编程:编写一程序(应该有多个函数),允许 从键盘输入任意多个英语单词(单词可以重 复),中间用空格分开,输入0表示输入结 束。该程序可以统计同一个英语单词被输入几次,最后对英文单词按字典顺序输出,后面跟上该单词被输入的次数。(提示,尝试用结构体组织数据,把单词和该单出现的次数用一个结构体来描述。
时间: 2023-05-25 17:03:24 浏览: 93
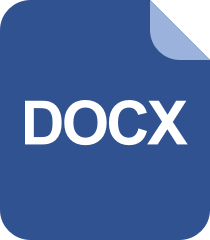
从键盘任意输入两个整数,输出两数之间的素数,素数判断用函数实现。 扩展要求:1)程序可以循环执行,判断完一组可以再进行下一组;可以设定一个特殊键退出

#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义结构体
typedef struct wordCount {
char* word; // 单词
int count; // 出现次数
} WordCount;
// 比较函数,用于 qsort 排序
int cmp(const void* a, const void* b) {
WordCount* wa = (WordCount*)a;
WordCount* wb = (WordCount*)b;
return strcmp(wa->word, wb->word);
}
int main() {
int capacity = 10; // 数组容量
int count = 0; // 实际元素数量
WordCount* words = (WordCount*)malloc(capacity * sizeof(WordCount)); // 动态数组
// 循环读取单词,并添加到数组中
while (1) {
char input[100];
scanf("%s", input);
if (strcmp(input, "0") == 0) {
break;
}
// 查找单词是否已存在于数组中
int found = 0;
for (int i = 0; i < count; i++) {
if (strcmp(words[i].word, input) == 0) {
found = 1;
words[i].count++;
break;
}
}
// 如果单词不存在,则新增一个元素到数组中
if (!found) {
if (count == capacity) {
capacity *= 2;
words = (WordCount*)realloc(words, capacity * sizeof(WordCount));
}
words[count].word = (char*)malloc(strlen(input) + 1);
strcpy(words[count].word, input);
words[count].count = 1;
count++;
}
}
// 按字典顺序排序
qsort(words, count, sizeof(WordCount), cmp);
// 输出结果
for (int i = 0; i < count; i++) {
printf("%s %d\n", words[i].word, words[i].count);
free(words[i].word);
}
free(words);
return 0;
}
阅读全文
相关推荐
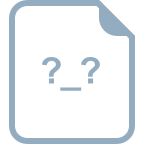
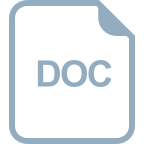
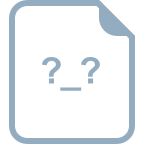
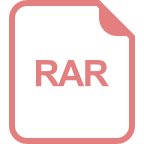
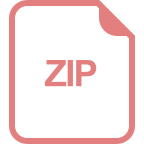
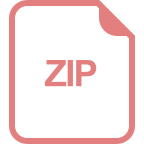
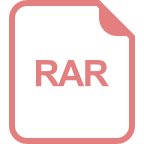
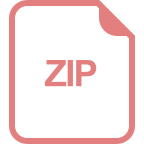
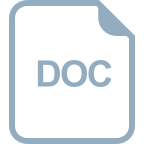
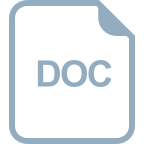
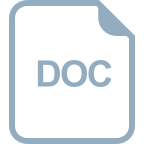
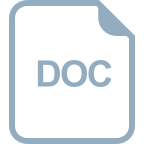
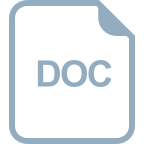
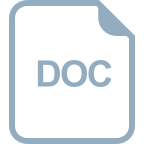
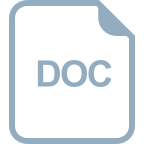
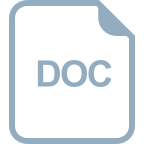
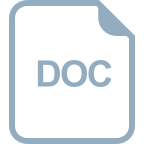
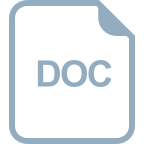