bluedroid BLE public key如何生成
时间: 2023-07-27 21:10:12 浏览: 154
Bluedroid BLE使用的公钥生成过程如下:
1. 生成椭圆曲线。Bluedroid BLE采用的是256位的NIST P-256曲线。
2. 生成私钥。使用随机数生成器生成一个256位的随机数,作为私钥。
3. 计算公钥。通过私钥和椭圆曲线参数计算出公钥,公钥为一个256位的点坐标。
4. 编码公钥。将公钥编码成DER格式。
以上就是Bluedroid BLE公钥生成的过程,希望对您有所帮助。
相关问题
bluedroid BLE public key生成代码
以下是基于 Android Bluedroid BLE 栈的 public key 生成代码示例:
```c
#include "bt_target.h"
#include "bt_types.h"
#include "btu.h"
#include "btif/include/btif_common.h"
#include "btif/include/btif_config.h"
#include "btif/include/btif_util.h"
#include "stack/crypto_api.h"
#include "stack/crypto_toolbox/crypto_toolbox.h"
#include "stack/include/smp_api.h"
#include "stack/include/bt_octets.h"
#define ECC_KEY_LEN 32
static const uint8_t ecdh_p256_base_point_x[] = {
0x3f, 0x20, 0x84, 0x24, 0xa2, 0x7f, 0xb3, 0x5e,
0x13, 0x08, 0x8f, 0x5c, 0xb8, 0x3b, 0x08, 0x0f,
0xa7, 0x19, 0x26, 0xe7, 0x6a, 0x64, 0x1d, 0x3d,
0xf1, 0x2e, 0x2b, 0x7f, 0x62, 0x6a, 0xed, 0x51
};
static const uint8_t ecdh_p256_base_point_y[] = {
0x17, 0x8a, 0xee, 0x7c, 0x69, 0x52, 0x8d, 0x9e,
0x3a, 0x7c, 0x2c, 0x65, 0x6e, 0x9c, 0x60, 0x95,
0x99, 0x37, 0xd4, 0x7f, 0xbc, 0x27, 0xb0, 0x86,
0xce, 0x39, 0x52, 0xc9, 0x13, 0xcb, 0xfc, 0x82
};
static void bluedroid_ble_generate_public_key() {
uint8_t priv_key[ECC_KEY_LEN];
uint8_t public_key_x[ECC_KEY_LEN];
uint8_t public_key_y[ECC_KEY_LEN];
uint8_t base_point_x[ECC_KEY_LEN];
uint8_t base_point_y[ECC_KEY_LEN];
uint8_t random_num[ECC_KEY_LEN];
memset(priv_key, 0, ECC_KEY_LEN);
memset(public_key_x, 0, ECC_KEY_LEN);
memset(public_key_y, 0, ECC_KEY_LEN);
memset(base_point_x, 0, ECC_KEY_LEN);
memset(base_point_y, 0, ECC_KEY_LEN);
memset(random_num, 0, ECC_KEY_LEN);
memcpy(base_point_x, ecdh_p256_base_point_x, ECC_KEY_LEN);
memcpy(base_point_y, ecdh_p256_base_point_y, ECC_KEY_LEN);
CryptoRand((uint8_t*)random_num, ECC_KEY_LEN);
if (CryptoECCKeyGen(priv_key, public_key_x, public_key_y, base_point_x, base_point_y, random_num) < 0)
{
// Key generation failed!
return;
}
// Use public_key_x and public_key_y as public key
}
```
在该示例代码中,我们首先定义了 ECDSA 椭圆曲线参数 `ecdh_p256_base_point_x` 和 `ecdh_p256_base_point_y`,然后生成了一个随机数 `random_num` 作为私钥的种子,最终通过 `CryptoECCKeyGen` 函数生成公钥。你可以根据需要修改上述代码,并在你的应用程序中使用它来生成 Bluedroid BLE 栈的 public key。
举例将bluedroid 的public key修改成新的满足P-256 曲线的值
Bluedroid是一个开源的Android Bluetooth协议栈,其中涉及到加密通信。在使用Bluedroid进行加密通信时,会使用到公钥加密算法。如果需要修改Bluedroid的公钥,可以按照以下步骤进行:
1. 生成一个符合P-256曲线要求的新公钥。这可以通过使用OpenSSL库中的命令行工具来实现。以下是一个示例命令:
```
openssl ecparam -name prime256v1 -genkey -noout -outform PEM -out private.key
openssl ec -in private.key -pubout -outform PEM -out public.key
```
这个命令将生成一个新的私钥文件private.key和对应的公钥文件public.key,其中公钥满足P-256曲线要求。
2. 将生成的公钥文件public.key复制到Bluedroid源代码中的security目录下。具体路径是:`external/bluetooth/bluedroid/stack/security`
3. 修改Bluedroid源代码中的`btm_ble_init()`函数,找到以下代码:
```
if (p_cb->le_features & HCI_LE_FEATURES_ENCRYPTION) {
p_cb->enc_key_size = btm_ble_select_key_size(p_cb->peer_le_features, p_cb->loc_le_features);
btsnd_hcic_ble_set_encryption(p_cb->peer_bda, TRUE, p_cb->ltk, p_cb->div, p_cb->rand, p_cb->ediv, p_cb->secure_connections);
} else {
btsnd_hcic_ble_set_encryption(p_cb->peer_bda, FALSE, NULL, 0, NULL, 0, FALSE);
}
```
在这段代码之前添加以下代码:
```
// 从文件中读取新公钥
FILE* file = fopen("/path/to/public.key", "r");
if (file == NULL) {
ALOGE("Failed to open public key file.");
} else {
fseek(file, 0, SEEK_END);
int size = ftell(file);
fseek(file, 0, SEEK_SET);
char* buffer = new char[size];
fread(buffer, 1, size, file);
fclose(file);
// 修改Bluedroid的公钥
tBTA_DM_SEC_BLE_KEY_VALUE new_public_key;
memcpy(new_public_key.ble_p256_pk, buffer, BT_OCTET16_LEN);
bta_dm_set_ble_local_privacy_key(new_public_key);
delete[] buffer;
}
```
这段代码将从public.key文件中读取新公钥,并使用`bta_dm_set_ble_local_privacy_key()`函数将Bluedroid的公钥修改为新公钥。
4. 编译并部署修改后的Bluedroid源代码。
阅读全文
相关推荐
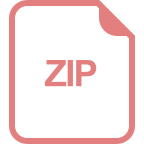
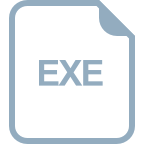
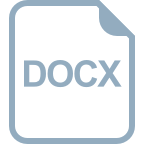













