vue 多视频时 如何截取视频的第一帧做为封面
时间: 2023-11-24 19:06:00 浏览: 36
要截取视频的第一帧做为封面,可以使用 HTML5 的 video 元素和 canvas 元素来实现。
首先,在 Vue 的组件中,可以使用 video 元素来加载视频:
```html
<video ref="myVideo" src="your-video-url"></video>
```
然后,在 mounted 钩子函数中,可以监听 video 元素的 loadedmetadata 事件,获取视频的宽高信息以及视频播放时长:
```javascript
mounted() {
this.$refs.myVideo.addEventListener('loadedmetadata', this.handleVideoLoaded)
},
methods: {
handleVideoLoaded() {
const video = this.$refs.myVideo
this.videoWidth = video.videoWidth
this.videoHeight = video.videoHeight
this.videoDuration = video.duration
}
}
```
接下来,可以使用 canvas 元素来截取视频的第一帧作为封面:
```javascript
const canvas = document.createElement('canvas')
canvas.width = this.videoWidth
canvas.height = this.videoHeight
const ctx = canvas.getContext('2d')
ctx.drawImage(this.$refs.myVideo, 0, 0, this.videoWidth, this.videoHeight)
const coverUrl = canvas.toDataURL()
```
最后,将封面图片的 URL 赋值给组件的 data 中的 coverUrl 属性,就可以在页面中显示视频的封面了:
```javascript
this.coverUrl = coverUrl
```
完整的代码如下:
```html
<template>
<div>
<video ref="myVideo" src="your-video-url"></video>
<img :src="coverUrl" alt="video-cover">
</div>
</template>
<script>
export default {
data() {
return {
videoWidth: 0,
videoHeight: 0,
videoDuration: 0,
coverUrl: ''
}
},
mounted() {
this.$refs.myVideo.addEventListener('loadedmetadata', this.handleVideoLoaded)
},
methods: {
handleVideoLoaded() {
const video = this.$refs.myVideo
this.videoWidth = video.videoWidth
this.videoHeight = video.videoHeight
this.videoDuration = video.duration
const canvas = document.createElement('canvas')
canvas.width = this.videoWidth
canvas.height = this.videoHeight
const ctx = canvas.getContext('2d')
ctx.drawImage(this.$refs.myVideo, 0, 0, this.videoWidth, this.videoHeight)
const coverUrl = canvas.toDataURL()
this.coverUrl = coverUrl
}
}
}
</script>
```
相关推荐
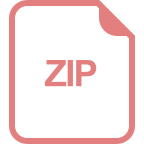
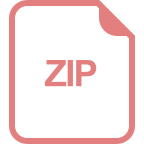














