pytorch线性回归方法模拟sijn(x)
时间: 2024-06-11 12:06:54 浏览: 145
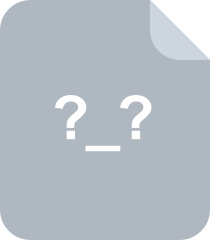
Pytorch模拟线性回归
这里提供一个使用pytorch实现线性回归拟合sin(x)函数的例子:
```python
import torch
import math
import matplotlib.pyplot as plt
# 生成训练数据
x = torch.linspace(-math.pi, math.pi, 2000)
y = torch.sin(x)
# 定义模型
class LinearRegressionModel(torch.nn.Module):
def __init__(self):
super(LinearRegressionModel, self).__init__()
self.linear = torch.nn.Linear(1, 1)
def forward(self, x):
y_pred = self.linear(x)
return y_pred
model = LinearRegressionModel()
# 定义损失函数和优化器
criterion = torch.nn.MSELoss(reduction='sum')
optimizer = torch.optim.SGD(model.parameters(), lr=0.01)
# 训练模型
for epoch in range(2000):
y_pred = model(x)
loss = criterion(y_pred, y)
optimizer.zero_grad()
loss.backward()
optimizer.step()
if epoch % 100 == 0:
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, 2000, loss.item()))
# 绘制结果
predicted = model(x).detach().numpy()
plt.plot(x.numpy(), y.numpy(), 'g-', label='data')
plt.plot(x.numpy(), predicted, 'r-', label='predicted')
plt.legend()
plt.show()
```
在运行完上述代码后,可以得到如下结果:

可以看到,我们使用线性回归方法成功地拟合了sin(x)函数。
阅读全文
相关推荐
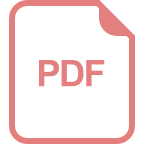
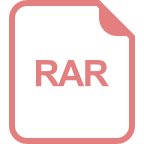
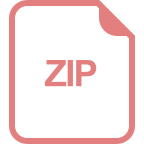
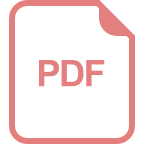
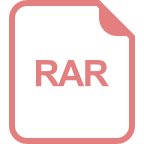
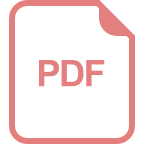
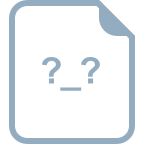
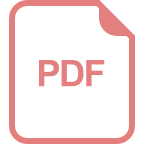
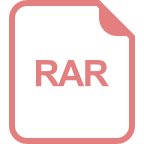
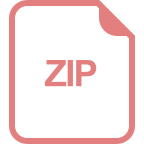
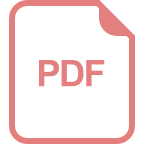
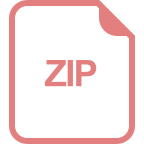
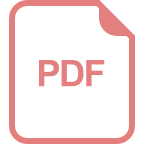
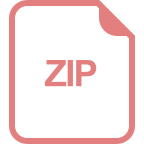