PKCS8EncodedKeySpec priPKCS8 = new PKCS8EncodedKeySpec(Base64.getBytesBASE64(prikeyvalue)); KeyFactory keyf = KeyFactory.getInstance("RSA"); PrivateKey myprikey = keyf.generatePrivate(priPKCS8); // 用私钥对信息生成数字签名 java.security.Signature signet = java.security.Signature.getInstance("MD5withRSA"); signet.initSign(myprikey); signet.update(sign_str.getBytes(StandardCharsets.UTF_8)); byte[] signed = signet.sign(); // 对信息的数字签名 return new String(org.apache.commons.codec.binary.Base64.encodeBase64(signed)); 上面的代码用python怎么写?
时间: 2023-03-05 08:36:04 浏览: 131
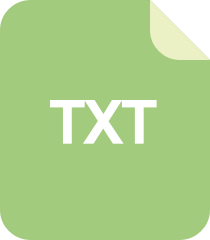
Java RSA私钥 pkcs1转pkcs8;

以下是使用 Python 实现上述 Java 代码的示例代码:
```python
import base64
from Crypto.PublicKey import RSA
from Crypto.Signature import PKCS1_v1_5
from Crypto.Hash import MD5
# 将 Base64 编码的私钥字符串解码为字节数组
prikeyvalue = base64.b64decode(prikeyvalue)
# 用 PKCS#8 格式解析私钥
pri_key = RSA.import_key(prikeyvalue)
# 用私钥对信息生成数字签名
hash_value = MD5.new(sign_str.encode('utf-8'))
signer = PKCS1_v1_5.new(pri_key)
signed = signer.sign(hash_value)
# 对信息的数字签名进行 Base64 编码并返回
return base64.b64encode(signed).decode('utf-8')
```
这里使用了 Python 中的 `Crypto` 库来实现数字签名。请确保在运行代码前先安装 `pycryptodome` 库,可以使用以下命令安装:
```bash
pip install pycryptodome
```
阅读全文
相关推荐
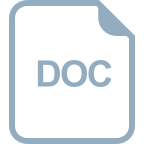
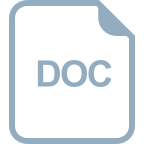















