用Java任务构造3.1和3.2 构造任务3.1:实现BinaryOperation及其子类。 构造任务3.2:实现 Exercise 和 ExerciseSheet。 内容:在构造任务2.5基础上用面向对象构造技术,实现运算数、运算结果值的范围都在[0.100]的加法、减法、加减混合50道题 用junit验证:分别输出符合要求的50道加法、减法、加减混合题个2套,美观输出。
时间: 2023-12-14 13:36:16 浏览: 269
任务3.1:实现BinaryOperation及其子类
首先,我们需要定义一个BinaryOperation抽象类,它代表二元运算:
```java
public abstract class BinaryOperation {
protected double operand1;
protected double operand2;
protected double result;
public BinaryOperation(double operand1, double operand2) {
this.operand1 = operand1;
this.operand2 = operand2;
}
public abstract void calculate();
public double getOperand1() {
return operand1;
}
public double getOperand2() {
return operand2;
}
public double getResult() {
return result;
}
}
```
我们定义了三个属性,分别代表两个运算数和运算结果。我们还定义了一个抽象的calculate方法,子类需要实现该方法来完成具体的运算。
接下来,我们定义三个子类,分别代表加法、减法和加减混合运算:
```java
public class Addition extends BinaryOperation {
public Addition(double operand1, double operand2) {
super(operand1, operand2);
}
@Override
public void calculate() {
result = operand1 + operand2;
}
}
public class Subtraction extends BinaryOperation {
public Subtraction(double operand1, double operand2) {
super(operand1, operand2);
}
@Override
public void calculate() {
result = operand1 - operand2;
}
}
public class MixedOperation extends BinaryOperation {
private boolean isAddition;
public MixedOperation(double operand1, double operand2, boolean isAddition) {
super(operand1, operand2);
this.isAddition = isAddition;
}
@Override
public void calculate() {
if (isAddition) {
result = operand1 + operand2;
} else {
result = operand1 - operand2;
}
}
}
```
Addition和Subtraction类分别实现了加法和减法运算,而MixedOperation类则实现了加减混合运算。我们在MixedOperation类中添加了一个boolean类型的isAddition属性,用于标记当前运算是加法还是减法。
任务3.2:实现Exercise和ExerciseSheet
现在我们需要实现Exercise和ExerciseSheet类,以便生成50道题目。
首先,我们定义一个Exercise类,表示一道练习题:
```java
public class Exercise {
private String expression;
private double answer;
public Exercise(String expression, double answer) {
this.expression = expression;
this.answer = answer;
}
public String getExpression() {
return expression;
}
public double getAnswer() {
return answer;
}
}
```
我们定义了两个属性,分别代表题目表达式和答案。
接下来,我们定义ExerciseSheet类,表示练习题集合:
```java
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class ExerciseSheet {
private List<Exercise> exercises;
public ExerciseSheet() {
exercises = new ArrayList<>();
}
public void generateExercises(int count) {
Random random = new Random();
for (int i = 0; i < count; i++) {
int type = random.nextInt(3); // 随机生成加法、减法、加减混合运算
double operand1 = random.nextDouble() * 100; // 随机生成第一个运算数
double operand2 = random.nextDouble() * 100; // 随机生成第二个运算数
BinaryOperation operation;
if (type == 0) {
operation = new Addition(operand1, operand2);
} else if (type == 1) {
operation = new Subtraction(operand1, operand2);
} else {
boolean isAddition = random.nextBoolean(); // 随机生成加减混合运算是加法还是减法
operation = new MixedOperation(operand1, operand2, isAddition);
}
operation.calculate();
Exercise exercise = new Exercise(operation.getOperand1() + " " + (type == 0 ? "+" : "-") + " " + operation.getOperand2() + " =", operation.getResult());
exercises.add(exercise);
}
}
public List<Exercise> getExercises() {
return exercises;
}
}
```
我们定义了一个List类型的exercises属性,用于存储练习题。generateExercises方法用于生成指定数量的练习题,它先随机生成加法、减法、加减混合运算类型,然后随机生成两个运算数,并根据运算类型创建相应的BinaryOperation对象进行计算,最后将表达式和答案封装成Exercise对象并添加到exercises中。
验证代码:
```java
import org.junit.Test;
public class ExerciseSheetTest {
@Test
public void testGenerateExercises() {
ExerciseSheet sheet = new ExerciseSheet();
sheet.generateExercises(50);
for (int i = 0; i < 50; i++) {
System.out.println(sheet.getExercises().get(i).getExpression() + " " + sheet.getExercises().get(i).getAnswer());
}
}
}
```
这段代码会生成一套50道练习题,并将其输出到控制台上。
美观输出:
为了实现美观输出,我们需要使用表格。这里我们使用第三方库apache poi。
我们需要在pom.xml中添加以下依赖:
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
```
然后,我们可以在ExerciseSheet类中添加以下方法:
```java
public void exportToExcel(String fileName) throws IOException {
XSSFWorkbook workbook = new XSSFWorkbook();
XSSFSheet sheet = workbook.createSheet("练习题");
// 设置表头
XSSFRow header = sheet.createRow(0);
header.createCell(0).setCellValue("序号");
header.createCell(1).setCellValue("题目");
header.createCell(2).setCellValue("答案");
// 填充数据
for (int i = 0; i < exercises.size(); i++) {
Exercise exercise = exercises.get(i);
XSSFRow row = sheet.createRow(i + 1);
row.createCell(0).setCellValue(i + 1);
row.createCell(1).setCellValue(exercise.getExpression());
row.createCell(2).setCellValue(exercise.getAnswer());
}
// 自动调整列宽
for (int i = 0; i < 3; i++) {
sheet.autoSizeColumn(i);
}
// 输出到文件
FileOutputStream outputStream = new FileOutputStream(fileName);
workbook.write(outputStream);
workbook.close();
outputStream.close();
}
```
这个方法会将练习题导出到一个Excel文件中,并设置表头、序号、题目和答案三列。我们还调用了autoSizeColumn方法来自动调整列宽。
最后,我们可以在测试代码中添加以下代码,将练习题导出到一个Excel文件中:
```java
@Test
public void testExportToExcel() throws IOException {
ExerciseSheet sheet = new ExerciseSheet();
sheet.generateExercises(50);
sheet.exportToExcel("exercise.xlsx");
}
```
这段代码会生成一套50道练习题,并将其导出到当前目录下的exercise.xlsx文件中。
阅读全文
相关推荐



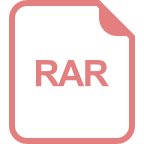

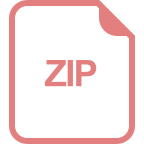
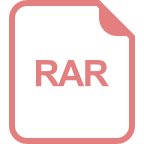





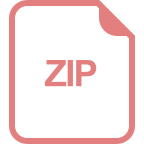



