Java JSch在远程机器上更改用户 并输入密码并执行多条命令
时间: 2023-12-22 19:04:29 浏览: 149
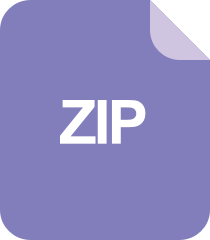
Jsch批量执行多条命令,包含切换用户输入密码
使用Java JSch可以连接到远程机器并执行命令,下面是一个示例代码,可以更改用户并执行多条命令:
```java
import com.jcraft.jsch.*;
public class SSHExample {
public static void main(String[] args) {
String user = "username"; // 远程机器的用户名
String password = "password"; // 远程机器的密码
String host = "remote_host"; // 远程机器的IP地址或域名
int port = 22; // 远程机器的SSH端口号,默认是22
try {
JSch jsch = new JSch();
Session session = jsch.getSession(user, host, port);
session.setPassword(password);
session.setConfig("StrictHostKeyChecking", "no");
session.connect();
// 更改用户
String command = "sudo su - otheruser"; // 需要更改的用户
Channel channel = session.openChannel("exec");
((ChannelExec) channel).setCommand(command);
channel.connect();
// 输入密码
String sudoPassword = "otheruser_password"; // 需要输入的密码
channel = session.openChannel("exec");
((ChannelExec) channel).setCommand("echo \"" + sudoPassword + "\" | sudo -S ls");
channel.connect();
// 执行多条命令
String[] commands = {"cd /path/to/directory", "ls -l", "date"};
for (String cmd : commands) {
channel = session.openChannel("exec");
((ChannelExec) channel).setCommand(cmd);
channel.setInputStream(null);
((ChannelExec) channel).setErrStream(System.err);
channel.connect();
channel.disconnect();
}
session.disconnect();
} catch (JSchException e) {
e.printStackTrace();
}
}
}
```
在这个示例中,我们先通过JSch连接到远程机器,然后使用`sudo su - otheruser`命令切换到需要更改的用户,接着使用`echo`命令和管道符号`|`输入密码,最后使用`openChannel`方法打开一个新的`Channel`并执行多条命令。
阅读全文
相关推荐
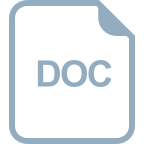
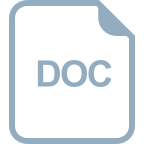
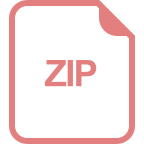
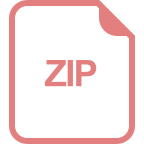
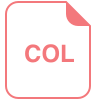
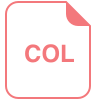
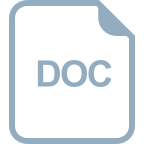
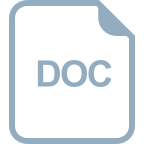
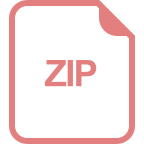
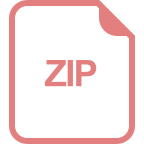
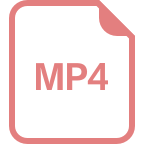
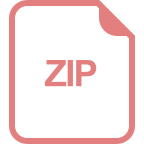
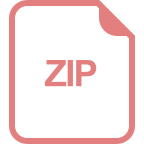
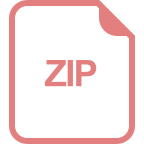
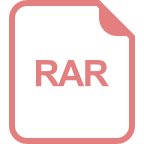
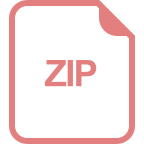