HttpClient调取完接口后怎么用
时间: 2023-05-17 07:03:19 浏览: 102
您可以使用HttpClient的getResponse方法来获取接口返回的数据,然后根据接口返回的数据进行相应的处理。以下是一个示例代码:
HttpClient httpClient = new HttpClient();
GetMethod getMethod = new GetMethod("http://www.example.com/api");
httpClient.executeMethod(getMethod);
String response = getMethod.getResponseBodyAsString();
// 对接口返回的数据进行处理
当然,具体的处理方式需要根据接口返回的数据格式和业务需求来确定。
相关问题
java如何调取第三方接口
在 Java 中调用第三方接口需要使用 Java 提供的网络请求 API,通常有两种方式:
1. 使用 HttpURLConnection 类发送 HTTP 请求
HttpURLConnection 是 Java 中用于发送 HTTP 请求的类,可以使用此类发送 GET、POST、PUT、DELETE 等请求。具体步骤如下:
```java
// 创建 URL 对象
URL url = new URL("http://example.com/api");
// 打开连接
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
// 设置请求方法
conn.setRequestMethod("GET");
// 设置请求头
conn.setRequestProperty("Content-Type", "application/json");
// 发送请求
conn.connect();
// 获取响应码
int responseCode = conn.getResponseCode();
// 获取响应数据
InputStream inputStream = conn.getInputStream();
// 读取响应数据
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream));
String line;
StringBuilder response = new StringBuilder();
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
inputStream.close();
// 关闭连接
conn.disconnect();
// 处理响应数据
System.out.println(response.toString());
```
2. 使用 HttpClient 库发送 HTTP 请求
HttpClient 是 Apache 提供的开源 HTTP 客户端库,可以用于发送 HTTP 请求,支持 GET、POST、PUT、DELETE 等请求。具体步骤如下:
```java
// 创建 HttpClient 对象
CloseableHttpClient httpClient = HttpClients.createDefault();
// 创建 HttpGet 或 HttpPost 对象
HttpGet httpGet = new HttpGet("http://example.com/api");
// 设置请求头
httpGet.setHeader("Content-Type", "application/json");
// 发送请求
CloseableHttpResponse response = httpClient.execute(httpGet);
// 获取响应数据
HttpEntity entity = response.getEntity();
String result = EntityUtils.toString(entity, "UTF-8");
// 关闭响应和连接
response.close();
httpClient.close();
// 处理响应数据
System.out.println(result);
```
需要注意的是,在调用第三方接口时,需要遵循接口提供方的协议和规范,如授权、请求频率等限制。同时,为了保证数据安全,建议将敏感信息加密传输。
使用Feign客户端调取的方法步骤,请举例描述
Feign是一个轻量级、声明式的HTTP客户端库,用于简化微服务之间基于RESTful API的通信。以下是使用Feign客户端调取远程API的基本步骤:
1. 添加依赖:首先在你的项目中引入Feign的依赖,通常是在Maven或Gradle构建文件中。例如,在Maven中添加:
```xml
<dependency>
<groupId>io.github.openfeign</groupId>
<artifactId>feign-okhttp</artifactId>
<!-- 版本号 -->
<version>9.8.0</version>
</dependency>
```
2. 创建接口:定义一个接口,这个接口模拟了你要调用的实际服务API。接口方法签名应与远程服务的HTTP请求对应。例如:
```java
public interface UserApi {
@GetMapping("/users/{id}")
User getUser(@PathVariable("id") Long id);
@PostMapping("/register")
RegisterResponse register(RegisterRequest request);
}
```
3. 定义Feign配置:如果需要自定义超时、编码等设置,可以在`@FeignClient`注解上配置。如果没有特殊需求,一般可以省略。
```java
@FeignClient(name = "api-server", url = "https://example.com/api")
public interface UserApi {
// ...
}
```
4. 实现客户端:创建一个实现该接口的类,并由Feign自动调用远程接口完成实际请求。例如:
```java
@Configuration
@EnableFeignClients
public class FeignConfiguration {
@Bean
public UserApi userApi() {
return new UserApiImplementation();
}
}
@Service
class UserApiImplementation implements UserApi {
@Override
public User getUser(Long id) {
// 调用实际的HTTP GET请求并返回响应结果
return httpClient.get(User.class, "/users/" + id);
}
// 其他方法的实现同理
}
```
5. 调用服务:现在可以从服务提供者(通常是业务模块)的任何地方通过`UserApi`接口来调用Feign客户端提供的服务。
阅读全文
相关推荐
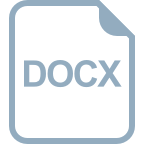
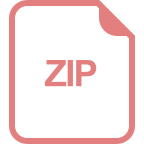
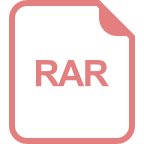
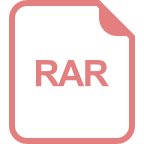
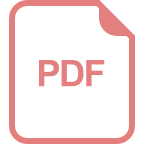
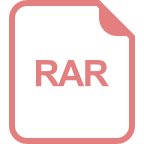

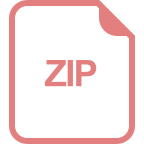