词法分析器c语言编写
时间: 2023-07-02 20:08:28 浏览: 113
词法分析器是编译器的一个重要组成部分,它负责将源代码转换成单词流,为后续的语法分析和代码生成提供基础。下面是一个简单的词法分析器的C语言实现:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
#define MAXLEN 100 // 最大单词长度
// Token类型
typedef enum {
NONE, // 未定义
ID, // 标识符
INT, // 整数
PLUS, // 加号
MINUS, // 减号
TIMES, // 乘号
SLASH, // 除号
ASSIGN, // 赋值
SEMI // 分号
} TokenType;
// Token结构体
typedef struct {
TokenType type; // Token类型
char value[MAXLEN]; // Token值
} Token;
// 从文件中读取下一个字符
char getNextChar(FILE *fp) {
return fgetc(fp);
}
// 识别单词
TokenType recognizeToken(char *word) {
if (strcmp(word, "+") == 0) {
return PLUS;
} else if (strcmp(word, "-") == 0) {
return MINUS;
} else if (strcmp(word, "*") == 0) {
return TIMES;
} else if (strcmp(word, "/") == 0) {
return SLASH;
} else if (strcmp(word, "=") == 0) {
return ASSIGN;
} else if (strcmp(word, ";") == 0) {
return SEMI;
} else if (isdigit(word[0])) {
return INT;
} else {
return ID;
}
}
// 词法分析函数
Token getNextToken(FILE *fp) {
char ch;
char word[MAXLEN];
int i = 0;
Token token = {NONE, ""};
// 跳过空白字符
while (isspace(ch = getNextChar(fp))) ;
if (ch == EOF) {
token.type = NONE;
} else if (ch == '+' || ch == '-' || ch == '*' || ch == '/' || ch == '=' || ch == ';') {
// 识别运算符和分号
word[i++] = ch;
word[i] = '\0';
token.type = recognizeToken(word);
} else if (isdigit(ch)) {
// 识别整数
word[i++] = ch;
while (isdigit(ch = getNextChar(fp))) {
word[i++] = ch;
}
word[i] = '\0';
token.type = INT;
} else if (isalpha(ch)) {
// 识别标识符
word[i++] = ch;
while (isalnum(ch = getNextChar(fp))) {
word[i++] = ch;
}
word[i] = '\0';
token.type = ID;
} else {
token.type = NONE;
}
if (token.type != NONE) {
strcpy(token.value, word);
}
return token;
}
// 测试程序
int main() {
FILE *fp;
Token token;
fp = fopen("test.txt", "r");
if (fp == NULL) {
printf("文件打开失败\n");
return 1;
}
while ((token = getNextToken(fp)).type != NONE) {
printf("token: %d, value: %s\n", token.type, token.value);
}
fclose(fp);
return 0;
}
```
在上面的代码中,我们定义了一个 `Token` 结构体表示单词,其中包含单词类型和单词值两个字段。`TokenType` 枚举类型定义了常见的单词类型,如标识符、整数、运算符等。`getNextChar` 函数从文件中读取下一个字符,`recognizeToken` 函数根据单词的字符串值来识别单词类型。最后,`getNextToken` 函数根据读取到的字符序列来识别单词,并返回一个 `Token` 结构体。
在测试程序中,我们打开一个名为 `test.txt` 的文件,读取其中的单词并逐个输出其类型和值。注意,这里的文件中单词之间必须用空格或换行符分隔开,否则词法分析器无法正确读取单词。
阅读全文
相关推荐

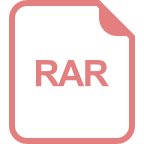














