arduino使用1个按键、1个LED灯和1个喇叭,设计一个利用按键控制喇叭声响和灯亮灭的作品(英文名为:ButtonLedTone),具体要求如下: 按一次按键,灯亮;再按一次按键,灯灭;一直如此循环; 每次按下按键,喇叭都会响一下(约100毫秒),声音要清脆响亮; 要做按键去抖动功能,确保对按键状态变化的准确判断。
时间: 2023-04-09 18:03:46 浏览: 228
Sure, I can help you with that! Here's the code for the ButtonLedTone project using an Arduino board:
```
int ledPin = 13; // LED connected to digital pin 13
int speakerPin = 8; // Speaker connected to digital pin 8
int buttonPin = 2; // Button connected to digital pin 2
int buttonState = 0; // variable for storing button state
int lastButtonState = 0; // variable for storing last button state
int toneDuration = 100; // duration of tone in milliseconds
void setup() {
pinMode(ledPin, OUTPUT); // set LED pin as output
pinMode(speakerPin, OUTPUT); // set speaker pin as output
pinMode(buttonPin, INPUT); // set button pin as input
}
void loop() {
buttonState = digitalRead(buttonPin); // read button state
if (buttonState != lastButtonState) { // check for button state change
delay(10); // debounce delay
buttonState = digitalRead(buttonPin); // read button state again
if (buttonState == HIGH) { // button pressed
digitalWrite(ledPin, !digitalRead(ledPin)); // toggle LED state
tone(speakerPin, 1000, toneDuration); // play tone
}
}
lastButtonState = buttonState; // store last button state
}
```
This code uses the `digitalRead()` function to read the state of the button connected to pin 2. It also uses the `digitalWrite()` function to toggle the state of the LED connected to pin 13 and the `tone()` function to play a tone on the speaker connected to pin 8. The `delay()` function is used to debounce the button and ensure accurate state change detection.
I hope this helps! Let me know if you have any other questions.
阅读全文
相关推荐








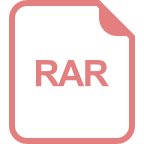






