用c++解决下述问题:描述 Akko is learning magic in Luna Nova Magical Academy. It is said that there are 10^5 types of different curses in the magic world. It is too hard for Akko to memorize all of them. Fortunately, Proffesor Meridies says that she can help Akko make a Curse Dictionary. When Akko hears a curse, the Dictionary will tell her the effect of the curse. Also, when Akko wants to do something, the Dictionary will find the curse for her. If there is no such curse, the Dictionary will be silence. Can you write a program to reproduce the Curse Dictionary? 输入 Each test case will contain no more than 10^5 curses. Each curse will be like "[name] effect". The name and effect of the curse will only contain lowercase letters. There will be only one space between ']' and the string after it. The length of the name will not exceed 20 and the length of the effect will not exceed 80. The end of the dictionary is a line of "END". After all curses there will be an integer N (1<=N<1000), followed by N queries. Every query will be a "[curse name]" or a "curse effect". There will be only one test case in each input file. 输出 For each test case, you must output the effect of the curse by its name or the name of the curse by its effect. If there is no curse satisfying the condition, print "silence".
时间: 2024-02-14 14:10:39 浏览: 135
以下是用C++解决这道题的代码实现:
```cpp
#include <iostream>
#include <string>
#include <unordered_map>
using namespace std;
int main() {
unordered_map<string, string> curses; // 用哈希表存储咒语和效果的对应关系
// 读入咒语和效果
string curse;
while (getline(cin, curse) && curse != "END") {
string name = curse.substr(1, curse.find(']') - 1);
string effect = curse.substr(curse.find(']') + 2);
curses[name] = effect;
}
// 处理查询请求
int n;
cin >> n;
getchar(); // 读入换行符
while (n--) {
string query;
getline(cin, query);
if (query[0] == '[') { // 根据咒语名称查询对应效果
string name = query.substr(1, query.size() - 2);
if (curses.count(name)) {
cout << curses[name] << endl;
} else {
cout << "silence" << endl;
}
} else { // 根据效果查询对应咒语名称
bool found = false;
for (auto it = curses.begin(); it != curses.end(); ++it) {
if (it->second == query) {
cout << "[" << it->first << "]" << endl;
found = true;
break;
}
}
if (!found) {
cout << "silence" << endl;
}
}
}
return 0;
}
```
思路如下:
1. 用 `unordered_map` 存储咒语和效果的对应关系,咒语名称作为键,效果作为值。
2. 读入咒语和效果,将其存储到哈希表中。
3. 处理查询请求,如果查询请求以 `[` 开头,则根据咒语名称查询对应效果;否则,根据效果查询对应咒语名称。
4. 输出查询结果。
注意事项:
1. 读入咒语和效果时,要使用 `getline` 函数,因为每行可能包含空格。
2. 读入查询请求时,要先读入一个换行符,否则可能会将上一行的回车符作为查询请求的第一个字符。
3. 在输出查询结果时,要注意加上换行符。
阅读全文
相关推荐

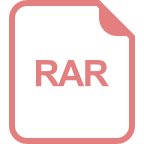
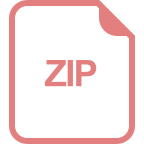
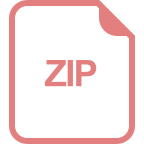
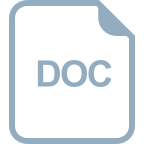
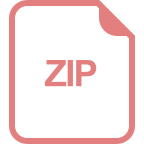