用opencv提取迷宫中点的坐标
时间: 2023-11-14 13:57:46 浏览: 164
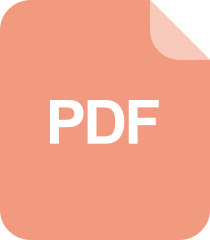
使用opencv中匹配点对的坐标提取方式
提取迷宫中点的坐标可以通过以下步骤实现:
1. 读取迷宫图像并将其转换为灰度图像。
```python
import cv2
img = cv2.imread("maze.png")
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
2. 对灰度图像进行二值化处理,将迷宫的墙壁和路径分离出来。
```python
thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)[1]
```
3. 对二值化图像进行边缘检测,提取迷宫路径的轮廓。
```python
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
```
4. 对路径轮廓进行筛选,去除长度较小的轮廓。
```python
contours_filtered = []
for contour in contours:
if len(contour) > 50:
contours_filtered.append(contour)
```
5. 对筛选后的轮廓进行近似处理,得到轮廓的多边形拟合。
```python
polygons = []
for contour in contours_filtered:
epsilon = 0.03 * cv2.arcLength(contour, True)
polygon = cv2.approxPolyDP(contour, epsilon, True)
polygons.append(polygon)
```
6. 对多边形拟合进行筛选,得到迷宫路径上的关键点。
```python
keypoints = []
for polygon in polygons:
for point in polygon:
keypoints.append((point[0][0], point[0][1]))
```
7. 将关键点按照 x 坐标排序,然后按照 y 坐标排序,得到迷宫路径上的中心点坐标。
```python
keypoints_sorted_x = sorted(keypoints, key=lambda x: x[0])
keypoints_sorted_y = sorted(keypoints_sorted_x, key=lambda x: x[1])
centers = []
for i in range(1, len(keypoints_sorted_y)):
center = ((keypoints_sorted_y[i][0] + keypoints_sorted_y[i-1][0]) // 2, (keypoints_sorted_y[i][1] + keypoints_sorted_y[i-1][1]) // 2)
centers.append(center)
```
完整代码如下:
```python
import cv2
img = cv2.imread("maze.png")
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)[1]
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
contours_filtered = []
for contour in contours:
if len(contour) > 50:
contours_filtered.append(contour)
polygons = []
for contour in contours_filtered:
epsilon = 0.03 * cv2.arcLength(contour, True)
polygon = cv2.approxPolyDP(contour, epsilon, True)
polygons.append(polygon)
keypoints = []
for polygon in polygons:
for point in polygon:
keypoints.append((point[0][0], point[0][1]))
keypoints_sorted_x = sorted(keypoints, key=lambda x: x[0])
keypoints_sorted_y = sorted(keypoints_sorted_x, key=lambda x: x[1])
centers = []
for i in range(1, len(keypoints_sorted_y)):
center = ((keypoints_sorted_y[i][0] + keypoints_sorted_y[i-1][0]) // 2, (keypoints_sorted_y[i][1] + keypoints_sorted_y[i-1][1]) // 2)
centers.append(center)
for center in centers:
cv2.circle(img, center, 5, (0, 255, 0), -1)
cv2.imshow("result", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐
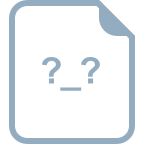
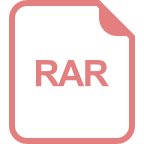
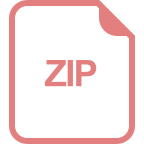
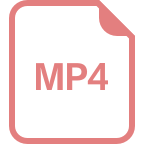
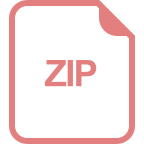
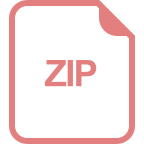
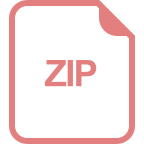
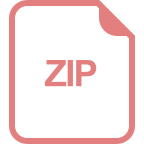
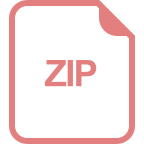