如何使用Python实现SHA256withRSA算法进行消息签名和验证,以及如何结合RSA(例如ECB模式)和PKCS1Padding来处理敏感信息的加密与解密过程?
时间: 2024-12-10 17:37:03 浏览: 26
在Python中,可以使用第三方库如`cryptography`来实现SHA256withRSA算法的消息签名和验证,以及RSA和PKCS#1 padding的加密和解密。以下是基本步骤:
1. 安装`cryptography`库:
```bash
pip install cryptography
```
2. 导入所需模块并生成密钥对(公钥用于加密,私钥用于解密和签名):
```python
from cryptography.hazmat.primitives import serialization, hashes
from cryptography.hazmat.primitives.asymmetric import rsa, padding
# 生成RSA密钥对
private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048
)
public_key = private_key.public_key()
```
3. 对数据进行哈希(这里使用SHA256):
```python
data_to_sign = "your message"
hash_object = hashes.Hash(hashes.SHA256())
hash_object.update(data_to_sign.encode('utf-8'))
digest = hash_object.finalize()
```
4. 签名数据(使用私钥):
```python
signature = private_key.sign(digest, padding.PSS(mgf=padding.MGF1(hashes.SHA256()), salt_length=padding.PSS.MAX_LENGTH))
```
5. 验证签名(使用公钥):
```python
try:
public_key.verify(signature, digest, padding.PSS(mgf=padding.MGF1(hashes.SHA256()), salt_length=padding.PSS.MAX_LENGTH))
print("Signature is valid")
except (ValueError, InvalidSignature):
print("Signature is invalid")
```
对于RSA加PKCS1Padding的加密与解密:
```python
# 加密(使用公钥和PKCS1v1_5 Padding)
plaintext = b"Sensitive data"
cipher_text = public_key.encrypt(plaintext, padding.PKCS1v15())
# 解密(使用私钥)
decrypted_text = private_key.decrypt(cipher_text)
```
在这个例子中,我们使用了ECB(Electronic Codebook)模式作为加密模式,但它通常不适合长期存储敏感信息,因为ECB会将相同的明文块加密成相同的密文块。如果你需要更安全的方式,可以考虑使用CBC(Cipher Block Chaining)或者其他模式,比如AES。
阅读全文
相关推荐
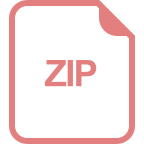
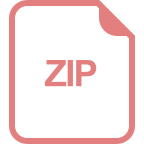
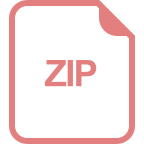
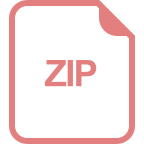
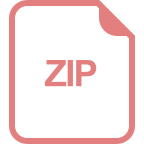
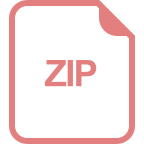
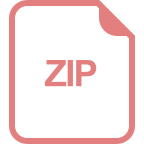
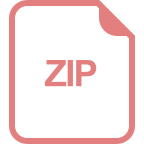
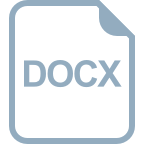