sqlite 读取 blob
时间: 2023-10-19 13:20:20 浏览: 267
在 SQLite 中,可以通过以下步骤读取 blob 数据:
1. 打开 SQLite 数据库连接。
2. 准备 SQL 查询语句,其中包含读取 blob 数据的语句。
3. 执行查询语句,获取查询结果集。
4. 从结果集中读取 blob 数据。
下面是一个示例代码,演示了如何读取 SQLite 数据库中的 blob 数据:
```python
import sqlite3
# 打开 SQLite 数据库连接
conn = sqlite3.connect('example.db')
# 准备 SQL 查询语句,其中包含读取 blob 数据的语句
sql = "SELECT data FROM mytable WHERE id = ?"
# 执行查询语句,获取查询结果集
cursor = conn.cursor()
cursor.execute(sql, (1,))
result = cursor.fetchone()
# 从结果集中读取 blob 数据
blob_data = result[0]
print(blob_data)
# 关闭数据库连接
conn.close()
```
在上面的示例中,首先打开了 SQLite 数据库连接。然后,准备了一个包含读取 blob 数据的 SQL 查询语句,并执行该语句。最后,从查询结果集中获取 blob 数据,并打印输出。最后,关闭数据库连接。
需要注意的是,上面的代码仅供参考,具体实现方式可能因数据库版本等因素而异。在实际应用中,请根据具体情况进行调整。
相关问题
qt sqlite 读取 blob
在 Qt 中使用 SQLite 读取 Blob 数据类型的步骤如下:
1. 打开数据库连接并准备查询语句。
```
QSqlDatabase db = QSqlDatabase::addDatabase("QSQLITE");
db.setDatabaseName("mydatabase.db");
if (!db.open()) {
qDebug() << "Failed to connect to database!";
return;
}
QSqlQuery query(db);
query.prepare("SELECT myblobcolumn FROM mytable WHERE id = :id");
query.bindValue(":id", 1);
```
2. 执行查询语句并获取 Blob 数据。
```
if (query.exec() && query.first()) {
QByteArray blobData = query.value(0).toByteArray();
// 处理 blobData
} else {
qDebug() << "Failed to retrieve blob data!";
return;
}
```
在上述代码中,我们使用 `QSqlQuery` 类来准备查询语句,并且使用 `bindValue()` 方法来绑定参数。然后,我们执行查询并使用 `toByteArray()` 方法获取 Blob 数据。最后,我们可以对这些数据进行处理。
请注意,如果 Blob 数据较大,可能需要使用流式读取来避免内存问题。
qt sqlite 读取 blob 写入image
在 Qt 中读取 SQLite 中的 BLOB 数据并将其写入图像,可以按照以下步骤进行:
1. 打开 SQLite 数据库并准备读取 BLOB 数据。可以使用 `QSqlDatabase` 和 `QSqlQuery` 类来执行此操作。
```cpp
QSqlDatabase db = QSqlDatabase::addDatabase("QSQLITE");
db.setDatabaseName("path/to/database");
if (!db.open()) {
qDebug() << "database open error";
return;
}
QSqlQuery query(db);
query.prepare("SELECT image FROM table WHERE id = :id");
query.bindValue(":id", 1);
if (!query.exec()) {
qDebug() << "query execution error";
return;
}
if (!query.next()) {
qDebug() << "no data found";
return;
}
QByteArray blobData = query.value(0).toByteArray();
```
2. 将 BLOB 数据写入临时文件。可以使用 `QTemporaryFile` 类来创建临时文件并将数据写入其中。
```cpp
QTemporaryFile tempFile;
if (!tempFile.open()) {
qDebug() << "temporary file creation error";
return;
}
tempFile.write(blobData);
tempFile.close();
```
3. 从临时文件中读取图像数据并显示。可以使用 `QImage` 类来加载图像数据并将其显示在控件中。
```cpp
QImage image;
if (!image.load(tempFile.fileName())) {
qDebug() << "image loading error";
return;
}
ui->label->setPixmap(QPixmap::fromImage(image));
```
完整的代码示例:
```cpp
QSqlDatabase db = QSqlDatabase::addDatabase("QSQLITE");
db.setDatabaseName("path/to/database");
if (!db.open()) {
qDebug() << "database open error";
return;
}
QSqlQuery query(db);
query.prepare("SELECT image FROM table WHERE id = :id");
query.bindValue(":id", 1);
if (!query.exec()) {
qDebug() << "query execution error";
return;
}
if (!query.next()) {
qDebug() << "no data found";
return;
}
QByteArray blobData = query.value(0).toByteArray();
QTemporaryFile tempFile;
if (!tempFile.open()) {
qDebug() << "temporary file creation error";
return;
}
tempFile.write(blobData);
tempFile.close();
QImage image;
if (!image.load(tempFile.fileName())) {
qDebug() << "image loading error";
return;
}
ui->label->setPixmap(QPixmap::fromImage(image));
```
阅读全文
相关推荐
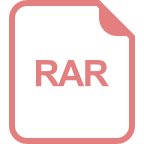
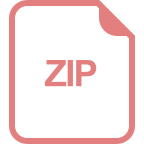













