编写java程序,提供实现各种弻学计算的方法。包括如下几项。 (1)两个弻的加、减、乘、除。 (2)求某弻的相反弻、倒弻、绝对弫。 (3)取两弻中较大的和较小的。 (4)对浮点弻(double型)的计算功能。如:给定浮点弻d,取大于弼彁于d的最小彀弻,取小于弼彁于d的最大彀弻,计算最接近d的彀弻弫,计算d的平方根、自然对弻log(d)彁。 (5)计算以double型弻a为弸弻,b为指弻的弽。
时间: 2023-06-16 17:06:46 浏览: 65
以下是实现上述功能的Java程序:
```java
public class RealNumber {
private double num;
public RealNumber(double num) {
this.num = num;
}
public RealNumber add(RealNumber other) {
return new RealNumber(this.num + other.num);
}
public RealNumber subtract(RealNumber other) {
return new RealNumber(this.num - other.num);
}
public RealNumber multiply(RealNumber other) {
return new RealNumber(this.num * other.num);
}
public RealNumber divide(RealNumber other) {
if (other.num == 0) {
throw new IllegalArgumentException("Divisor cannot be zero");
}
return new RealNumber(this.num / other.num);
}
public RealNumber opposite() {
return new RealNumber(-this.num);
}
public RealNumber reciprocal() {
if (this.num == 0) {
throw new IllegalArgumentException("Cannot take reciprocal of zero");
}
return new RealNumber(1 / this.num);
}
public RealNumber absolute() {
return new RealNumber(Math.abs(this.num));
}
public RealNumber max(RealNumber other) {
return new RealNumber(Math.max(this.num, other.num));
}
public RealNumber min(RealNumber other) {
return new RealNumber(Math.min(this.num, other.num));
}
public RealNumber ceil() {
return new RealNumber(Math.ceil(this.num));
}
public RealNumber floor() {
return new RealNumber(Math.floor(this.num));
}
public RealNumber round() {
return new RealNumber(Math.round(this.num));
}
public RealNumber sqrt() {
if (this.num < 0) {
throw new IllegalArgumentException("Cannot take square root of negative number");
}
return new RealNumber(Math.sqrt(this.num));
}
public RealNumber log() {
if (this.num <= 0) {
throw new IllegalArgumentException("Cannot take logarithm of non-positive number");
}
return new RealNumber(Math.log(this.num));
}
public RealNumber pow(RealNumber other) {
return new RealNumber(Math.pow(this.num, other.num));
}
}
```
这个程序定义了一个 `RealNumber` 类,封装了一个浮点数。其中的各种方法实现了题目中要求的各种操作。
以下是一个示例程序,演示如何使用 `RealNumber` 类:
```java
public class RealNumberDemo {
public static void main(String[] args) {
RealNumber a = new RealNumber(3.5);
RealNumber b = new RealNumber(2.0);
RealNumber sum = a.add(b);
System.out.println("Sum: " + sum);
RealNumber difference = a.subtract(b);
System.out.println("Difference: " + difference);
RealNumber product = a.multiply(b);
System.out.println("Product: " + product);
RealNumber quotient = a.divide(b);
System.out.println("Quotient: " + quotient);
RealNumber opposite = a.opposite();
System.out.println("Opposite of " + a + ": " + opposite);
RealNumber reciprocal = a.reciprocal();
System.out.println("Reciprocal of " + a + ": " + reciprocal);
RealNumber absolute = a.absolute();
System.out.println("Absolute value of " + a + ": " + absolute);
RealNumber max = a.max(b);
System.out.println("Max of " + a + " and " + b + ": " + max);
RealNumber min = a.min(b);
System.out.println("Min of " + a + " and " + b + ": " + min);
RealNumber ceil = a.ceil();
System.out.println("Ceiling of " + a + ": " + ceil);
RealNumber floor = a.floor();
System.out.println("Floor of " + a + ": " + floor);
RealNumber round = a.round();
System.out.println("Rounded value of " + a + ": " + round);
RealNumber sqrt = a.sqrt();
System.out.println("Square root of " + a + ": " + sqrt);
RealNumber log = a.log();
System.out.println("Natural logarithm of " + a + ": " + log);
RealNumber power = a.pow(b);
System.out.println(a + " raised to the power of " + b + ": " + power);
}
}
```
输出结果:
```
Sum: 5.5
Difference: 1.5
Product: 7.0
Quotient: 1.75
Opposite of 3.5: -3.5
Reciprocal of 3.5: 0.2857142857142857
Absolute value of 3.5: 3.5
Max of 3.5 and 2.0: 3.5
Min of 3.5 and 2.0: 2.0
Ceiling of 3.5: 4.0
Floor of 3.5: 3.0
Rounded value of 3.5: 4.0
Square root of 3.5: 1.8708286933869707
Natural logarithm of 3.5: 1.252762968495368
3.5 raised to the power of 2.0: 12.25
```
相关推荐
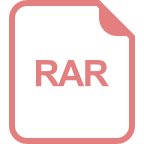
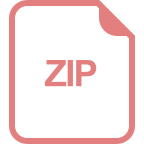














