将整数转换成字符串的函数C++
时间: 2024-09-08 10:02:00 浏览: 60
在C++中,将整数转换成字符串有多种方法。以下是几种常见的转换方式:
1. 使用`std::to_string`函数:
自C++11起,标准库中引入了`std::to_string`函数,可以直接将整数转换为字符串。使用起来非常简单,例如:
```cpp
#include <iostream>
#include <string>
int main() {
int num = 123;
std::string str_num = std::to_string(num);
std::cout << str_num << std::endl; // 输出: 123
return 0;
}
```
2. 使用`std::ostringstream`(输出流):
可以利用输出流将整数插入到字符串流中,然后再转换为字符串。这种方法在C++98中就已经存在,示例如下:
```cpp
#include <iostream>
#include <sstream>
#include <string>
int main() {
int num = 123;
std::ostringstream oss;
oss << num;
std::string str_num = oss.str();
std::cout << str_num << std::endl; // 输出: 123
return 0;
}
```
3. 使用`itoa`函数(非标准C++,仅限于特定环境):
在某些C++编译器中,如MSVC,还提供了`itoa`函数,它不是C++标准的一部分,因此它的可用性可能会受到限制。使用示例如下:
```cpp
#include <stdio.h>
int main() {
int num = 123;
char str_num[100];
itoa(num, str_num, 10); // 10表示十进制
printf("%s\n", str_num); // 输出: 123
return 0;
}
```
请注意,推荐使用`std::to_string`或`std::ostringstream`,因为它们是C++标准库提供的功能,更具有可移植性和类型安全性。
阅读全文
相关推荐
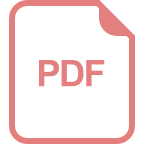
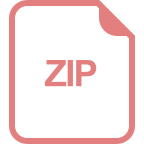
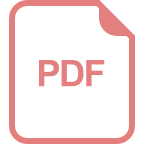



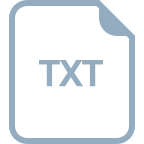
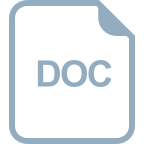
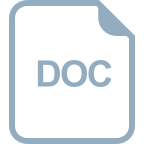









