vue中在js内改table表格的样式
时间: 2024-05-09 21:20:00 浏览: 121
可以使用以下方法在Vue的JavaScript代码中改变表格的样式:
1. 通过class或id选择器获取表格元素,例如:
```
<template>
<table id="myTable">
...
</table>
</template>
<script>
export default {
mounted() {
const table = document.getElementById('myTable');
table.style.backgroundColor = 'lightblue';
table.style.color = 'white';
}
}
</script>
```
2. 使用Vue的ref属性来获取表格元素,例如:
```
<template>
<table ref="myTable">
...
</table>
</template>
<script>
export default {
mounted() {
const table = this.$refs.myTable;
table.style.backgroundColor = 'lightblue';
table.style.color = 'white';
}
}
</script>
```
注意:直接在JavaScript代码中修改样式可能会影响组件的可维护性和易用性,最好在CSS文件中定义好样式,然后在Vue组件中应用CSS类来改变表格的样式。
相关问题
vue中在js内改el-table表格的样式
可以通过以下几种方式在js中改变el-table表格的样式:
1. 使用CSS样式:可以在CSS文件中定义样式,然后通过给el-table组件添加class属性来应用样式。示例代码如下:
```css
.my-table {
background-color: #f2f2f2;
border: 1px solid #ccc;
}
```
```html
<el-table :data="tableData" class="my-table">
<!-- table columns -->
</el-table>
```
2. 直接在JS中设置样式:可以通过给el-table组件添加style属性来直接在JS中设置样式。示例代码如下:
```html
<el-table :data="tableData" :style="{backgroundColor:'#f2f2f2',border:'1px solid #ccc'}">
<!-- table columns -->
</el-table>
```
3. 使用scoped slot:可以使用scoped slot来自定义表格的样式。示例代码如下:
```html
<el-table :data="tableData">
<template slot-scope="{row}">
<tr :style="{background: row.id % 2 === 0 ? '#f2f2f2' : ''}">
<td>{{ row.name }}</td>
<td>{{ row.age }}</td>
</tr>
</template>
</el-table>
```
上述代码中,使用了scoped slot来自定义每一行的样式,根据行的id来设置不同的背景色。
vue中如何更改el-table表格背景色
在 Vue 中,你可以通过自定义 CSS 或者通过 el-table 的属性来更改表格背景色。
方法一:使用自定义 CSS
1. 在你的组件样式中,添加一个自定义的类名,例如 `myTable`:
```css
.myTable {
background-color: #f0f0f0;
}
```
2. 在 el-table 标签上添加 `class` 属性,并将自定义的类名添加到其中:
```html
<el-table class="myTable">
<!-- 表格内容 -->
</el-table>
```
这样就可以将表格的背景色设置为 `#f0f0f0`。
方法二:使用 el-table 的属性
el-table 组件提供了一些可以用来自定义样式的属性,其中包括 `row-class-name` 属性。
1. 在你的组件中,定义一个方法来设置每一行的类名:
```javascript
methods: {
rowClassName({ row }) {
// 根据条件返回不同的类名
if (row.someCondition) {
return 'myRowClass';
} else {
return 'defaultRowClass';
}
},
}
```
2. 在 el-table 标签上添加 `row-class-name` 属性,并将方法名作为属性值传入:
```html
<el-table :row-class-name="rowClassName">
<!-- 表格内容 -->
</el-table>
```
在 `rowClassName` 方法中,你可以根据不同的条件返回不同的类名,然后通过自定义 CSS 来设置相应的背景色。
希望以上两种方法能够帮助到你!
阅读全文
相关推荐
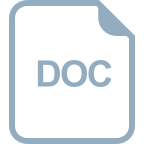
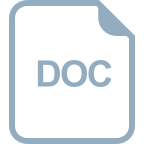
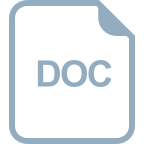
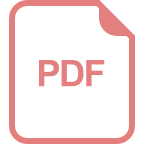
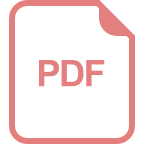
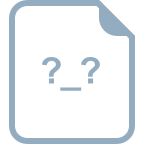
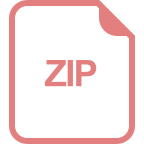









