bouncycastle generate IPEK according to BDK and KSN example
时间: 2024-05-12 11:19:10 浏览: 118
As an AI language model, I don't have access to examples of keys or sensitive information. However, I can provide you with the general steps for generating an IPEK using BouncyCastle:
1. Generate a random 16-byte key, called the "Key Encryption Key" (KEK).
2. Using the KEK and the BDK (Base Derivation Key), generate a 16-byte key called the "Initial PIN Encryption Key" (IPEK).
3. XOR the IPEK with the first 8 bytes of the KSN (Key Serial Number) to generate the "PIN Encryption Key" (PEK).
4. XOR the IPEK with the second 8 bytes of the KSN to generate the "Data Encryption Key" (DEK).
Here's the Java code using BouncyCastle:
```
import org.bouncycastle.crypto.engines.AESEngine;
import org.bouncycastle.crypto.modes.CBCBlockCipher;
import org.bouncycastle.crypto.params.KeyParameter;
import org.bouncycastle.crypto.params.ParametersWithIV;
import org.bouncycastle.util.encoders.Hex;
public class IpekGenerator {
private static final byte[] BDK = Hex.decode("0123456789ABCDEFFEDCBA9876543210");
private static final byte[] KSN = Hex.decode("FFFF9876543210E00001");
public static void main(String[] args) {
// Generate KEK
byte[] kek = new byte[16];
new SecureRandom().nextBytes(kek);
// Generate IPEK
byte[] ipek = generateIpek(BDK, kek);
System.out.println("IPEK: " + Hex.toHexString(ipek));
// Generate PEK and DEK
byte[] pek = generatePek(ipek, KSN);
byte[] dek = generateDek(ipek, KSN);
System.out.println("PEK: " + Hex.toHexString(pek));
System.out.println("DEK: " + Hex.toHexString(dek));
}
private static byte[] generateIpek(byte[] bdk, byte[] kek) {
byte[] data = new byte[16];
System.arraycopy(kek, 0, data, 0, 8);
System.arraycopy(bdk, 0, data, 8, 8);
CBCBlockCipher cipher = new CBCBlockCipher(new AESEngine());
ParametersWithIV params = new ParametersWithIV(new KeyParameter(bdk), new byte[16]);
cipher.init(true, params);
byte[] ipek = new byte[16];
cipher.processBlock(data, 0, ipek, 0);
return ipek;
}
private static byte[] generatePek(byte[] ipek, byte[] ksn) {
byte[] pek = new byte[8];
for (int i = 0; i < 8; i++) {
pek[i] = (byte) (ipek[i] ^ ksn[i]);
}
return pek;
}
private static byte[] generateDek(byte[] ipek, byte[] ksn) {
byte[] dek = new byte[8];
for (int i = 0; i < 8; i++) {
dek[i] = (byte) (ipek[i + 8] ^ ksn[i]);
}
return dek;
}
}
```
阅读全文
相关推荐
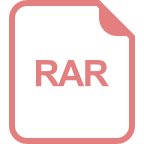
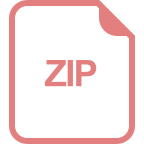
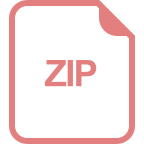
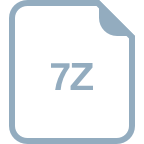










