贪吃蛇 python
时间: 2023-06-22 12:24:15 浏览: 55
好的,以下是一个简单的贪吃蛇游戏的 Python 代码:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 游戏区域的尺寸
width = 800
height = 600
# 创建游戏窗口
screen = pygame.display.set_mode((width, height))
# 设置游戏标题
pygame.display.set_caption("贪吃蛇")
# 定义颜色
white = (255, 255, 255)
black = (0, 0, 0)
red = (255, 0, 0)
green = (0, 255, 0)
# 蛇的初始位置
x = width / 2
y = height / 2
# 蛇的初始移动方向
direction = "right"
# 蛇的初始长度
snake_length = 1
# 蛇身体的坐标列表
snake_list = []
# 生成食物的随机坐标
food_x = round(random.randrange(0, width - 10) / 10.0) * 10.0
food_y = round(random.randrange(0, height - 10) / 10.0) * 10.0
# 游戏循环
game_over = False
while not game_over:
# 监听游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
direction = "left"
elif event.key == pygame.K_RIGHT:
direction = "right"
elif event.key == pygame.K_UP:
direction = "up"
elif event.key == pygame.K_DOWN:
direction = "down"
# 根据移动方向更新蛇的坐标
if direction == "right":
x += 10
elif direction == "left":
x -= 10
elif direction == "up":
y -= 10
elif direction == "down":
y += 10
# 清空游戏窗口
screen.fill(black)
# 绘制食物
pygame.draw.rect(screen, green, [food_x, food_y, 10, 10])
# 绘制蛇
snake_head = []
snake_head.append(x)
snake_head.append(y)
snake_list.append(snake_head)
if len(snake_list) > snake_length:
del snake_list[0]
for segment in snake_list[:-1]:
if segment == snake_head:
game_over = True
for segment in snake_list:
pygame.draw.rect(screen, white, [segment[0], segment[1], 10, 10])
# 如果蛇吃到了食物,长度加一,重新生成食物
if x == food_x and y == food_y:
snake_length += 1
food_x = round(random.randrange(0, width - 10) / 10.0) * 10.0
food_y = round(random.randrange(0, height - 10) / 10.0) * 10.0
# 更新游戏窗口
pygame.display.update()
# 控制游戏帧率
clock = pygame.time.Clock()
clock.tick(15)
# 退出游戏
pygame.quit()
quit()
```
这个游戏还比较简单,如果你想要实现更复杂的功能,可以参考 Pygame 的文档或者其他网上的教程。
相关推荐







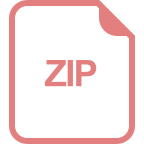