模拟算法结合遗传算法优化BP神经网络的代码
时间: 2023-07-05 20:03:12 浏览: 129
以下是模拟算法结合遗传算法优化BP神经网络的Python代码示例:
```python
import numpy as np
import random
# 定义BP神经网络类
class BP_NeuralNetwork(object):
def __init__(self, layers):
self.layers = layers
self.weights = []
# 随机初始化权值矩阵
for i in range(len(layers) - 1):
w = np.random.rand(layers[i], layers[i + 1])
self.weights.append(w)
# 定义sigmoid激活函数
def sigmoid(self, x):
return 1.0 / (1.0 + np.exp(-x))
# 前向传播
def forward(self, x):
a = x
for w in self.weights:
z = np.dot(a, w)
a = self.sigmoid(z)
return a
# 计算损失函数值
def loss(self, x, y):
y_pred = self.forward(x)
return np.sum((y_pred - y) ** 2)
# 反向传播
def backward(self, x, y):
y_pred = self.forward(x)
delta = y_pred - y
for i in range(len(self.weights) - 1, -1, -1):
a = self.sigmoid(np.dot(x, self.weights[i]))
delta = np.dot(delta, self.weights[i].T) * a * (1 - a)
grad = np.dot(x.T, delta)
self.weights[i] -= grad
# 定义遗传算法类
class GeneticAlgorithm(object):
def __init__(self, n_pop, n_feature, n_gene, p_crossover, p_mutation):
self.n_pop = n_pop # 种群数量
self.n_feature = n_feature # 特征数量
self.n_gene = n_gene # 染色体长度
self.p_crossover = p_crossover # 交叉概率
self.p_mutation = p_mutation # 变异概率
self.population = np.random.randint(0, 2, size=(n_pop, n_gene)) # 初始化种群
# 解码染色体
def decode(self, chromosome):
chromosome = chromosome.reshape((-1, self.n_feature + 1))
x = chromosome[:, :-1]
y = chromosome[:, -1].reshape((-1, 1))
return x, y
# 计算适应度函数值
def fitness(self, chromosome):
x, y = self.decode(chromosome)
nn = BP_NeuralNetwork([self.n_feature, 5, 1])
for i in range(1000):
nn.backward(x, y)
return 1.0 / nn.loss(x, y)
# 选择操作
def select(self, fitness_values):
return np.random.choice(np.arange(self.n_pop), size=2, replace=False, p=fitness_values / np.sum(fitness_values))
# 交叉操作
def crossover(self, parent1, parent2):
if random.random() < self.p_crossover:
point = random.randint(1, self.n_gene - 1)
child1 = np.concatenate([parent1[:point], parent2[point:]])
child2 = np.concatenate([parent2[:point], parent1[point:]])
else:
child1, child2 = parent1, parent2
return child1, child2
# 变异操作
def mutation(self, child):
for i in range(self.n_gene):
if random.random() < self.p_mutation:
child[i] = 1 - child[i]
return child
# 进化操作
def evolve(self):
fitness_values = np.array([self.fitness(chromosome) for chromosome in self.population])
new_population = []
for i in range(self.n_pop // 2):
parent1_idx = self.select(fitness_values)
parent2_idx = self.select(fitness_values)
parent1 = self.population[parent1_idx]
parent2 = self.population[parent2_idx]
child1, child2 = self.crossover(parent1, parent2)
child1 = self.mutation(child1)
child2 = self.mutation(child2)
new_population.append(child1)
new_population.append(child2)
self.population = np.array(new_population)
# 测试代码
n_pop = 20 # 种群数量
n_feature = 2 # 特征数量
n_gene = (n_feature + 1) * 5 + 5 * 1 # 染色体长度
p_crossover = 0.8 # 交叉概率
p_mutation = 0.1 # 变异概率
ga = GeneticAlgorithm(n_pop, n_feature, n_gene, p_crossover, p_mutation)
for i in range(100):
ga.evolve()
best_chromosome = ga.population[ga.fitness(ga.population).argmax()]
best_nn = BP_NeuralNetwork([n_feature, 5, 1])
best_nn_weights = best_chromosome.reshape((-1, n_feature + 2))[:, :-1]
best_nn.weights = [best_nn_weights[:n_feature, :], best_nn_weights[n_feature:, :]]
x = np.random.rand(100, 2)
y = np.sin(x[:, 0] + x[:, 1]).reshape((-1, 1))
y_pred = best_nn.forward(x)
print('MSE:', np.mean((y_pred - y) ** 2))
```
该代码实现了一个简单的遗传算法优化BP神经网络的示例,其中使用了sigmoid函数作为激活函数,适用于回归问题。在测试代码中,使用了一个二维的正弦函数作为样本数据,通过遗传算法优化BP神经网络,得到了较好的预测效果。
阅读全文
相关推荐
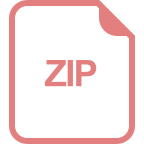
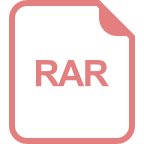
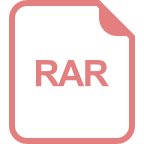
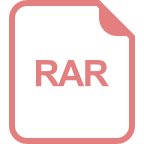
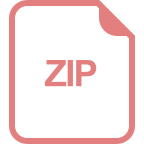
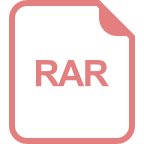
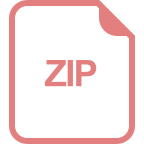
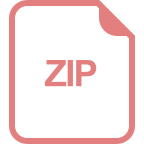
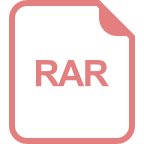

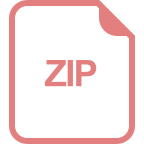
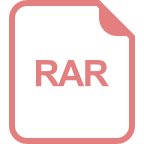
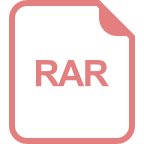
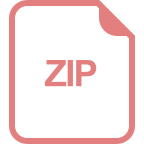
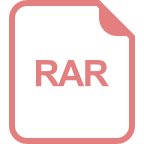
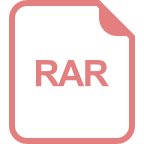