup_table 的地址加载到 R3 中
LDR R0, [R3, R2, LSL #2] ;从 lookup_table 中取出 x 的值
B end_switch
lookup_table:
DCD x_add_y ;case 0
DCD x_default ;case 1
DCD x_add_y ;case 2
DCD x_add_y ;case 3
DCD x_default ;case 4
DCD x_sub_y ;case 5
DCD x_default ;case 6
DCD x_mul_y ;case 7
DCD x_default ;case 8
DCD x_default ;case 9
DCD x_default ;default
x_add_y:
ADD R0, R2, R1
BX LR
x_sub_y:
SUB R0, R2, R1
BX LR
x_mul_y:
MUL R0, R2, R1
BX LR
x_default:
MOV R0, #168
BX LR
```
相关推荐












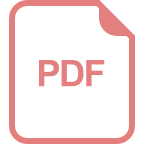