from torchvision.models import densenet121
时间: 2024-01-03 12:02:08 浏览: 38
from torchvision.models import densenet121是一个用于深度学习的Python库,它提供了一个名为densenet121的预训练模型,该模型能够用于图像分类任务。在导入这个模型之后,我们可以利用它来进行图像识别和分类工作。densenet121是一个基于深度残差网络(DenseNet)的模型,它的设计采用了密集连接(dense connectivity)和过渡块(transition block)这两个重要的结构,使得模型在提取特征时更加高效和准确。
使用densenet121模型的过程可以分为几个步骤。首先,我们需要导入densenet121模型,然后加载预训练的权重参数。接着,我们可以将待分类的图像输入到模型中,通过前向传播的方式得到模型对图像的分类结果。最后,根据模型输出的结果来完成具体的图像分类任务。
除了图像分类之外,densenet121模型还可以用于迁移学习,即将其在其他数据集上训练好的权重参数应用到新的任务中,从而加快模型的训练速度并提高模型的性能。
总之,from torchvision.models import densenet121提供了一个方便快捷的方式来使用densenet121模型进行图像分类和迁移学习,为深度学习任务提供了更多的选择和可能性。
相关问题
import cv2 import numpy as np import torch as torch from torchvision.models import densenet121 # Load the DenseNet model model = densenet121(pretrained=True) # Read the image image = cv2.imread('C:/Users/23594/Desktop/888.jpg') # Convert the image to grayscale grayscale_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) # Resize the image to the size of the model input resized_image = cv2.resize(grayscale_image, (224, 224)) # Normalize the image normalized_image = resized_image / 255.0 # Convert the image to a tensor image_tensor = torch.from_numpy(normalized_image).float() # Predict the key points of the person predictions = model(image_tensor) # Convert the predictions to a list of points points = [] for i in range(len(predictions[0])): points.append((predictions[0][i][0], predictions[0][i][1])) # Draw the key points on the image cv2.drawKeypoints(image, points, np.array([]), (0, 255, 0), flags=cv2.DRAW_MATCHES_FLAGS_DRAW_RICH_KEYPOINTS) # Display the image cv2.imshow('Image', image) cv2.waitKey(0)import cv2 import numpy as np import torch as torch from torchvision.models import densenet121 # Load the DenseNet model model = densenet121(pretrained=True) # Read the image image = cv2.imread('C:/Users/23594/Desktop/888.jpg') # Convert the image to grayscale grayscale_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) # Resize the image to the size of the model input resized_image = cv2.resize(grayscale_image, (224, 224)) # Normalize the image normalized_image = resized_image / 255.0 # Convert the image to a tensor image_tensor = torch.from_numpy(normalized_image).float() # Predict the key points of the person predictions = model(image_tensor) # Convert the predictions to a list of points points = [] for i in range(len(predictions[0])): points.append((predictions[0][i][0], predictions[0][i][1])) # Draw the key points on the image cv2.drawKeypoints(image, points, np.array([]), (0, 255, 0), flags=cv2.DRAW_MATCHES_FLAGS_DRAW_RICH_KEYPOINTS) # Display the image cv2.imshow('Image', image) cv2.waitKey(0)
这段代码是使用预训练的DenseNet模型对一张人物图片进行关键点检测,并在图片上标出关键点。它使用了OpenCV和PyTorch库。具体流程包括:
1. 导入必要的库,包括OpenCV、NumPy和PyTorch等。
2. 加载预训练的DenseNet模型。
3. 读取待检测的人物图片,并将其转为灰度图像。
4. 调整图片大小为与模型输入大小相同。
5. 将像素值归一化到0到1之间,并将其转为PyTorch的张量。
6. 对张量进行预测,得到关键点的坐标。
7. 将预测结果转为关键点的列表。
8. 在原始图片上标出关键点。
9. 显示标注后的图片。
需要注意的是,这段代码没有进行任何异常处理和参数调优,仅仅是演示了一个基本的关键点检测流程。
densenet121实现图片大小为48×48×3四分类代码
以下是使用PyTorch实现densenet121进行48x48x3图像四分类的示例代码:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import torchvision.transforms as transforms
from torch.utils.data import DataLoader
from torchvision.datasets import ImageFolder
from torchvision.models import densenet121
# 定义超参数
batch_size = 32
learning_rate = 0.001
num_epochs = 10
# 数据预处理
transform = transforms.Compose([
transforms.Resize(48),
transforms.CenterCrop(48),
transforms.ToTensor(),
transforms.Normalize(mean=[0.5, 0.5, 0.5], std=[0.5, 0.5, 0.5])
])
# 加载数据集
train_dataset = ImageFolder(root="./train", transform=transform)
train_loader = DataLoader(train_dataset, batch_size=batch_size, shuffle=True)
val_dataset = ImageFolder(root="./val", transform=transform)
val_loader = DataLoader(val_dataset, batch_size=batch_size, shuffle=False)
test_dataset = ImageFolder(root="./test", transform=transform)
test_loader = DataLoader(test_dataset, batch_size=batch_size, shuffle=False)
# 定义模型
model = densenet121(pretrained=True)
num_ftrs = model.classifier.in_features
model.classifier = nn.Linear(num_ftrs, 4) # 四分类问题,输出节点数为4
# 定义损失函数和优化器
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=learning_rate)
# 训练模型
for epoch in range(num_epochs):
model.train()
for images, labels in train_loader:
optimizer.zero_grad()
outputs = model(images)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
# 在验证集上测试模型性能
model.eval()
with torch.no_grad():
correct = 0
total = 0
for images, labels in val_loader:
outputs = model(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
val_acc = correct / total
print("Epoch {}, Validation Accuracy: {:.2f}%".format(epoch+1, val_acc*100))
# 在测试集上测试模型性能
model.eval()
with torch.no_grad():
correct = 0
total = 0
for images, labels in test_loader:
outputs = model(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
test_acc = correct / total
print("Test Accuracy: {:.2f}%".format(test_acc*100))
```
在上述代码中,我们使用ImageFolder类从文件夹中加载图像数据集,并使用DataLoader类进行批量读取。我们使用预训练的densenet121模型,将其全连接层的输出节点数修改为4。使用交叉熵损失函数进行训练,使用Adam优化器进行参数更新。在每个epoch结束时,我们在验证集上测试模型性能,并输出验证集准确率。最后,在测试集上测试模型性能并输出测试集准确率。
相关推荐
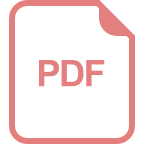













