页面有多个dialog弹框,如何通过继承或组合等方式,将相似的弹框进行抽象和重用,请详细解说,请附上el-dialog 代码等案例,需要非常详细
时间: 2023-05-14 20:05:03 浏览: 116
好的,这是一个很好的编程问题。在处理多个相似的弹框时,我们可以使用继承或组合等方式来进行抽象和重用。下面是一些可能的解决方案:
1. 继承方式
我们可以创建一个基础的弹框类,然后通过继承来创建其他相似的弹框。这样,我们可以在基础类中定义通用的属性和方法,然后在子类中重写或扩展这些属性和方法。
例如,我们可以创建一个基础的 Dialog 类,它包含了所有弹框都需要的属性和方法,如标题、内容、确定和取消按钮等。然后,我们可以创建其他子类,如 ConfirmDialog、AlertDialog 等,它们都继承自 Dialog 类,并且可以重写或扩展一些属性和方法。
下面是一个简单的示例代码:
```html
<template>
<el-dialog :title="title" :visible.sync="visible" :close-on-click-modal="false">
<div v-html="content"></div>
<span slot="footer">
<el-button @click="handleCancel">取消</el-button>
<el-button type="primary" @click="handleOk">确定</el-button>
</span>
</el-dialog>
</template>
<script>
export default {
props: {
title: String,
content: String,
visible: Boolean
},
methods: {
handleOk() {
this.$emit('ok')
},
handleCancel() {
this.$emit('cancel')
}
}
}
</script>
```
2. 组合方式
除了继承,我们还可以使用组合方式来重用相似的弹框。组合方式是指将多个组件组合在一起,形成一个新的组件。这样,我们可以将多个相似的弹框组合成一个新的弹框组件,然后在需要使用的地方直接引用这个组件。
例如,我们可以创建一个 DialogWrapper 组件,它包含了所有弹框都需要的属性和方法,如标题、内容、确定和取消按钮等。然后,我们可以创建其他子组件,如 ConfirmDialog、AlertDialog 等,它们都包含了自己的特定内容和逻辑。最后,我们可以将这些子组件组合在一起,形成一个新的弹框组件。
下面是一个简单的示例代码:
```html
<template>
<el-dialog :title="title" :visible.sync="visible" :close-on-click-modal="false">
<slot></slot>
<span slot="footer">
<el-button @click="handleCancel">取消</el-button>
<el-button type="primary" @click="handleOk">确定</el-button>
</span>
</el-dialog>
</template>
<script>
export default {
props: {
title: String,
visible: Boolean
},
methods: {
handleOk() {
this.$emit('ok')
},
handleCancel() {
this.$emit('cancel')
}
}
}
</script>
```
```html
<template>
<dialog-wrapper :title="title" :visible.sync="visible">
<div v-html="content"></div>
</dialog-wrapper>
</template>
<script>
import DialogWrapper from './DialogWrapper.vue'
export default {
components: {
DialogWrapper
},
props: {
title: String,
content: String,
visible: Boolean
}
}
</script>
```
以上是两种常见的解决方案,具体的实现方式可以根据实际情况进行调整。希望能对你有所帮助!
阅读全文
相关推荐
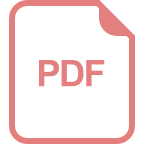
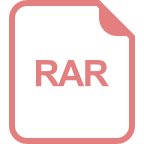
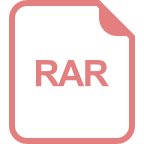
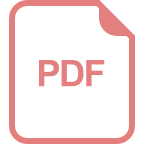
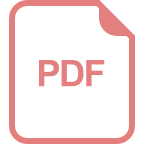
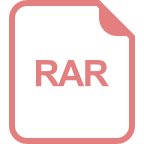
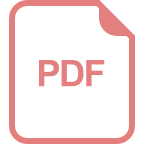
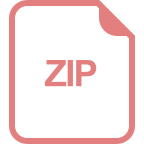
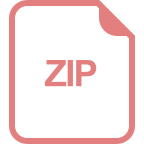
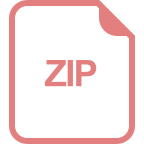
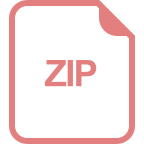
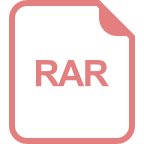
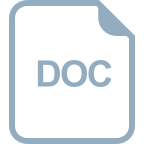