实现一个名为stock基类以及一个stock的子类mystock,在mystock实例调用stock类中的方法和属性代码
时间: 2024-04-28 21:26:02 浏览: 125
好的,这是一个实现上述需求的示例代码:
```python
class Stock:
def __init__(self, symbol, name):
self.symbol = symbol
self.name = name
self.price = 0.0
self.change = 0.0
def set_price(self, price):
self.price = price
def set_change(self, change):
self.change = change
def print_info(self):
print(f"{self.symbol} ({self.name}): {self.price}, {self.change}%")
class MyStock(Stock):
def __init__(self, symbol, name, quantity):
super().__init__(symbol, name)
self.quantity = quantity
def buy(self, amount):
print(f"Bought {amount} shares of {self.symbol}")
self.quantity += amount
def sell(self, amount):
print(f"Sold {amount} shares of {self.symbol}")
self.quantity -= amount
def print_info(self):
super().print_info()
print(f"Quantity: {self.quantity}")
```
在上面的代码中,我们先定义了一个名为`Stock`的基类,它有`symbol`、`name`、`price`和`change`四个属性,以及`set_price()`、`set_change()`和`print_info()`三个方法。
然后我们定义了一个名为`MyStock`的子类,它继承自`Stock`,并新增了一个`quantity`属性和`buy()`、`sell()`两个方法,用于购买和出售股票,并根据交易量更新`quantity`属性。
`MyStock`类还覆盖了`print_info()`方法,通过调用`super()`函数先调用父类的`print_info()`方法,再打印`quantity`属性的值。
现在我们可以创建一个`MyStock`的实例,并调用基类`Stock`中的方法和属性:
```python
mystock = MyStock("AAPL", "Apple Inc.", 100)
mystock.set_price(150.0)
mystock.set_change(1.5)
mystock.buy(50)
mystock.sell(25)
mystock.print_info()
```
输出结果为:
```
Bought 50 shares of AAPL
Sold 25 shares of AAPL
AAPL (Apple Inc.): 150.0, 1.5%
Quantity: 125
```
我们可以看到,`MyStock`类成功地调用了`Stock`类中的`set_price()`、`set_change()`和`print_info()`方法,并且在基础上新增了自己的方法和属性。
阅读全文
相关推荐





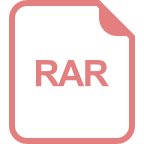










